JavaScript Function Best Practices: Writing Efficient and Maintainable Code
JavaScript functions are the building blocks of any web application, making it essential to follow best practices to ensure your code is efficient, maintainable, and scalable. In this blog, we’ll explore some of the most important best practices for writing JavaScript functions, with practical examples to help you implement these techniques in your projects.
Understanding the Importance of Function Best Practices
Following best practices in writing JavaScript functions not only improves code readability but also enhances performance, making your applications more reliable and easier to maintain. These practices are essential whether you’re working on a small script or a large-scale application.
1. Keep Functions Small and Focused
A well-designed function should do one thing and do it well. Keeping your functions small and focused improves readability and makes testing and debugging easier.
Example: Splitting a Large Function into Smaller Ones
Instead of having one large function that handles multiple tasks, split it into smaller, more focused functions.
```javascript function processUserData(userData) { const validatedData = validateData(userData); const processedData = processData(validatedData); saveData(processedData); } function validateData(data) { // Validation logic return data; } function processData(data) { // Processing logic return data; } function saveData(data) { // Save logic } ```
2. Use Descriptive Function Names
Function names should be clear and descriptive, reflecting what the function does. This practice makes your code more understandable and easier to maintain.
Example: Naming Functions Clearly
Instead of using vague names like `doStuff`, use descriptive names like `calculateTotalPrice` or `fetchUserData`.
```javascript function calculateTotalPrice(items) { return items.reduce((total, item) => total + item.price, 0); } ```
3. Avoid Side Effects
Functions should ideally be pure, meaning they don’t cause side effects. A pure function always returns the same result for the same inputs and doesn’t alter any external state.
Example: Writing a Pure Function
```javascript function add(a, b) { return a + b; // No side effects } // Impure function example let counter = 0; function incrementCounter() { counter++; // Alters external state } ```
4. Use Default Parameters
JavaScript allows you to set default parameter values, which can simplify your functions and make them more flexible.
Example: Using Default Parameters
```javascript function greet(name = "Guest") { console.log(`Hello, ${name}!`); } greet(); // "Hello, Guest!" greet("Alice"); // "Hello, Alice!" ```
5. Leverage Arrow Functions
Arrow functions offer a concise syntax and lexical `this` binding, making them a powerful tool in modern JavaScript development.
Example: Using Arrow Functions
```javascript const multiply = (a, b) => a * b; const numbers = [1, 2, 3, 4]; const squares = numbers.map(n => n * n); ```
6. Implement Function Composition
Function composition involves combining multiple functions to create a new function. This technique promotes code reusability and readability.
Example: Function Composition with Arrow Functions
```javascript const add = a => b => a + b; const multiply = a => b => a * b; const addAndMultiply = (a, b, c) => multiply(add(a)(b))(c); console.log(addAndMultiply(2, 3, 4)); // Output: 20 ```
7. Handle Errors Gracefully
Implementing error handling within your functions ensures that your code can handle unexpected situations without crashing.
Example: Using Try-Catch for Error Handling
8. Document Your Functions
Adding comments and JSDoc annotations to your functions provides clarity for other developers (and future you), making your code easier to understand and maintain.
Example: Using JSDoc for Documentation
```javascript /** * Adds two numbers together. * @param {number} a - The first number. * @param {number} b - The second number. * @returns {number} The sum of the two numbers. */ function add(a, b) { return a + b; } ```
Conclusion
By following these JavaScript function best practices, you can write more efficient, maintainable, and scalable code. From keeping your functions small and focused to leveraging modern JavaScript features like arrow functions and default parameters, these techniques will help you become a better JavaScript developer.
Further Reading:
Table of Contents
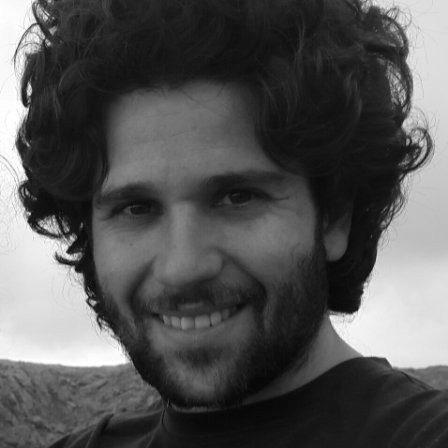
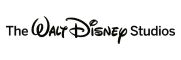