JavaScript Function Signatures: Defining and Using Function Types
Function signatures in JavaScript play a critical role in defining how functions are structured and used. By clearly specifying the types of inputs and outputs for functions, you can write more robust, readable, and maintainable code. This article explores how to define and use function types in JavaScript, offering practical examples to demonstrate these concepts.
Understanding Function Signatures
A function signature refers to the type and number of arguments a function accepts and the type of value it returns. In strongly-typed languages like TypeScript, function signatures are explicit, but in JavaScript, which is dynamically typed, developers can still benefit from adopting similar practices to avoid errors and ensure consistency.
Defining Function Types in JavaScript
Even though JavaScript is not statically typed, you can use comments, tools like JSDoc, or TypeScript (a superset of JavaScript) to define and enforce function types. Here’s how you can define function signatures using different approaches.
Example: Using JSDoc to Define Function Signatures
JSDoc allows you to annotate JavaScript code with comments that define types, making it easier for developers to understand the expected inputs and outputs of a function.
```javascript / * Adds two numbers together. * @param {number} a - The first number. * @param {number} b - The second number. * @returns {number} The sum of the two numbers. */ function add(a, b) { return a + b; } ```
By defining the types of `a` and `b` as `number`, and specifying that the function returns a `number`, you make the function’s signature clear.
Function Overloading in JavaScript
While function overloading is not natively supported in JavaScript as it is in languages like Java or C#, you can simulate it by using optional parameters or by checking the types and number of arguments at runtime.
Example: Simulating Function Overloading
```javascript function greet(name, age) { if (typeof age === 'undefined') { return `Hello, ${name}!`; } else { return `Hello, ${name}, you are ${age} years old!`; } } console.log(greet("Alice")); // Hello, Alice! console.log(greet("Bob", 30)); // Hello, Bob, you are 30 years old! ```
In this example, the `greet` function handles two different scenarios based on whether the `age` parameter is provided, effectively mimicking function overloading.
Using Arrow Functions with Type Annotations
Arrow functions offer a concise syntax in JavaScript, and you can still use JSDoc or TypeScript annotations to define their signatures.
Example: Annotating Arrow Functions
```javascript / * Multiplies two numbers. * @param {number} x - The first number. * @param {number} y - The second number. * @returns {number} The product of x and y. */ const multiply = (x, y) => x * y; console.log(multiply(3, 4)); // 12 ```
This approach ensures that even with the compact syntax of arrow functions, the expected types are clear.
Leveraging TypeScript for Function Signatures
For projects where type safety is paramount, TypeScript can be used to define function signatures explicitly. TypeScript’s type system allows for a richer definition of function types, including specifying optional parameters, return types, and even the structure of objects passed to functions.
Example: Function Signatures in TypeScript
```typescript function divide(a: number, b: number): number { if (b === 0) throw new Error("Division by zero is not allowed"); return a / b; } console.log(divide(10, 2)); // 5 ```
Here, TypeScript enforces that `a` and `b` are numbers and that the function returns a `number`, providing an additional layer of safety.
Conclusion
Defining and using function signatures in JavaScript, whether through JSDoc annotations, TypeScript, or careful coding practices, can greatly enhance code quality. By clearly stating the expected types and behavior of functions, you reduce the risk of bugs and make your code more maintainable and easier to understand.
Further Reading:
Table of Contents
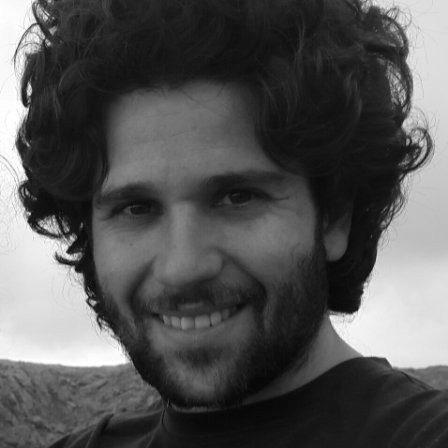
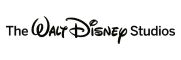