Handling Asynchronous Operations with JavaScript Promise Functions
In the world of modern web development, asynchronous programming is a fundamental concept. With the rise of complex applications and user interactions, the need to manage asynchronous operations efficiently has become paramount. JavaScript’s Promise functions provide a powerful way to handle asynchronous tasks in a clean and organized manner. In this blog post, we’ll dive into the world of Promise functions, exploring what they are, how they work, and best practices for effectively using them to handle asynchronous operations.
Table of Contents
1. Understanding Asynchronous Programming
1.1. The Need for Asynchronous Operations
In JavaScript, many tasks take time to complete, such as fetching data from a server, reading files, or waiting for user input. If these tasks were executed synchronously, they could block the entire program, leading to unresponsive user interfaces and poor user experiences.
1.2. Callback Hell: The Old Approach
Early attempts to manage asynchronous code often resulted in a nesting pattern known as “Callback Hell.” Asynchronous functions were passed as callbacks to other functions, leading to deeply nested code that was hard to read, maintain, and debug.
javascript getUserData(function(userData) { getPosts(userData.id, function(posts) { getComments(posts[0].id, function(comments) { // More nested callbacks... }); }); });
1.3. Introducing Promises: A Better Solution
Promises were introduced to address the issues of callback hell. A Promise is an object representing the eventual completion or failure of an asynchronous operation. It provides a more structured way to handle asynchronous code and brings readability and maintainability to the codebase.
2. Exploring JavaScript Promises
2.1. Creating a Promise
A Promise can be created using the Promise constructor. It takes a function with two parameters: resolve and reject. The resolve function is called when the asynchronous operation succeeds, and the reject function is called when it fails.
javascript const fetchData = new Promise((resolve, reject) => { // Simulate an asynchronous operation setTimeout(() => { const data = { id: 1, name: "John Doe" }; resolve(data); // Operation succeeded // reject("Error fetching data"); // Operation failed }, 1000); });
2.2. Promise States: Pending, Fulfilled, Rejected
A Promise has three possible states:
- Pending: The initial state, neither fulfilled nor rejected.
- Fulfilled: The asynchronous operation completed successfully, and the promise now has a value.
- Rejected: The asynchronous operation failed, and the promise now has a reason for the failure.
javascript fetchData .then(data => { console.log("Fulfilled:", data); }) .catch(error => { console.error("Rejected:", error); });
2.3. Chaining Promises for Sequential Operations
Promises can be chained to perform sequential operations. Each .then() returns a new Promise, allowing you to create a sequence of asynchronous tasks.
javascript fetchData .then(data => { return getPosts(data.id); // Returns a promise for getting posts }) .then(posts => { return getComments(posts[0].id); // Returns a promise for getting comments }) .then(comments => { console.log("Comments:", comments); }) .catch(error => { console.error("Error:", error); });
2.4. Handling Errors with catch()
The catch() method is used to handle errors in a promise chain. If any promise in the chain is rejected, the control will jump directly to the nearest catch() block.
javascript fetchData .then(data => { return getPosts(data.id); }) .then(posts => { // Simulate an error throw new Error("Error fetching comments"); }) .catch(error => { console.error("Error:", error); // Handle the error });
3. Working with Multiple Promises
3.1. Promise.all(): Running Multiple Promises in Parallel
The Promise.all() method takes an array of promises and returns a new promise that resolves with an array of resolved values from the input promises. This is useful when you want to perform multiple independent asynchronous operations in parallel.
javascript const promise1 = fetchData(); const promise2 = fetchPosts(); Promise.all([promise1, promise2]) .then(results => { console.log("All promises resolved:", results); }) .catch(error => { console.error("Error:", error); });
3.2. Promise.race(): Getting the Result of the Fastest Promise
The Promise.race() method returns a new promise that resolves or rejects as soon as the first promise in the input array resolves or rejects. This is handy when you want to execute a task multiple times and take the result of the first successful one.
javascript const timeoutPromise = new Promise((resolve, reject) => { setTimeout(() => { reject("Timeout exceeded"); }, 500); }); const fetchDataPromise = fetchData(); Promise.race([fetchDataPromise, timeoutPromise]) .then(result => { console.log("First promise resolved:", result); }) .catch(error => { console.error("Error:", error); });
3.3. Promise.allSettled(): Handling Multiple Promises Regardless of Outcome
The Promise.allSettled() method returns a promise that resolves with an array of results from all the input promises, whether they were fulfilled or rejected. This is useful when you want to handle all the outcomes without stopping the execution due to a rejection.
javascript const promise1 = fetchData(); const promise2 = fetchPosts(); Promise.allSettled([promise1, promise2]) .then(results => { console.log("All promises settled:", results); });
4. Async/Await: Syntactic Sugar for Promises
4.1. Writing Asynchronous Code in a Synchronous Style
Async/await is a more modern and elegant way to work with promises. The async keyword is used to define a function as asynchronous, and the await keyword is used to pause the execution of a function until a promise is resolved.
javascript async function fetchDataAndPosts() { try { const data = await fetchData(); const posts = await fetchPosts(); console.log("Data:", data); console.log("Posts:", posts); } catch (error) { console.error("Error:", error); } }
4.2. Error Handling with Try/Catch
When using async/await, error handling can be done using a traditional try/catch block, providing a more familiar structure.
5. Best Practices for Using Promise Functions
5.1. Avoiding the Pyramid of Doom
Promise functions are designed to eliminate the “Pyramid of Doom,” a situation where multiple nested callbacks result in unreadable and hard-to-maintain code.
5.2. Breaking Down Complex Tasks into Smaller Promises
Instead of creating large monolithic promise chains, break down complex tasks into smaller, manageable promises. This improves readability and makes debugging easier.
5.3. Proper Error Handling to Prevent Silent Failures
Always include error handling in your promise chains to ensure that failures are not silently ignored. Use .catch() or try/catch blocks to handle errors appropriately.
5.4. Cleaning Up Resources with finally()
The finally() method can be added to a promise chain to ensure that a certain piece of code is executed regardless of whether the promise is fulfilled or rejected. This is useful for cleaning up resources or performing final actions.
Conclusion
In conclusion, mastering asynchronous programming is crucial for writing efficient and responsive JavaScript applications. Promise functions offer an elegant solution to handling asynchronous operations, reducing callback hell and making code more readable. By understanding the concepts of promises, chaining, error handling, and leveraging async/await, you can build maintainable and robust asynchronous code. Remember to follow best practices to ensure your code remains clean, maintainable, and free from common pitfalls. Happy coding!
Asynchronous programming is an essential skill for modern JavaScript developers. By understanding and effectively using Promise functions, you can create more responsive and efficient applications while keeping your codebase organized and maintainable. Whether you’re fetching data from an API, handling user interactions, or managing complex workflows, mastering asynchronous operations will significantly enhance your web development capabilities.
Table of Contents
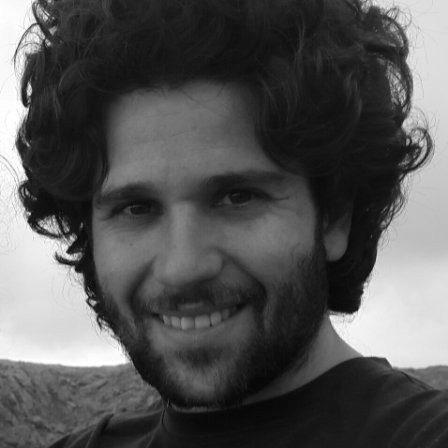
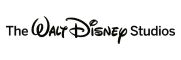