JavaScript Function Memoization Techniques for Performance Boost
In JavaScript, performance optimization is a critical concern, especially when dealing with functions that are called repeatedly with the same inputs. Memoization is a powerful technique that can significantly enhance performance by caching the results of expensive function calls and returning the cached result when the same inputs occur again. This article explores various memoization techniques in JavaScript, providing practical examples and strategies to boost the performance of your applications.
Understanding Memoization
Memoization is a form of caching that stores the results of function calls in a cache, typically a key-value store. When the function is called again with the same arguments, the cached result is returned instead of recomputing the result. This can lead to substantial performance gains, especially for functions with heavy computational workloads or those that are called frequently.
1. Basic Memoization Technique
The most straightforward way to implement memoization in JavaScript is by using an object or a `Map` to store cached results. Below is a simple example demonstrating how to memoize a recursive function.
Example: Memoizing a Recursive Fibonacci Function
```javascript function memoize(fn) { const cache = {}; return function (...args) { const key = JSON.stringify(args); if (cache[key]) { return cache[key]; } const result = fn(...args); cache[key] = result; return result; }; } function fibonacci(n) { if (n <= 1) return n; return fibonacci(n - 1) + fibonacci(n - 2); } const memoizedFibonacci = memoize(fibonacci); console.log(memoizedFibonacci(40)); // Calculated and cached console.log(memoizedFibonacci(40)); // Retrieved from cache ```
In this example, the `memoize` function creates a cache for storing the results of the `fibonacci` function. When the `fibonacci` function is called again with the same input, the result is returned from the cache, avoiding unnecessary recomputation.
2. Advanced Memoization with Custom Cache Strategies
In some scenarios, you may need more sophisticated caching strategies, such as limiting the cache size or handling complex input types. Here’s an example of a custom memoization strategy using a `Map` with a cache size limit.
Example: Memoizing with Limited Cache Size
```javascript function memoize(fn, cacheSize = 5) { const cache = new Map(); return function (...args) { const key = JSON.stringify(args); if (cache.has(key)) { return cache.get(key); } const result = fn(...args); cache.set(key, result); if (cache.size > cacheSize) { const firstKey = cache.keys().next().value; cache.delete(firstKey); } return result; }; } function expensiveCalculation(a, b) { // Simulate a time-consuming computation return a b Math.random(); } const memoizedCalculation = memoize(expensiveCalculation, 3); console.log(memoizedCalculation(2, 3)); // Calculated and cached console.log(memoizedCalculation(2, 3)); // Retrieved from cache ```
This version of `memoize` limits the cache size to a specified number of entries. When the cache exceeds the limit, the oldest entry is removed. This technique is useful in memory-constrained environments or when the function is called with a large variety of inputs.
3. Memoizing Asynchronous Functions
Memoization can also be applied to asynchronous functions that return promises. This is particularly useful for functions that make network requests or perform other I/O operations.
Example: Memoizing an Asynchronous API Call
```javascript function memoizeAsync(fn) { const cache = new Map(); return async function (...args) { const key = JSON.stringify(args); if (cache.has(key)) { return cache.get(key); } const promise = fn(...args); cache.set(key, promise); try { const result = await promise; return result; } catch (error) { cache.delete(key); throw error; } }; } async function fetchData(url) { const response = await fetch(url); return response.json(); } const memoizedFetchData = memoizeAsync(fetchData); memoizedFetchData('https://api.example.com/data').then(console.log); memoizedFetchData('https://api.example.com/data').then(console.log); // Cached result ```
In this example, the `memoizeAsync` function handles the caching of promises returned by asynchronous functions. The cache stores the promise itself, and if the promise is resolved or rejected, the appropriate action is taken.
4. Clearing the Cache
Sometimes, you may need to clear the cache, either periodically or based on specific conditions. This can be achieved by exposing a method to clear the cache or by integrating cache-clearing logic into the memoization function.
Example: Adding Cache Clearing Capability
```javascript function memoizeWithClear(fn) { const cache = new Map(); function memoized(...args) { const key = JSON.stringify(args); if (cache.has(key)) { return cache.get(key); } const result = fn(...args); cache.set(key, result); return result; } memoized.clearCache = () => cache.clear(); return memoized; } const memoizedWithClear = memoizeWithClear(expensiveCalculation); memoizedWithClear(2, 3); memoizedWithClear.clearCache(); ```
This approach provides a `clearCache` method that can be called to clear the cache when needed.
Conclusion
Memoization is a powerful technique for optimizing the performance of JavaScript functions, particularly those that are computationally expensive or frequently called. By caching results and returning them for repeated inputs, you can significantly reduce the time and resources needed to execute your code. Whether you are dealing with recursive algorithms, API calls, or any other functions, understanding and implementing memoization can lead to more efficient and responsive applications.
Further Reading
Table of Contents
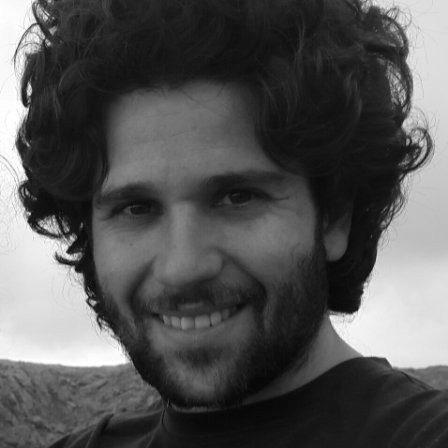
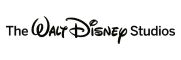