JavaScript Function Testing: Strategies and Tools for Quality Assurance
In the fast-paced world of software development, ensuring the quality and reliability of your code is paramount. JavaScript, being a dynamic and widely used language, requires rigorous testing to maintain code integrity. This article explores strategies and tools for testing JavaScript functions, offering practical examples and best practices for achieving robust quality assurance.
The Importance of Testing JavaScript Functions
JavaScript functions form the backbone of any application, handling everything from simple logic to complex operations. Properly testing these functions ensures that your application behaves as expected, reducing bugs and improving maintainability.
Strategies for Testing JavaScript Functions
1. Unit Testing
Definition: Unit testing involves testing individual functions in isolation to ensure they perform as expected.
Best Practices: Focus on testing one aspect of the function at a time, mocking external dependencies, and writing tests that cover both expected and edge cases.
Example: Testing a Simple Function
```javascript function add(a, b) { return a + b; } // Test case test('adds 1 + 2 to equal 3', () => { expect(add(1, 2)).toBe(3); }); ```
2. Integration Testing
Definition: Integration testing checks how multiple functions or modules work together.
Best Practices: Test the interaction between different functions or components, and use real or mock data to simulate real-world scenarios.
Example: Testing Function Interactions
```javascript function fetchData(callback) { setTimeout(() => { callback('data'); }, 1000); } function processData(data) { return data.toUpperCase(); } // Test case test('fetches and processes data', done => { function callback(data) { expect(processData(data)).toBe('DATA'); done(); } fetchData(callback); }); ```
3. End-to-End Testing
Definition: End-to-end (E2E) testing simulates user interactions with the entire application, ensuring the complete system works as intended.
Best Practices: Automate E2E tests to cover critical user flows, and use tools like Cypress to streamline the process.
Example: Testing User Flow
```javascript describe('User Login', () => { it('should allow user to log in', () => { cy.visit('/login'); cy.get('input[name="username"]').type('user'); cy.get('input[name="password"]').type('password'); cy.get('button[type="submit"]').click(); cy.url().should('include', '/dashboard'); }); }); ```
Tools for JavaScript Function Testing
1. Jest
Overview: Jest is a popular testing framework by Facebook that provides a comprehensive solution for testing JavaScript applications.
Features: Snapshot testing, parallel execution, and built-in mocking.
Example: Using Jest for Unit Testing
```javascript test('multiplies 2 3 to equal 6', () => { expect(2 3).toBe(6); }); ```
2. Mocha
Overview: Mocha is a flexible testing framework that works well with various assertion libraries.
Features: Asynchronous testing, support for multiple reporters, and wide community adoption.
Example: Using Mocha for Testing
```javascript const assert = require('assert'); describe('Array', function() { describe('indexOf()', function() { it('should return -1 when the value is not present', function() { assert.equal([1, 2, 3].indexOf(4), -1); }); }); }); ```
3. Chai
Overview: Chai is an assertion library that can be used with Mocha to provide expressive and readable assertions.
Features: BDD/TDD assertion styles, chainable language, and plugins.
Example: Using Chai with Mocha
```javascript const expect = require('chai').expect; describe('String', function() { it('should return the correct length', function() { expect('Hello').to.have.lengthOf(5); }); }); ```
Conclusion
Testing JavaScript functions is a critical component of software development, ensuring that your code is reliable, maintainable, and free of bugs. By leveraging the strategies and tools outlined in this article, you can enhance the quality of your JavaScript applications and deliver a better user experience.
Further Reading:
Table of Contents
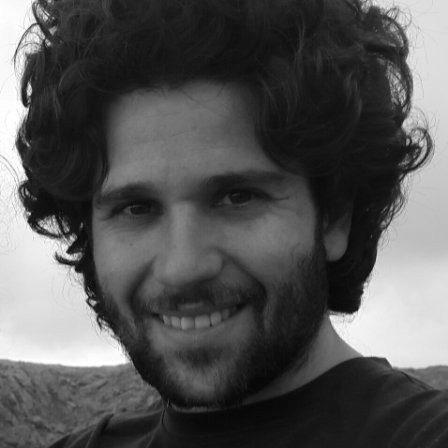
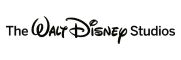