Exploring Kotlin Android Extensions: Simplify Your UI Development
When it comes to Android app development, creating a smooth and visually appealing user interface (UI) is of utmost importance. However, UI development can often be complex and time-consuming, involving multiple lines of code to access and manipulate UI elements. Kotlin Android Extensions offer a solution to this problem, simplifying the process and making UI development more efficient.
In this blog, we will delve into Kotlin Android Extensions and explore how they can dramatically improve your Android app development experience. From accessing UI elements to handling click events, Kotlin Android Extensions provide an expressive and concise syntax that reduces boilerplate code and minimizes the chances of errors.
1. What are Kotlin Android Extensions?
Kotlin Android Extensions are a set of Kotlin compiler plugins provided by the Kotlin Android Gradle plugin. They allow you to access views from your XML layout files directly in your Kotlin code, without the need for findViewById() or data binding. This feature not only reduces the amount of code you need to write but also enhances the readability of your code.
2. Setting Up Kotlin Android Extensions
To start using Kotlin Android Extensions in your Android project, you need to make sure you have the following in place:
- Kotlin Plugin: Ensure that you have the Kotlin plugin configured in your Android project’s build.gradle file.
gradle plugins { id 'com.android.application' id 'kotlin-android' }
- Kotlin Android Extensions Plugin: Add the Kotlin Android Extensions plugin to your app module’s build.gradle file.
gradle android { // ... } plugins { // ... id 'kotlin-android-extensions' }
Once these configurations are set up, you are ready to explore the world of Kotlin Android Extensions and simplify your UI development process.
3. Accessing Views with Kotlin Android Extensions
One of the primary benefits of using Kotlin Android Extensions is the ability to access views directly in your Kotlin code. Instead of using findViewById() to locate and reference views, you can simply refer to them by their IDs in your Kotlin code.
Let’s say you have a layout file called activity_main.xml containing a TextView and a Button:
xml <!-- activity_main.xml --> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:id="@+id/textViewWelcomeMessage" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello, welcome to Kotlin Android Extensions!" android:textSize="18sp" android:layout_centerInParent="true"/> <Button android:id="@+id/buttonClickMe" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Click Me!" android:layout_below="@id/textViewWelcomeMessage" android:layout_centerHorizontal="true"/> </RelativeLayout>
In your Kotlin code, you can access these views as simple properties. Here’s how you do it:
kotlin import kotlinx.android.synthetic.main.activity_main.* class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // Accessing the TextView and Button using Kotlin Android Extensions textViewWelcomeMessage.text = "Welcome to my app!" buttonClickMe.setOnClickListener { // Handle the button click event here } } }
As you can see, with Kotlin Android Extensions, you directly access the views by their IDs (the android:id attributes from the XML) without the need for any extra setup. This simplifies your code, makes it more readable, and reduces the chance of NullPointerExceptions that might occur with findViewById().
4. Handling Click Events with Kotlin Android Extensions
In addition to simplifying the process of accessing views, Kotlin Android Extensions also make handling click events more straightforward. Instead of setting click listeners with setOnClickListener() as shown in the previous example, you can use the onClick attribute directly in your XML layout.
Let’s update the activity_main.xml layout to use the onClick attribute for the Button:
xml <!-- activity_main.xml --> <RelativeLayout ... <Button android:id="@+id/buttonClickMe" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Click Me!" android:layout_below="@id/textViewWelcomeMessage" android:layout_centerHorizontal="true" android:onClick="onButtonClick"/> </RelativeLayout>
In your Kotlin code, you can now define the onButtonClick function to handle the click event:
kotlin import kotlinx.android.synthetic.main.activity_main.* class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) } // Click event handler for the Button fun onButtonClick(view: View) { // Handle the button click event here } }
By using the onClick attribute in the XML layout, you no longer need to set the click listener programmatically. This approach improves the separation of concerns and makes your code cleaner.
5. Handling Nullable Views with Kotlin Android Extensions
In some cases, you might have views in your layout that are not always present or visible. For example, you might have a conditional layout that includes or excludes certain views based on certain conditions.
When using Kotlin Android Extensions, you may encounter a situation where the view is nullable if it’s not present in the layout. To handle such cases, Kotlin provides a safe access operator (?.) that allows you to work with nullable views safely.
