JavaScript Function Immutability: Creating Immutable Functions
Introduction to Function Immutability in JavaScript
Immutability is a key concept in functional programming, emphasizing that data should not be altered once created. In JavaScript, embracing immutability can lead to more predictable and bug-resistant code, especially in large-scale applications. This article explores the concept of function immutability in JavaScript and provides practical examples of how to create and work with immutable functions.
Why Immutability Matters
Immutability ensures that functions do not have side effects—meaning they do not alter the state of variables or objects outside their scope. This leads to code that is easier to debug, test, and maintain, as the same input will always produce the same output, without unexpected mutations.
Creating Immutable Functions in JavaScript
In JavaScript, immutability can be achieved by following certain best practices. Let’s explore some of these practices with code examples.
1. Avoiding Mutations in Functions
One of the key aspects of immutability is to avoid mutating input data. Instead of modifying the original object or array, return a new copy with the desired changes.
Example: Avoiding Mutation in Arrays
```javascript function addItemToArray(arr, item) { // Return a new array instead of mutating the original one return [...arr, item]; } const originalArray = [1, 2, 3]; const newArray = addItemToArray(originalArray, 4); console.log(originalArray); // [1, 2, 3] console.log(newArray); // [1, 2, 3, 4] ```
In the example above, the `addItemToArray` function returns a new array with the added item, leaving the original array unchanged.
2. Working with Immutable Data Structures
Libraries like [Immutable.js](https://immutable-js.github.io/immutable-js/) provide data structures that are inherently immutable. These structures ensure that any changes create a new copy of the data, rather than modifying the original.
Example: Using Immutable.js
```javascript const { Map } = require('immutable'); const originalMap = Map({ key: 'value' }); const newMap = originalMap.set('key', 'newValue'); console.log(originalMap.get('key')); // 'value' console.log(newMap.get('key')); // 'newValue' ```
Using `Immutable.js`, the `set` operation returns a new map with the updated value, ensuring the original map remains unchanged.
3. Freezing Objects with `Object.freeze`
JavaScript’s `Object.freeze` method can be used to prevent modifications to objects, making them immutable. However, it’s important to note that `Object.freeze` only works for shallow immutability, meaning nested objects can still be mutated.
Example: Freezing an Object
```javascript const originalObject = Object.freeze({ key: 'value' }); originalObject.key = 'newValue'; // This will silently fail in non-strict mode console.log(originalObject.key); // 'value' ```
Freezing an object ensures that its properties cannot be changed, protecting the data from unintended mutations.
4. Avoiding Side Effects in Functions
Immutability also involves ensuring that functions do not produce side effects. This means they should not alter any external state and should only depend on their input parameters.
Example: Pure Function without Side Effects
```javascript let count = 0; function incrementCounter(value) { return value + 1; } const newCount = incrementCounter(count); console.log(count); // 0 console.log(newCount); // 1 ```
The `incrementCounter` function is pure and immutable because it only relies on its input and does not alter the external `count` variable.
Immutability in Modern JavaScript Development
Modern JavaScript frameworks like React promote the use of immutability to manage state efficiently. By adhering to immutable patterns, developers can take advantage of features like efficient re-rendering and time-travel debugging.
Conclusion
Embracing immutability in JavaScript functions can lead to more predictable, maintainable, and error-resistant code. Whether you’re avoiding mutations, using immutable data structures, or ensuring that functions are pure, immutability is a powerful concept that can significantly improve your JavaScript development practices.
Further Reading
Table of Contents
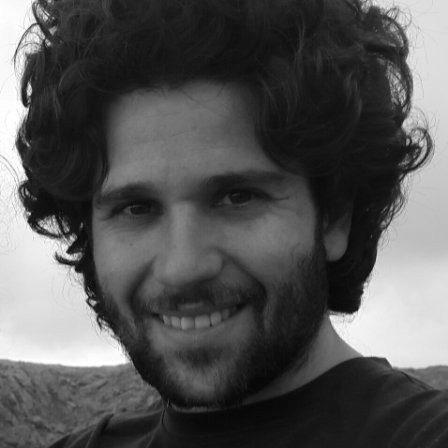
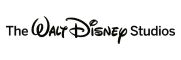