JavaScript Function Overriding: Extending Functionality Safely
Function overriding in JavaScript is a powerful technique that allows developers to modify or extend existing functionality. However, without careful consideration, it can lead to unexpected behavior and bugs. This article explores how to safely override functions in JavaScript and provides practical examples to help you implement these changes effectively.
Understanding Function Overriding in JavaScript
Function overriding occurs when a function in a subclass or derived object has the same name as a function in its superclass or prototype chain. The new function replaces the old one when called on the subclass or object instance, allowing for extended or modified behavior.
Safely Overriding Functions
Overriding functions should be done with caution to avoid unintended side effects. Key considerations include preserving the original function’s behavior, handling edge cases, and ensuring compatibility with other parts of your code.
1. Basic Function Overriding
In JavaScript, you can easily override a function by redefining it in a subclass or derived object. However, to maintain the original functionality, it’s often necessary to call the parent function using the `super` keyword (in classes) or by directly invoking the function from the prototype chain.
Example: Overriding a Method in a Class
```javascript class Animal { speak() { console.log("Animal speaks"); } } class Dog extends Animal { speak() { console.log("Dog barks"); super.speak(); // Calling the parent class method } } const myDog = new Dog(); myDog.speak(); // Output: // Dog barks // Animal speaks ```
In this example, the `Dog` class overrides the `speak` method but also calls the original `speak` method from the `Animal` class using `super.speak()`.
2. Overriding Methods in Prototypes
When dealing with prototype-based inheritance, you might want to override methods in an object’s prototype. This can be done by directly assigning a new function to the prototype’s method.
Example: Overriding a Prototype Method
```javascript function Car() {} Car.prototype.start = function() { console.log("Car is starting"); }; const myCar = new Car(); myCar.start(); // Car is starting // Override the start method Car.prototype.start = function() { console.log("Car is now starting with new functionality"); }; myCar.start(); // Car is now starting with new functionality ```
Here, the `start` method in the `Car` prototype is overridden to provide new functionality.
3. Preserving Functionality with Wrapping
To ensure that the original functionality is preserved while adding new behavior, you can wrap the original function in a new one. This is especially useful when you need to add pre-processing or post-processing steps.
Example: Wrapping a Function
```javascript function calculate(a, b) { return a + b; } const originalCalculate = calculate; calculate = function(a, b) { console.log("Calculating..."); const result = originalCalculate(a, b); console.log("Result:", result); return result; }; calculate(5, 3); // Output: // Calculating... // Result: 8 ```
In this example, the original `calculate` function is wrapped in a new function that adds logging before and after the calculation.
4. Avoiding Conflicts with Other Code
When overriding functions, especially in shared environments like libraries or frameworks, it’s crucial to avoid conflicts with other code that may rely on the original functionality. Consider using unique method names or namespaces to minimize the risk of collisions.
Example: Using Namespaces to Avoid Conflicts
```javascript const MyLibrary = { calculate: function(a, b) { return a * b; } }; const originalCalculate = MyLibrary.calculate; MyLibrary.calculate = function(a, b) { console.log("Multiplying..."); return originalCalculate(a, b); }; MyLibrary.calculate(4, 5); // Output: // Multiplying... // 20 ```
Here, the `calculate` function is safely overridden within the `MyLibrary` namespace, reducing the risk of conflicts.
Conclusion
Function overriding in JavaScript is a flexible technique that, when used correctly, can extend and enhance existing functionality. By following best practices such as preserving original behavior, using wrapping techniques, and avoiding conflicts, you can safely implement function overriding in your projects. With careful consideration, this powerful tool can be used to create more dynamic and adaptable code.
Further Reading
Table of Contents
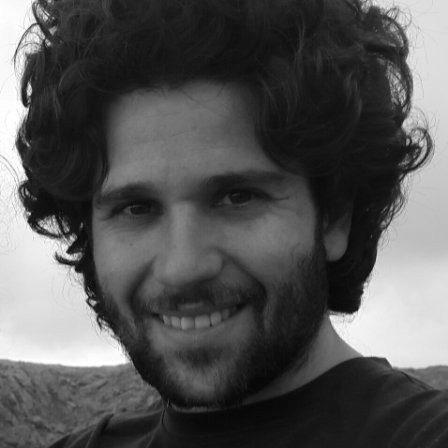
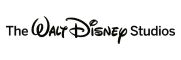