Exploring JavaScript Named vs. Anonymous Functions: Pros and Cons
JavaScript, the backbone of modern web development, offers a plethora of features to create dynamic and interactive web applications. One of its fundamental building blocks is the function, which enables developers to encapsulate blocks of code for reuse and maintainability. When it comes to functions, two main categories stand out: named and anonymous functions. In this article, we’ll delve into the pros and cons of both approaches, along with examples and best use cases.
Table of Contents
1. Named Functions: Defining Identity
Named functions, as the name suggests, are functions with a specified identifier. They are declared using the function keyword followed by the function’s name, a set of parameters within parentheses, and the function body enclosed in curly braces. Let’s explore their advantages and disadvantages.
1.1. Pros of Named Functions:
1.1.1. Self-Explanatory Code:
Named functions provide a clear and descriptive name to the encapsulated logic, making the code more readable and understandable. This aids in the maintenance of the codebase, as developers can quickly grasp the purpose of the function.
1.1.2. Easier Recursion:
Recursion, a programming technique where a function calls itself, is more straightforward with named functions. The function’s name serves as an identifier for the recursive call, enhancing the clarity of the code.
1.2. Cons of Named Functions:
1.2.1. Global Namespace Pollution:
Named functions are added to the global namespace, which can lead to naming conflicts when multiple functions have the same name. This issue is known as namespace pollution and can result in unexpected behavior.
1.2.2. Hoisting Impact:
In JavaScript, function declarations are hoisted to the top of their scope. While this can be advantageous, it can also lead to unexpected behavior if not managed properly, as functions might be called before they are defined.
1.3. Example of Named Function:
javascript // Named Function function calculateSquare(number) { return number * number; } const result = calculateSquare(5); console.log(result); // Output: 25
2. Anonymous Functions: Embracing Flexibility
Anonymous functions, also known as lambda functions or function expressions, lack a specific name. They are defined without an identifier and can be assigned to variables or passed as arguments to other functions.
2.1. Pros of Anonymous Functions:
2.1.1. Local Scope:
Since anonymous functions are often assigned to variables, they have a more limited scope. This helps in preventing global namespace pollution and potential naming conflicts.
2.1.2. Closures and Encapsulation:
Anonymous functions are often used to create closures, allowing them to capture and maintain the surrounding state. This can be advantageous for scenarios like event handling, where the function retains access to variables even after its parent function has completed execution.
2.2. Cons of Anonymous Functions:
2.2.1. Readability Challenges:
The absence of a descriptive name for anonymous functions can hinder code readability, especially in complex codebases. Understanding the purpose of the function requires analyzing its context.
2.2.2. Limited Recursion:
Anonymous functions can be challenging to use in recursive scenarios due to the lack of a named identifier. Recursion might require extra workarounds to ensure correct function calls.
2.3. Example of Anonymous Function:
javascript // Anonymous Function assigned to a variable const multiply = function(a, b) { return a * b; }; const product = multiply(3, 4); console.log(product); // Output: 12
3. Best Use Cases:
3.1. Named Functions:
Use named functions when clarity and self-explanatory code are essential, especially for functions that will be used frequently or across multiple parts of the codebase.
Opt for named functions when recursion plays a significant role in your application logic.
3.2. Anonymous Functions:
Choose anonymous functions when encapsulation and closure behavior are necessary, such as in event listeners and asynchronous callbacks.
Utilize anonymous functions for short-lived functions that are only used in a specific context.
Conclusion
JavaScript functions are integral to writing clean, organized, and maintainable code. Whether you opt for named functions with their descriptive identities or anonymous functions with their encapsulation abilities, the choice depends on the specific use case. Named functions shine in scenarios where readability and recursion are crucial, while anonymous functions excel in cases requiring local scope and closures. By understanding the pros and cons of each approach, developers can make informed decisions to create effective and efficient JavaScript code.
Table of Contents
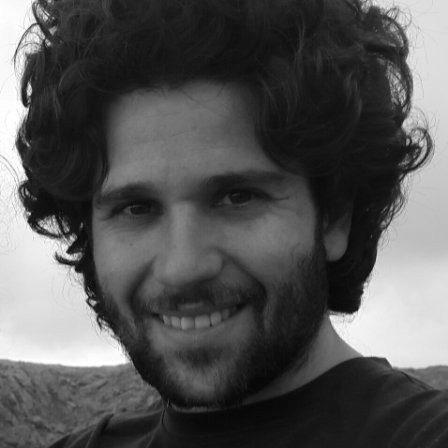
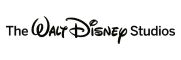