JavaScript Function Memoization Libraries: Performance Optimization Made Easy
Memoization is a powerful technique for optimizing the performance of JavaScript functions. By caching the results of expensive function calls, memoization reduces redundant calculations, leading to significant performance improvements, especially in applications with repetitive computations. This article explores how memoization works in JavaScript and introduces popular memoization libraries that simplify its implementation.
Why Use Memoization?
Memoization is especially useful in scenarios where functions are called repeatedly with the same arguments. By storing the result of the first function call and returning it for subsequent calls with the same arguments, memoization can drastically reduce the execution time of your JavaScript code. This is particularly beneficial in recursive functions, complex computations, and functions with high-frequency calls.
Implementing Basic Memoization in JavaScript
Before diving into libraries, let’s understand how memoization can be implemented manually in JavaScript.
Example: Basic Memoization Implementation
Here’s a simple example of memoization in JavaScript, where a function `slowFunction` is memoized to improve performance.
```javascript function memoize(fn) { const cache = new Map(); return function (...args) { const key = JSON.stringify(args); if (cache.has(key)) { return cache.get(key); } const result = fn(...args); cache.set(key, result); return result; }; } function slowFunction(num) { // Simulate an expensive computation console.log('Computing...'); return num * 2; } const memoizedFunction = memoize(slowFunction); console.log(memoizedFunction(5)); // Computing... 10 console.log(memoizedFunction(5)); // 10 (cached result) ```
Exploring Memoization Libraries
While implementing memoization from scratch is straightforward, using a library can save time and provide additional features. Below are some popular JavaScript libraries for memoization.
1. Lodash Memoize
Lodash, a widely-used utility library, includes a `memoize` function that is both easy to use and highly efficient.
Example: Using Lodash’s Memoize
```javascript const _ = require('lodash'); function expensiveCalculation(n) { console.log('Calculating...'); return n * n; } const memoizedCalculation = _.memoize(expensiveCalculation); console.log(memoizedCalculation(4)); // Calculating... 16 console.log(memoizedCalculation(4)); // 16 (cached result) ```
Advantages:
– Simple to use and integrate into existing codebases.
– Supports cache customization.
2. Fast Memoize
Fast Memoize is a lightweight library focused on speed and efficiency. It’s ideal for performance-critical applications.
Example: Using Fast Memoize
```javascript const memoize = require('fast-memoize'); function heavyComputation(x) { console.log('Running computation...'); return x + 10; } const memoizedComputation = memoize(heavyComputation); console.log(memoizedComputation(7)); // Running computation... 17 console.log(memoizedComputation(7)); // 17 (cached result) ```
Advantages:
– Optimized for speed.
– Minimalistic and fast to set up.
3. Memoizee
Memoizee is a highly configurable memoization library that offers various options for cache management, including time-based expiration and cache limits.
Example: Using Memoizee
```javascript const memoizee = require('memoizee'); function computeFactorial(n) { console.log('Calculating factorial...'); return n <= 1 ? 1 : n * computeFactorial(n - 1); } const memoizedFactorial = memoizee(computeFactorial); console.log(memoizedFactorial(5)); // Calculating factorial... 120 console.log(memoizedFactorial(5)); // 120 (cached result) ```
Advantages:
– Highly customizable.
– Supports advanced features like cache expiration and primitive argument caching.
When to Use Memoization?
Memoization is a powerful optimization technique but should be used judiciously. It’s best suited for pure functions (functions without side effects) that are called frequently with the same inputs. Avoid memoizing functions with unpredictable outcomes or side effects, as this can lead to unexpected behavior.
Conclusion
Memoization is a straightforward yet powerful technique to optimize JavaScript performance. By leveraging memoization libraries like Lodash, Fast Memoize, and Memoizee, you can enhance your application’s efficiency with minimal effort. Understanding when and how to use memoization will help you write faster, more efficient JavaScript code.
Further Reading:
Table of Contents
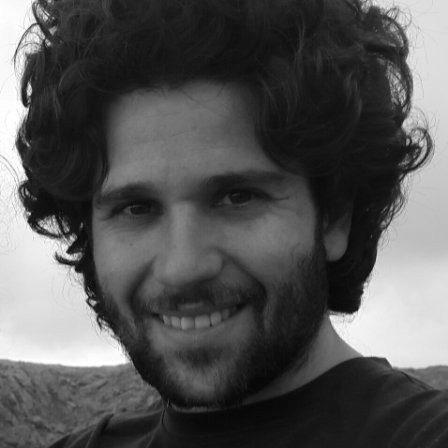
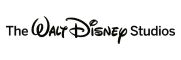