JavaScript Function Parameter Destructuring: Unpacking Function Inputs
JavaScript function parameter destructuring is a powerful feature that allows you to extract values from objects and arrays directly within the function signature. This technique helps to create cleaner, more readable code by reducing the need for repetitive variable assignments and making your function’s purpose more apparent.
Understanding the Basics of Destructuring
Destructuring in JavaScript is the process of unpacking values from arrays or properties from objects into distinct variables. When applied to function parameters, destructuring allows you to directly capture these values or properties in the function’s parameter list, simplifying the code.
Example 1: Destructuring Objects in Function Parameters
Suppose you have a function that accepts an object representing user information. With traditional parameter handling, you might extract properties manually inside the function. Using destructuring, you can simplify this process:
```javascript // Traditional approach function displayUserInfo(user) { const name = user.name; const age = user.age; console.log(`Name: ${name}, Age: ${age}`); } // With parameter destructuring function displayUserInfo({ name, age }) { console.log(`Name: ${name}, Age: ${age}`); } const user = { name: 'Alice', age: 30 }; displayUserInfo(user); // Output: Name: Alice, Age: 30 ```
In this example, the `displayUserInfo` function directly extracts the `name` and `age` properties from the `user` object within the function’s parameter list, making the code more concise.
Example 2: Destructuring Nested Objects
Destructuring also supports nested objects, allowing you to extract deeply nested properties with ease.
```javascript function displayUserDetails({ name, address: { city, country } }) { console.log(`Name: ${name}, City: ${city}, Country: ${country}`); } const user = { name: 'Bob', address: { city: 'New York', country: 'USA' } }; displayUserDetails(user); // Output: Name: Bob, City: New York, Country: USA ```
Here, the `address` object is nested within the `user` object, but the destructuring in the function parameter list allows direct access to `city` and `country`.
Example 3: Destructuring Arrays in Function Parameters
Just as with objects, you can destructure arrays in function parameters to unpack elements directly.
```javascript function displayCoordinates([x, y]) { console.log(`X: ${x}, Y: ${y}`); } const point = [10, 20]; displayCoordinates(point); // Output: X: 10, Y: 20 ```
This example shows how array elements can be directly unpacked into individual variables within the function signature.
Example 4: Providing Default Values
Destructuring also supports default values, which can be very useful when certain parameters might be undefined.
```javascript function greet({ name = 'Guest', message = 'Welcome!' }) { console.log(`${message}, ${name}`); } greet({ name: 'Charlie' }); // Output: Welcome!, Charlie greet({}); // Output: Welcome!, Guest ```
In this example, if `name` or `message` are not provided, the function uses the default values specified.
Example 5: Using Rest Syntax with Destructuring
You can combine destructuring with the rest syntax (`…`) to capture remaining elements or properties in an array or object.
```javascript function logUserDetails({ name, age, ...otherDetails }) { console.log(`Name: ${name}, Age: ${age}`); console.log('Other Details:', otherDetails); } const user = { name: 'Dave', age: 25, profession: 'Developer', country: 'Canada' }; logUserDetails(user); /* Output: Name: Dave, Age: 25 Other Details: { profession: 'Developer', country: 'Canada' } */ ```
In this example, the `…otherDetails` syntax captures all other properties of the `user` object that are not explicitly destructured.
Conclusion
JavaScript function parameter destructuring is a versatile and efficient feature that enhances the readability and maintainability of your code. Whether you’re dealing with objects, arrays, or a combination of both, destructuring allows you to write cleaner, more intuitive functions by directly unpacking inputs within the function signature.
Further Reading:
Table of Contents
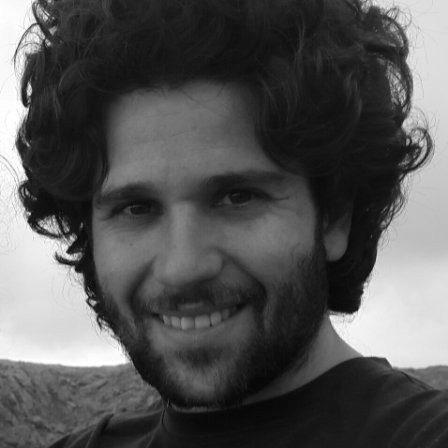
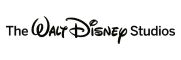