How do you define a class in Kotlin?
In Kotlin, a class is a blueprint for creating objects, which are instances of the class. Classes encapsulate data and behavior related to a specific entity or concept in the application domain. They serve as templates for creating objects with common properties and behaviors.
To define a class in Kotlin, you use the class keyword followed by the class name and an optional constructor. Here’s a simple example of a class representing a Person with name and age properties:
kotlin class Person(val name: String, val age: Int)
In this example, Person is the name of the class, and it has two properties: name of type String and age of type Int. The val keyword before each property indicates that they are immutable and have getter methods automatically generated.
Kotlin also supports secondary constructors, initialization blocks, and visibility modifiers like private, protected, and internal for class members.
You can create instances of a class using the new keyword, although it’s optional in Kotlin. For example:
kotlin val person = Person("John", 30)
This creates a new Person object with the specified name and age.
Classes in Kotlin can also contain methods, nested classes, properties, and companion objects, providing flexibility in organizing and modeling complex systems.
Defining classes in Kotlin follows a concise and expressive syntax, promoting readability and maintainability of codebases. Classes serve as fundamental building blocks for creating object-oriented designs and modeling entities in Kotlin applications.
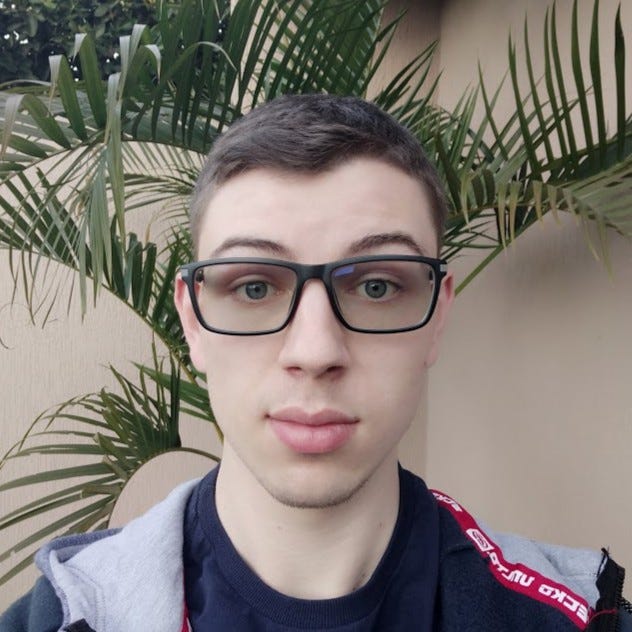
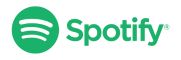