What is an abstract class in Kotlin?
An abstract class in Kotlin acts as a template or blueprint for other classes, offering a common structure and behavior that can be inherited by its subclasses. Unlike concrete classes, abstract classes cannot be instantiated directly; instead, they are meant to be extended and serve as a foundation for more specific implementations.
One of the primary features of an abstract class is the ability to define abstract methods. These methods are declared without providing an implementation in the abstract class itself. Instead, subclasses must override these abstract methods and provide their own implementation.
Abstract classes may also contain concrete methods, which are methods with defined behavior. These concrete methods can be used directly by subclasses without requiring overriding. This combination of abstract and concrete methods allows abstract classes to provide both common functionality and flexibility for subclasses to customize behavior as needed.
Abstract classes are particularly useful when defining a family of related classes that share common characteristics and behavior. By defining a common interface through an abstract class, developers can promote code reuse and maintain consistency across different implementations.
To define an abstract class in Kotlin, you use the abstract keyword before the class declaration. Here’s a simple example of an abstract class named Shape:
kotlin abstract class Shape { abstract fun calculateArea(): Double // Abstract method fun display() { println("This is a shape.") } }
In this example, the Shape class declares an abstract method calculateArea() without providing an implementation. Additionally, it contains a concrete method display() that prints a message indicating that it is a shape.
Subclasses of the Shape class must provide their own implementation of the calculateArea() method. For example, a Circle class and a Rectangle class extending Shape would each provide their own logic for calculating the area specific to their shape.
Abstract classes in Kotlin provide a way to define common structure and behavior for a group of related classes. They enable code reuse, promote consistency, and allow for flexibility in customizing behavior through method overriding. By leveraging abstract classes, developers can create more modular, maintainable, and scalable codebases.
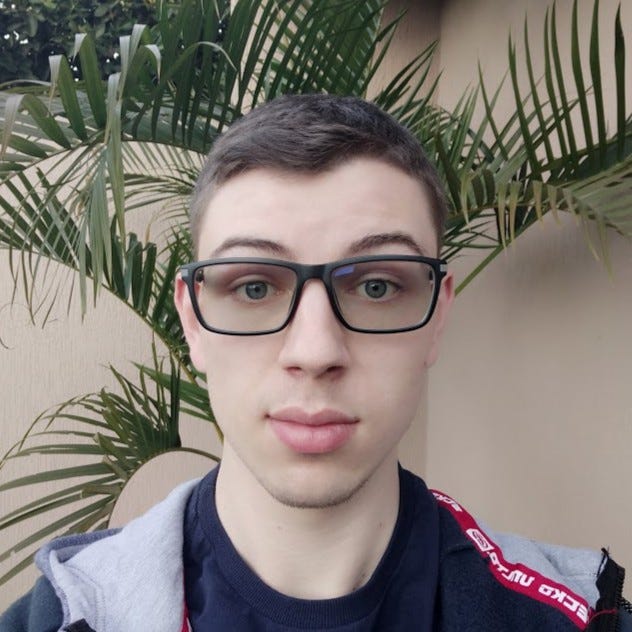
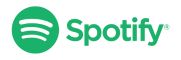