Android Testing with Kotlin: Ensuring Quality in Your Apps
In today’s fast-paced world, where mobile applications have become an integral part of our lives, ensuring the quality of Android apps is paramount. Users expect apps to be bug-free, performant, and reliable. This is where Android testing comes into play. Testing your Android apps using Kotlin provides a solid foundation for delivering high-quality applications. In this blog, we will explore the importance of Android testing, delve into various testing techniques, frameworks, and tools, and provide code samples to help you get started on your journey to building robust and reliable Android applications.
1. Why Android Testing Matters
As an Android developer, you may wonder why testing is crucial for your app development process. Here are some key reasons:
- Quality Assurance: Testing allows you to identify and fix bugs, ensuring a seamless user experience and minimizing negative reviews or uninstalls.
- Reliability: Rigorous testing helps in catching potential issues early on, leading to more reliable and stable applications.
- User Satisfaction: By delivering a bug-free app, you enhance user satisfaction and build trust, ultimately driving user retention and positive word-of-mouth recommendations.
2. Fundamentals of Android Testing
Before diving into specific testing techniques, it’s essential to understand the fundamentals of Android testing:
- Unit Testing: Unit tests focus on individual components or functions of your app, verifying their correctness in isolation. Kotlin, with its concise syntax and strong type system, is an excellent choice for writing unit tests.
Sample Code:
kotlin import org.junit.Test import org.junit.Assert.* class ExampleUnitTest { @Test fun addition_isCorrect() { assertEquals(4, 2 + 2) } }
- Instrumentation Testing: Instrumentation tests allow you to test your app’s behavior in a simulated environment, including user interactions and UI components. Android’s Instrumentation framework, combined with Kotlin, enables comprehensive testing of your app’s UI and integration with system components.
Sample Code:
kotlin import androidx.test.ext.junit.runners.AndroidJUnit4 import androidx.test.platform.app.InstrumentationRegistry import androidx.test.rule.ActivityTestRule import org.junit.Assert.* import org.junit.Rule import org.junit.Test import org.junit.runner.RunWith @RunWith(AndroidJUnit4::class) class ExampleInstrumentedTest { @Rule @JvmField val activityTestRule = ActivityTestRule(MainActivity::class.java) @Test fun validateTextViewText() { val appContext = InstrumentationRegistry.getInstrumentation().targetContext val activity = activityTestRule.activity val textView = activity.findViewById<TextView>(R.id.textView) assertEquals(appContext.getString(R.string.app_name), textView.text) } }
3. Testing Frameworks and Tools
To streamline your Android testing efforts, several frameworks and tools are available. Let’s explore a few popular choices:
- Espresso: Espresso is an Android testing framework that allows you to write concise and reliable UI tests. It offers a fluent API for simulating user interactions and verifying UI elements, making it an excellent choice for instrumented testing.
Sample Code:
kotlin @Test fun clickButton_updatesTextView() { onView(withId(R.id.button)).perform(click()) onView(withId(R.id.textView)).check(matches(withText("Button Clicked"))) }
- Mockito: Mockito is a powerful mocking framework that simplifies the creation of test doubles, such as stubs or mocks, to isolate dependencies in unit tests. It helps in testing components in isolation without relying on the actual implementations of their dependencies.
Sample Code:
kotlin @Test fun fetchData_success_displayData() { val mockApiService = mock(ApiService::class.java) val presenter = Presenter(mockApiService) // Stubbing the API response val responseData = TestData.getResponseData() `when`(mockApiService.getData()).thenReturn(Single.just(responseData)) presenter.fetchData() verify(mockApiService).getData() verify(mockView).displayData(responseData) }
4. Continuous Integration and Testing
Continuous Integration (CI) is an essential part of the software development process. Automating your testing workflows using CI tools, such as Jenkins, CircleCI, or Bitrise, ensures that your tests run consistently and reliably with every code change.
Sample Configuration (Bitrise.yml):
yaml workflows: test: steps: - script@1: inputs: - content: |- #!/usr/bin/env bash ./gradlew test
5. Best Practices for Android Testing
To make the most of your Android testing efforts, consider the following best practices:
- Test Early and Often: Start testing early in the development process and continue testing at regular intervals to catch issues early and prevent regression.
- Test Edge Cases: Ensure your tests cover various scenarios, including boundary conditions and exceptional cases, to validate your app’s behavior under different circumstances.
- Use Test Doubles: Employ mocks, stubs, and fakes to isolate dependencies and control their behavior, making your tests more deterministic and less reliant on external factors.
- Test Coverage: Aim for high test coverage to increase confidence in your codebase. Use tools like JaCoCo to measure and monitor your test coverage.
Conclusion
Android testing with Kotlin is essential for building high-quality, reliable, and bug-free applications. By embracing the testing fundamentals, leveraging testing frameworks and tools, integrating continuous testing, and following best practices, you can ensure that your apps meet user expectations and stand out in the competitive Android marketplace. So, start incorporating robust testing practices in your development workflow today and deliver top-notch Android apps that users love and trust.
Table of Contents
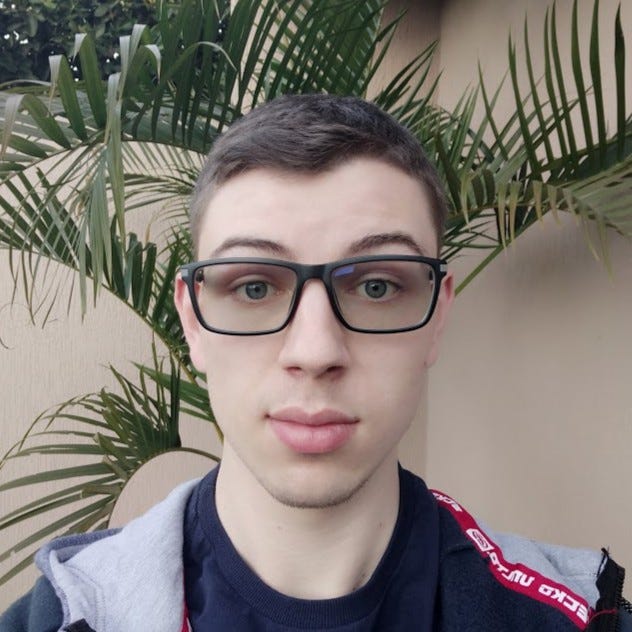
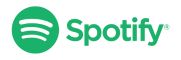