Kotlin + Jetpack: The Unbeatable Combo Every Android Developer Must Know
With the ever-evolving world of Android development, developers are always on the lookout for tools and frameworks that can enhance their productivity, reduce boilerplate, and simplify complex tasks. Two of the most remarkable advancements in recent Android development are Kotlin and Android Jetpack. When combined, they revolutionize the way developers create robust, maintainable, and efficient Android apps. Let’s dive into how Kotlin and Android Jetpack can supercharge your app development.
1. Kotlin: A Modern Language for Android Development
1.1. Concise and Expressive
The major advantage Kotlin brings to the table is its concise and expressive syntax. For instance, consider defining a data class.
Java:
```java public class User { private String name; private int age; public User(String name, int age) { this.name = name; this.age = age; } // getters, setters, equals(), hashCode(), and toString() omitted for brevity. } ```
Kotlin:
```kotlin data class User(val name: String, val age: Int) ```
Kotlin reduces the boilerplate considerably, making code more readable and maintainable.
2. Null Safety
Kotlin’s type system is designed to eliminate the null reference errors by making all types non-nullable by default.
```kotlin var name: String = "John" name = null // Compilation error ```
3. Extension Functions
Extend the functionality of existing classes without modifying their code.
```kotlin fun String.shout(): String = this.toUpperCase() + "!!!" println("hello".shout()) // Outputs: HELLO!!! ```
4. Android Jetpack: A Suite of Libraries and Tools
Jetpack encompasses a wide array of libraries and tools, each designed to solve specific Android development challenges. Let’s discuss some key components.
4.1. LiveData and ViewModel
A classic problem in Android development is handling lifecycle changes (like screen rotations). LiveData and ViewModel provide a solution.
ViewModel retains data across configuration changes, ensuring your UI data survives.
LiveData is an observable data holder. It’s lifecycle-aware, meaning it updates only when the associated view (activity or fragment) is in an active state.
```kotlin class UserViewModel : ViewModel() { private val _user = MutableLiveData<User>() val user: LiveData<User> get() = _user fun fetchUser() { // Logic to fetch user _user.value = fetchedUser } } ```
4.2. Room Database
Room provides an abstraction layer over SQLite, allowing for more robust database access and easier management.
```kotlin @Entity data class UserEntity( @PrimaryKey val uid: Int, @ColumnInfo(name = "first_name") val firstName: String, @ColumnInfo(name = "last_name") val lastName: String ) @Dao interface UserDao { @Query("SELECT * FROM user") fun getAll(): List<UserEntity> @Insert fun insertAll(vararg users: UserEntity) } @Database(entities = [UserEntity::class], version = 1) abstract class AppDatabase : RoomDatabase() { abstract fun userDao(): UserDao } ```
5. Navigation Component
Navigation component simplifies the implementation of navigation between different screens (fragments) in your app.
Navigation Graph:
```xml <navigation xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto"> <fragment android:id="@+id/firstFragment" android:name="com.example.FirstFragment"> <action android:id="@+id/action_next" app:destination="@id/secondFragment" /> </fragment> <fragment android:id="@+id/secondFragment" android:name="com.example.SecondFragment" /> </navigation> ```
In Kotlin:
```kotlin findNavController().navigate(R.id.action_next) ```
6. WorkManager
For background tasks that need to run periodically or under certain conditions, WorkManager is a lifesaver.
```kotlin val workRequest = OneOffWorkRequestBuilder<MyWorker>().build() WorkManager.getInstance().enqueue(workRequest) ```
7. Combining Kotlin with Jetpack
Kotlin’s powerful language features combined with Jetpack’s robust libraries ensure a streamlined development experience. For instance, Kotlin’s coroutines work seamlessly with Jetpack components like Room and LiveData, allowing for more readable asynchronous code.
```kotlin @Query("SELECT * FROM user") suspend fun getAll(): List<UserEntity> ```
This seamless integration between Kotlin and Jetpack makes asynchronous operations on the main thread efficient and straightforward.
Conclusion
Kotlin and Android Jetpack, when used in conjunction, provide a modern, efficient, and streamlined way to develop Android apps. Kotlin simplifies the language side with its concise and expressive syntax, while Jetpack offers a plethora of tools and libraries addressing common app development challenges. Together, they supercharge Android app development, resulting in better apps in shorter development times. Whether you’re a novice or a seasoned developer, embracing Kotlin and Android Jetpack will undoubtedly elevate your Android development game.
Table of Contents
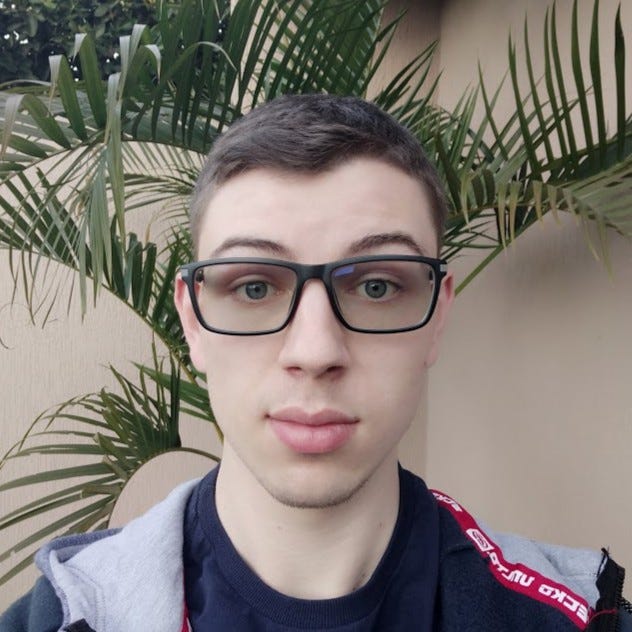
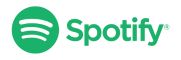