Let’s consider an example where we have a TextView that is conditionally included in the layout:
xml <!-- activity_main.xml --> <RelativeLayout ... <TextView android:id="@+id/textViewOptional" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="This is optional!" android:layout_below="@id/buttonClickMe" android:layout_centerHorizontal="true" android:visibility="gone"/> </RelativeLayout>
The android:visibility=”gone” attribute ensures that the TextView is initially not visible when the layout is inflated. Now, in your Kotlin code, you can handle this nullable view using the safe access operator:
kotlin import kotlinx.android.synthetic.main.activity_main.* class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // Accessing the optional TextView using the safe access operator textViewOptional?.text = "This is now visible!" textViewOptional?.visibility = View.VISIBLE } }
By using the safe access operator (?.), you can ensure that you only manipulate the view if it’s present in the layout. This helps you avoid unnecessary null checks and makes your code more concise.
6. Using Kotlin Android Extensions in Fragments
So far, we’ve explored using Kotlin Android Extensions in activities. But what if you have fragments in your Android app? The good news is that Kotlin Android Extensions can be used seamlessly in fragments as well.
When using Kotlin Android Extensions in fragments, the process is similar to what we’ve seen in activities. Let’s create a sample fragment called MyFragment and access views from its layout:
kotlin import kotlinx.android.synthetic.main.fragment_my.* class MyFragment : Fragment(R.layout.fragment_my) { override fun onViewCreated(view: View, savedInstanceState: Bundle?) { super.onViewCreated(view, savedInstanceState) // Accessing views from the fragment's layout textViewWelcomeMessage.text = "Welcome to my fragment!" buttonClickMe.setOnClickListener { // Handle the button click event here } } }
In this example, we access the views in the fragment’s layout (fragment_my.xml) in the same way we did for activities. The process is consistent, making it easy to work with UI elements in both activities and fragments.
7. Performance Considerations
While Kotlin Android Extensions provide a more concise and readable syntax for accessing views, it’s essential to consider their impact on performance. Under the hood, Kotlin Android Extensions generate extension properties for each view in your layout, which could lead to a slight increase in the APK size.
However, the performance impact is usually negligible for most apps. In situations where optimizing APK size is a top priority, you might consider using data binding or view binding instead, as they provide better control over the generated code.
Conclusion
Kotlin Android Extensions can significantly simplify your UI development in Android apps. By eliminating the need for boilerplate code and providing a more expressive syntax, they enhance code readability and reduce the chances of errors. Moreover, with Kotlin Android Extensions, handling click events becomes more straightforward, and you can safely work with nullable views.
By incorporating Kotlin Android Extensions into your Android development workflow, you can streamline your UI development process, boost productivity, and create more elegant and user-friendly apps. Give it a try in your next Android project and experience the power of Kotlin Android Extensions firsthand! Happy coding!
Remember, while Kotlin Android Extensions can greatly enhance your UI development, always consider the specific needs and requirements of your app before choosing the best approach for accessing and manipulating views. Happy coding and best of luck with your Android app development journey!
Table of Contents
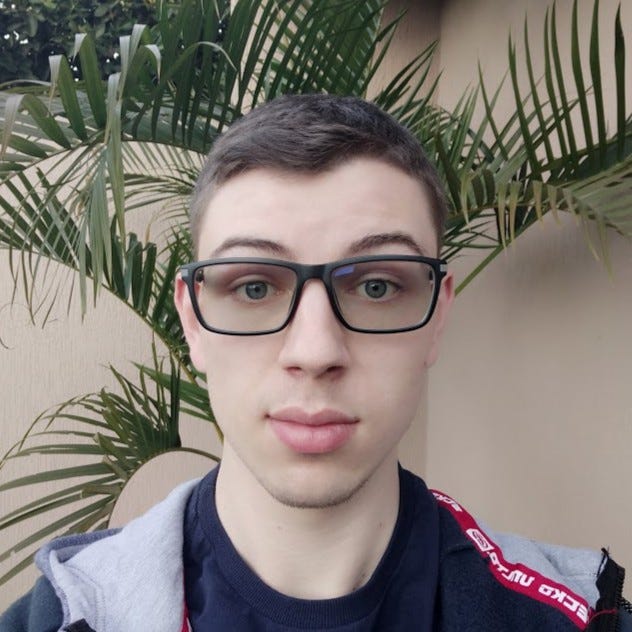
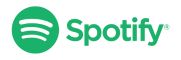