JavaScript Function Polymorphism: Adapting to Different Inputs
JavaScript is a versatile language known for its flexibility and dynamic nature. One of the key features that enhance its flexibility is function polymorphism. Function polymorphism allows functions to handle different types or numbers of inputs, adapting their behavior accordingly. This article delves into JavaScript function polymorphism, providing practical examples and techniques for creating flexible and robust functions.
Understanding Function Polymorphism
Function polymorphism refers to the ability of a function to operate in different ways based on the inputs it receives. This concept is crucial for writing code that can handle various scenarios and adapt to different data types or quantities of arguments. By utilizing polymorphism, developers can create functions that are both versatile and maintainable.
Implementing Function Polymorphism in JavaScript
1. Handling Different Types of Inputs
JavaScript functions can be designed to process different types of inputs by using type checking and conditional logic. This approach allows a single function to handle multiple data types.
Example: Processing Different Data Types
```javascript function processInput(input) { if (typeof input === 'string') { console.log(`String input: ${input.toUpperCase()}`); } else if (typeof input === 'number') { console.log(`Number input: ${input 2}`); } else { console.log('Unknown input type'); } } processInput('hello'); // Output: String input: HELLO processInput(5); // Output: Number input: 10 ```
2. Using Default Parameters for Flexibility
JavaScript functions can be made more flexible by using default parameters. This allows functions to provide default values for parameters that are not explicitly provided.
Example: Default Parameters
```javascript function greet(name = 'Guest', greeting = 'Hello') { console.log(`${greeting}, ${name}!`); } greet(); // Output: Hello, Guest! greet('Alice'); // Output: Hello, Alice! greet('Bob', 'Welcome'); // Output: Welcome, Bob! ```
3. Supporting Variable Numbers of Arguments
JavaScript functions can handle a variable number of arguments using the `arguments` object or the rest parameter syntax. This enables functions to process an arbitrary number of inputs.
Example: Using Rest Parameters
```javascript function sum(...numbers) { return numbers.reduce((total, num) => total + num, 0); } console.log(sum(1, 2, 3)); // Output: 6 console.log(sum(5, 10, 15, 20)); // Output: 50 ```
4. Overloading Functions Using Object Mapping
JavaScript does not support function overloading directly. However, function overloading can be simulated using object mapping to handle different scenarios based on input properties.
Example: Simulating Overloading
```javascript function calculate(value, options = {}) { const { operation = 'add', factor = 1 } = options; if (operation === 'add') { return value + factor; } else if (operation === 'multiply') { return value factor; } else { throw new Error('Unknown operation'); } } console.log(calculate(10, { operation: 'add', factor: 5 })); // Output: 15 console.log(calculate(10, { operation: 'multiply', factor: 3 })); // Output: 30 ```
Conclusion
JavaScript function polymorphism enables developers to write versatile and adaptable code. By handling different types of inputs, utilizing default parameters, supporting variable arguments, and simulating overloading, developers can create robust functions that cater to various use cases. Leveraging these techniques leads to more flexible and maintainable code, enhancing overall development efficiency.
Further Reading:
Table of Contents
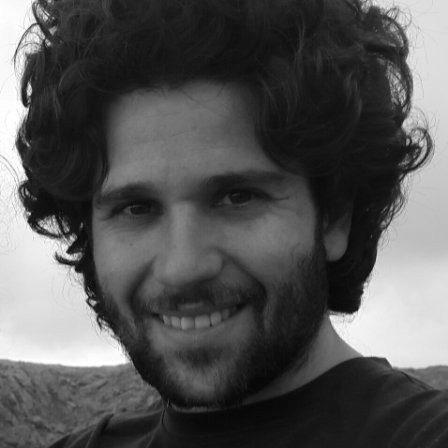
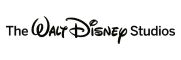