JavaScript Memoization: Boosting Performance with Cached Functions
As JavaScript applications grow in complexity and handle larger data sets, optimizing performance becomes crucial. One powerful technique to achieve better performance is “memoization.” Memoization is a form of caching that stores the results of expensive function calls and reuses them when the same inputs occur again. By leveraging memoization, you can significantly reduce redundant computations and improve the overall efficiency of your code.
Table of Contents
In this blog, we will dive into the world of memoization, understand its inner workings, explore its benefits, and learn how to apply it effectively in JavaScript applications. We’ll also cover different memoization strategies, including simple memoization, memoization with closure, and memoization with libraries like Lodash.
1. Understanding Memoization
What is Memoization?
Memoization is an optimization technique used to cache the results of expensive function calls and reuse them when the same inputs occur again. It is particularly useful for functions that take a significant amount of time to execute or involve repeated computations with identical inputs.
The basic idea behind memoization is to store the function’s return value in a cache based on its arguments. When the function is called again with the same arguments, instead of recomputing the result, the cached value is returned, saving time and resources.
How Does Memoization Work?
Let’s understand the memoization process with a simple example of a Fibonacci function:
javascript // Non-memoized Fibonacci function function fibonacci(n) { if (n <= 2) return 1; return fibonacci(n - 1) + fibonacci(n - 2); }
In the non-memoized version of the Fibonacci function, calling fibonacci(5) would result in multiple redundant calculations of the same Fibonacci numbers, leading to performance bottlenecks as n increases.
To implement memoization, we can introduce a cache object to store the results:
javascript function memoizedFibonacci(n, cache = {}) { if (n in cache) return cache[n]; if (n <= 2) return 1; cache[n] = memoizedFibonacci(n - 1, cache) + memoizedFibonacci(n - 2, cache); return cache[n]; }
By storing the results in the cache, the memoized version of the Fibonacci function will only compute each Fibonacci number once and reuse the cached values for subsequent calls with the same arguments, resulting in a significant performance boost.
2. The Benefits of Memoization
Performance Improvement
One of the primary advantages of memoization is its ability to improve performance in recursive or repetitive calculations. By storing previously computed results, the function avoids redundant work, leading to faster execution times.
Reduced Recalculations
In complex algorithms or recursive functions, the same subproblems are often recalculated multiple times. Memoization helps eliminate these redundant recalculations by saving the results in a cache, which can lead to substantial time savings.
Code Simplicity
Memoization allows developers to focus on writing simple and straightforward functions without worrying about optimization. By separating the concerns of computation and caching, the code becomes cleaner and more maintainable.
3. Implementing Memoization
Simple Memoization
Simple memoization can be achieved using a cache object within the function. Let’s look at a basic example of memoization for a factorial function:
javascript function factorial(n, cache = {}) { if (n in cache) return cache[n]; if (n === 0) return 1; cache[n] = n * factorial(n - 1, cache); return cache[n]; }
In this example, the factorial function stores computed factorials in the cache and reuses them when called with the same argument.
Memoization with Closure
Another approach to implement memoization involves using closures. We can create a higher-order function that accepts the original function as an argument and returns a memoized version of it. Let’s see how this can be done:
javascript function memoize(func) { const cache = {}; return function (...args) { const key = JSON.stringify(args); if (key in cache) return cache[key]; const result = func(...args); cache[key] = result; return result; }; } // Usage const memoizedFactorial = memoize(function factorial(n) { if (n === 0) return 1; return n * factorial(n - 1); });
In this approach, the memoize function wraps the original factorial function in a closure and returns a new function that handles caching using the cache object.
4. Memoization Libraries
Introducing Lodash
Lodash is a popular utility library in JavaScript that provides various functions to simplify common programming tasks. One of its features is the built-in memoization function, which can be extremely handy for optimizing performance.
Using Lodash’s Memoize Function
To use Lodash’s memoize function, you first need to install Lodash in your project:
bash npm install lodash
Now, let’s see how to apply memoization to a simple function using Lodash:
javascript const _ = require('lodash'); function expensiveOperation(arg) { // Assume this function is computationally expensive // and takes some time to execute return arg + 1; } const memoizedExpensiveOperation = _.memoize(expensiveOperation);
Lodash’s memoize function automatically caches the results of expensiveOperation, making subsequent calls with the same argument much faster.
5. Memoization Use Cases
Fibonacci Sequence
The Fibonacci sequence is a classic example of a problem that can benefit from memoization. Let’s compare the performance of the memoized and non-memoized Fibonacci functions:
javascript function fibonacci(n) { if (n <= 2) return 1; return fibonacci(n - 1) + fibonacci(n - 2); } const memoizedFibonacci = memoize(fibonacci);
When calculating larger Fibonacci numbers, the memoized version significantly outperforms the non-memoized version due to the avoidance of redundant calculations.
Factorial Function
The factorial function is another use case for memoization:
javascript function factorial(n) { if (n === 0) return 1; return n * factorial(n - 1); } const memoizedFactorial = memoize(factorial);
The memoizedFactorial function will prevent redundant calculations of factorial values, leading to faster and more efficient code.
6. Tips and Best Practices
Handling Impure Functions
Memoization is most effective for pure functions that always return the same output for a given input. Be cautious when using memoization with impure functions that rely on external state, as it might lead to incorrect or unexpected results.
Cache Expiration and Limitations
In some cases, memoization can lead to excessive memory usage if the cache keeps growing indefinitely. Implement cache expiration or size limits to prevent memory-related issues in long-running applications.
Profiling for Performance
Profile your application’s performance to identify functions that could benefit from memoization. Focus on bottlenecks and frequently called functions to achieve the most significant performance improvements.
Conclusion
Memoization is a powerful technique to boost JavaScript application performance by caching function results and reusing them when needed. By eliminating redundant computations, memoization significantly reduces execution time and enhances overall efficiency. Whether you choose to implement simple memoization, memoization with closure, or utilize libraries like Lodash, understanding and applying this optimization technique can lead to substantial performance gains in your code. Always remember to profile and test your application to ensure that memoization is enhancing performance as expected. Happy optimizing!
Table of Contents
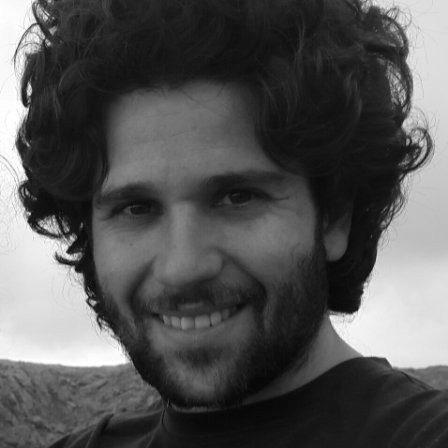
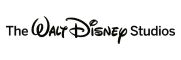