Mastering Functional Programming in JavaScript Through Practical Examples
In the realm of JavaScript and, more broadly, functional programming, higher-order functions represent a significant and powerful concept. These functions play a critical role in creating efficient, readable, and maintainable code, making them crucial for anyone looking to hire JavaScript developers. This article aims to delve into the concept of higher-order functions, their usage, and the benefits they bring to JavaScript development, thereby enhancing the capabilities of JavaScript developers in the competitive market.
1. What Are Higher-Order Functions?
Higher-order functions are a key component of functional programming in JavaScript. They are functions that operate on other functions, either by taking them as arguments or by returning them.
In simple terms, if a function changes or builds other functions, it is a higher-order function. Mastery of these higher-order functions is often an important criterion when organizations look to hire JavaScript developers.
The primary idea behind higher-order functions is to use functions as values, enabling us to create more abstract operations, like ‘sort’, ‘filter’, or ‘map’, and to build more complex logic through simple building blocks. These skills are highly valuable and sought after in the industry, which makes understanding them essential for JavaScript developers seeking new opportunities.
2. An Introduction to JavaScript Higher-Order Functions
There are three main types of higher-order functions in JavaScript:
- Functions that accept other functions as arguments.
- Functions that return other functions.
- Functions that do both – they accept one or more functions as arguments and also return a function.
Let’s dive into each one of them.
2.1 Functions That Accept Other Functions as Arguments
These are the most common types of higher-order functions. They take one or more functions as parameters and perform operations on them. Popular examples are Array methods like ‘map’, ‘filter’, ‘reduce’, and ‘forEach’.
Example: ‘Array.prototype.map()’
The `map()` method creates a new array populated with the results of calling a provided function on every element in the calling array. Here’s an example:
```javascript const numbers = [1, 2, 3, 4, 5]; const squares = numbers.map(function(num) { return num * num; }); console.log(squares); // [1, 4, 9, 16, 25] ```
Here, the `map()` function is a higher-order function because it takes a function as an argument.
Example: ‘Array.prototype.filter()’
The `filter()` method creates a new array with all elements that pass the test implemented by the provided function. Here’s an example:
```javascript const numbers = [1, 2, 3, 4, 5]; const evens = numbers.filter(function(num) { return num % 2 === 0; }); console.log(evens); // [2, 4] ```
In this example, `filter()` is the higher-order function accepting another function as an argument.
2.2 Functions That Return Other Functions
These higher-order functions return another function when executed. They are often used in closures and function factories.
Example: Function Factory
A function factory is a function that returns another function, and the returned function can be customized. Here’s a simple example:
```javascript function multiplyBy(factor) { return function(num) { return num * factor; } } const double = multiplyBy(2); console.log(double(5)); // 10 const triple = multiplyBy(3); console.log(triple(5)); // 15 ```
Here, `multiplyBy()` is a higher-order function that returns a function. The returned function is customized by the argument passed to the factory function.
2.3 Functions That Accept and Return Functions
Some higher-order functions can take a function as an argument and also return a function. These are often used in function composition and decorators.
Example: Function Composition
Function composition is a technique to combine multiple functions into a single function. Here’s an example:
```javascript function compose(f, g) { return function(x) { return f(g(x)); } } function double(x) { return x * 2; } function increment(x) { return x + 1; } const doubleAfterIncrement = compose(double, increment); console.log(doubleAfterIncrement(5)); // 12 ```
Here, `compose()` is a higher-order function that takes two functions `f` and `g` and returns a new function.
3. Benefits of Higher-Order Functions
Higher-order functions offer multiple benefits:
- Code Reusability and DRY (Don’t Repeat Yourself): They reduce code repetition by abstracting the common logic into a function.
- Code Readability and Maintenance: They make the code more readable and easier to understand. Also, since the logic is centralized, the code is easier to maintain and update.
- Abstraction: Higher-order functions allow us to abstract actions, not just values. They let us create functions with less specificity, which we can later fine-tune with specific actions.
- Functional Programming Paradigms: They make functional programming possible in JavaScript. By allowing functions to be used as data, we can utilize powerful programming techniques like currying, function composition, and point-free programming.
Conclusion
Higher-order functions are a fundamental concept in JavaScript, driving better efficiency, readability, and maintenance of the code. They are an important skill for JavaScript developers and often serve as a benchmark in hiring processes. They allow us to write more declarative and less imperative code, which is always beneficial in a complex codebase. Learning and mastering higher-order functions can significantly level up your JavaScript programming skills and increase your chances to get hired as a JavaScript developer. So keep exploring, coding, and improving!
Table of Contents
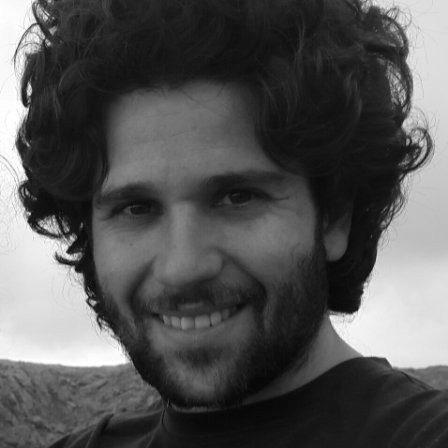
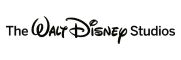