JavaScript Function Parameter Validation: Ensuring Correct Inputs
JavaScript functions are powerful tools that allow developers to encapsulate logic and reuse code efficiently. However, when functions receive incorrect or unexpected inputs, it can lead to bugs or even security vulnerabilities. Parameter validation is essential to ensure that functions operate correctly and reliably. This article explores various techniques to validate function parameters in JavaScript, with practical examples to guide you through the process.
Understanding the Importance of Parameter Validation
Parameter validation is the process of checking whether the inputs to a function meet certain criteria before executing the function’s logic. This step is crucial in preventing errors, handling edge cases, and ensuring that your code behaves as expected. Without proper validation, functions may produce incorrect results or fail unexpectedly, leading to difficult-to-debug issues.
Basic Validation Techniques
1. Type Checking
One of the simplest forms of validation is checking the type of the input parameters. JavaScript’s `typeof` operator can be used to ensure that the arguments passed to a function are of the correct type.
Example: Checking Parameter Types
```javascript function addNumbers(a, b) { if (typeof a !== 'number' || typeof b !== 'number') { throw new TypeError('Both arguments must be numbers'); } return a + b; } console.log(addNumbers(5, 10)); // 15 console.log(addNumbers(5, '10')); // Throws TypeError ```
In this example, the `addNumbers` function checks whether both `a` and `b` are numbers before performing the addition.
2. Handling Optional Parameters
Functions often have optional parameters that may or may not be provided by the caller. Using default parameters or checking for `undefined` values allows you to handle these cases gracefully.
Example: Handling Optional Parameters
```javascript function greet(name = 'Guest') { console.log(`Hello, ${name}!`); } greet('Alice'); // Hello, Alice! greet(); // Hello, Guest! ```
Here, the `greet` function provides a default value of `’Guest’` for the `name` parameter if it is not provided.
3. Using Custom Validation Functions
For more complex validation scenarios, custom validation functions can be employed. These functions can encapsulate specific validation logic that can be reused across multiple functions.
Example: Custom Validation Function
```javascript function isValidEmail(email) { const emailPattern = /^[^\s@]+@[^\s@]+\.[^\s@]+$/; return emailPattern.test(email); } function sendEmail(to, subject, body) { if (!isValidEmail(to)) { throw new Error('Invalid email address'); } // Proceed with sending the email } sendEmail('example@example.com', 'Hello', 'This is a test email'); // Success sendEmail('invalid-email', 'Hello', 'This is a test email'); // Throws Error ```
The `isValidEmail` function validates the email format before allowing the `sendEmail` function to proceed.
4. Leveraging Libraries for Validation
JavaScript offers various libraries that can simplify and enhance parameter validation. Libraries like `Joi` or `Validator.js` provide a rich set of tools for validating complex objects and data structures.
Example: Using Joi for Validation
```javascript const Joi = require('joi'); function validateUser(user) { const schema = Joi.object({ name: Joi.string().min(3).required(), email: Joi.string().email().required(), age: Joi.number().integer().min(18).required(), }); const { error } = schema.validate(user); if (error) { throw new Error(`Invalid user data: ${error.message}`); } } validateUser({ name: 'John', email: 'john@example.com', age: 25 }); // Success validateUser({ name: 'Jo', email: 'john@example.com', age: 25 }); // Throws Error ```
In this example, `Joi` is used to validate a `user` object against a defined schema, ensuring that all required fields are correctly formatted.
Ensuring Consistent Validation Across Your Codebase
5. Centralizing Validation Logic
To maintain consistency in how parameters are validated across your codebase, consider centralizing your validation logic. This can be done by creating a utility module that contains common validation functions.
Example: Centralized Validation Module
```javascript // validation.js export function isNonEmptyString(value) { return typeof value === 'string' && value.trim().length > 0; } export function isPositiveNumber(value) { return typeof value === 'number' && value > 0; } // main.js import { isNonEmptyString, isPositiveNumber } from './validation'; function createProduct(name, price) { if (!isNonEmptyString(name)) { throw new Error('Product name must be a non-empty string'); } if (!isPositiveNumber(price)) { throw new Error('Price must be a positive number'); } // Create the product } createProduct('Laptop', 999); // Success createProduct('', 999); // Throws Error ```
By centralizing the validation logic, you ensure that the same standards are applied consistently throughout your code.
Conclusion
Parameter validation is a crucial aspect of writing robust and reliable JavaScript code. By implementing the techniques discussed in this article, you can prevent errors, enhance security, and ensure that your functions behave as expected. Whether you choose to perform basic type checking, leverage custom validation functions, or utilize powerful libraries, the key is to make validation a standard practice in your development process.
Further Reading:
Table of Contents
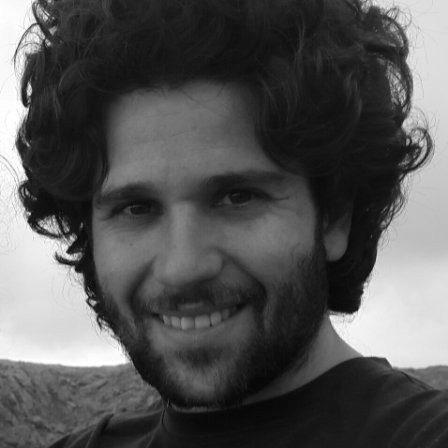
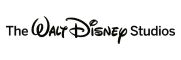