JavaScript Function Memoization Strategies: Performance Trade-offs
Memoization is a powerful optimization technique in JavaScript that can significantly improve the performance of functions by caching their results. This article explores different memoization strategies, examines their performance trade-offs, and provides practical examples to illustrate their use.
Understanding Memoization
Memoization involves storing the results of expensive function calls and reusing these results when the same inputs occur again. This approach can drastically reduce the time complexity of functions that are called repeatedly with the same arguments, especially in scenarios where functions have high computational costs.
Memoization Strategies in JavaScript
JavaScript provides several ways to implement memoization. Below are some common strategies and their performance implications:
1. Simple Object-based Memoization
A straightforward approach to memoization involves using an object to store previously computed results. This method works well for functions with a limited set of inputs.
Example: Memoizing with an Object
```javascript function memoize(fn) { const cache = {}; return function(...args) { const key = JSON.stringify(args); if (key in cache) { return cache[key]; } const result = fn(...args); cache[key] = result; return result; }; } function factorial(n) { if (n === 0 || n === 1) return 1; return n * factorial(n - 1); } const memoizedFactorial = memoize(factorial); console.log(memoizedFactorial(5)); // Computes and caches result console.log(memoizedFactorial(5)); // Returns cached result ```
Trade-offs:
– Pros: Simple to implement; works well for functions with primitive arguments.
– Cons: Can lead to high memory usage if the number of unique inputs is large.
2. Using `WeakMap` for Memory Efficiency
For functions with objects or arrays as arguments, `WeakMap` can be used to store cached results. `WeakMap` allows garbage collection of unused keys, making it more memory-efficient.
Example: Memoizing with `WeakMap`
```javascript function memoize(fn) { const cache = new WeakMap(); return function(...args) { const key = args[0]; // Assuming single object argument if (cache.has(key)) { return cache.get(key); } const result = fn(...args); cache.set(key, result); return result; }; } function computeComplexData(obj) { // Some complex computation return obj.value * 2; } const memoizedCompute = memoize(computeComplexData); const obj = { value: 10 }; console.log(memoizedCompute(obj)); // Computes and caches result console.log(memoizedCompute(obj)); // Returns cached result ```
Trade-offs:
– Pros: More memory-efficient; avoids issues with large cache sizes.
– Cons: Limited to objects and arrays; cannot handle primitive values.
3. Using LRU (Least Recently Used) Cache
An LRU cache strategy ensures that only a fixed number of recent function results are kept in memory, evicting the least recently used entries when the limit is reached.
Example: Implementing an LRU Cache
```javascript class LRUCache { constructor(limit) { this.limit = limit; this.cache = new Map(); } get(key) { if (!this.cache.has(key)) return undefined; const value = this.cache.get(key); this.cache.delete(key); this.cache.set(key, value); return value; } set(key, value) { if (this.cache.size >= this.limit) { const oldestKey = this.cache.keys().next().value; this.cache.delete(oldestKey); } this.cache.set(key, value); } } function memoize(fn, limit = 5) { const cache = new LRUCache(limit); return function(...args) { const key = JSON.stringify(args); const cachedResult = cache.get(key); if (cachedResult !== undefined) { return cachedResult; } const result = fn(...args); cache.set(key, result); return result; }; } function expensiveComputation(n) { // Simulate an expensive computation return n * n; } const memoizedComputation = memoize(expensiveComputation); console.log(memoizedComputation(10)); // Computes and caches result console.log(memoizedComputation(10)); // Returns cached result ```
Trade-offs:
– Pros: Efficient memory use; evicts old results based on usage.
– Cons: More complex implementation; requires managing cache size.
4. Using Libraries for Memoization
There are libraries available that offer robust memoization solutions. These libraries often include features such as LRU caching and function throttling.
Example: Using Lodash for Memoization
```javascript const _ = require('lodash'); function expensiveOperation(n) { // Simulate an expensive operation return n * n; } const memoizedOperation = _.memoize(expensiveOperation); console.log(memoizedOperation(5)); // Computes and caches result console.log(memoizedOperation(5)); // Returns cached result ```
Trade-offs:
– Pros: Quick to implement; tested and optimized solutions.
– Cons: External dependency; may include more features than needed.
Conclusion
Memoization is a valuable technique for optimizing performance in JavaScript applications. By choosing the appropriate strategy based on the function’s characteristics and memory constraints, you can significantly enhance your application’s efficiency. Whether you use simple object caching, `WeakMap`, LRU caching, or a library like Lodash, understanding the trade-offs will help you make informed decisions for better performance.
Further Reading:
Table of Contents
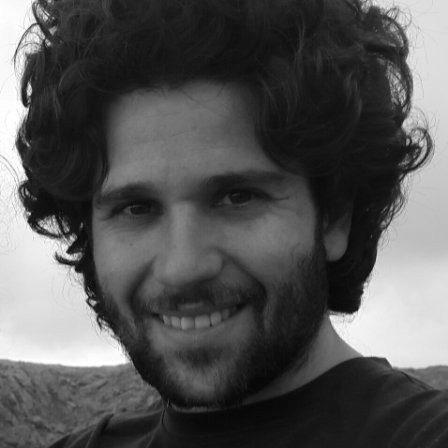
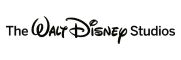