Seamless Android Development: The Synergy of Kotlin and AWS
Kotlin has firmly established itself as the language of choice for modern Android app development. AWS (Amazon Web Services), on the other hand, is a comprehensive cloud platform that offers a multitude of services for building, deploying, and managing applications in the cloud. When combined, Kotlin and AWS can help developers build cloud-powered Android apps with ease and efficiency.
In this blog post, we will delve into the integration of Kotlin with various AWS services to create robust Android applications.
1. Setting Up the AWS SDK for Kotlin
Before we can tap into the power of AWS, we must set up the AWS SDK for Kotlin. Begin by adding the required dependencies to your app’s `build.gradle.kts` or `build.gradle` file:
```kotlin implementation("software.amazon.awssdk:core:2.x.x") implementation("software.amazon.awssdk:services-<service_name>:2.x.x") ```
Replace `<service_name>` with the name of the AWS service you want to use.
2. AWS Cognito for User Authentication
User authentication is crucial for most apps. AWS Cognito provides secure user sign-up and sign-in services.
Example:
```kotlin val cognitoClient = CognitoIdentityProviderClient.builder().region(Region.US_EAST_1).build() val request = SignUpRequest.builder() .clientId("<YOUR_COGNITO_APP_CLIENT_ID>") .username("user@example.com") .password("password123") .build() val response = cognitoClient.signUp(request) ```
3. Storing Data with AWS DynamoDB
AWS DynamoDB is a NoSQL database service. Let’s say we’re building a note-taking app and want to store notes.
Example:
```kotlin val dynamoDbClient = DynamoDbClient.builder().region(Region.US_EAST_1).build() // Saving a note val item = mapOf( "UserId" to AttributeValue.builder().s("user@example.com").build(), "NoteId" to AttributeValue.builder().s(UUID.randomUUID().toString()).build(), "Content" to AttributeValue.builder().s("This is a note content").build() ) val putRequest = PutItemRequest.builder() .tableName("Notes") .item(item) .build() dynamoDbClient.putItem(putRequest) ```
4. Uploading Files to AWS S3
Imagine our note-taking app now allows attaching images. AWS S3 can store these images.
Example:
```kotlin val s3Client = S3Client.builder().region(Region.US_EAST_1).build() // Upload an image val requestBody = RequestBody.fromBytes("<BYTE_ARRAY_OF_YOUR_IMAGE>") val putObjectRequest = PutObjectRequest.builder() .bucket("your-s3-bucket-name") .key("path/to/image.jpg") .build() s3Client.putObject(putObjectRequest, requestBody) ```
5. AWS Lambda to Run Serverless Functions
Sometimes you need backend logic. Instead of setting up an entire server, you can use AWS Lambda with Kotlin.
Example:
You have a Lambda function to process images. Here’s how you’d invoke it:
```kotlin val lambdaClient = LambdaClient.builder().region(Region.US_EAST_1).build() val request = InvokeRequest.builder() .functionName("ProcessImageFunction") .payload(SdkBytes.fromByteArray("<BYTE_ARRAY_OF_YOUR_IMAGE>")) .build() val response = lambdaClient.invoke(request) val result = response.payload().asUtf8String() ```
6. Push Notifications with AWS SNS
Engage your users with push notifications using AWS SNS.
Example:
```kotlin val snsClient = SnsClient.builder().region(Region.US_EAST_1).build() val publishRequest = PublishRequest.builder() .topicArn("<YOUR_SNS_TOPIC_ARN>") .message("Hello from AWS SNS!") .build() snsClient.publish(publishRequest) ```
7. Monitoring with AWS CloudWatch
Ensure your app runs smoothly by monitoring its activities with AWS CloudWatch.
Example:
Log a custom metric (e.g., number of notes created):
```kotlin val cloudWatchClient = CloudWatchClient.builder().region(Region.US_EAST_1).build() val metricData = MetricDatum.builder() .metricName("NotesCreated") .value(1.0) .build() val putMetricDataRequest = PutMetricDataRequest.builder() .namespace("NoteAppMetrics") .metricData(metricData) .build() cloudWatchClient.putMetricData(putMetricDataRequest) ```
Conclusion
Combining Kotlin and AWS opens up endless possibilities for Android app developers. With a range of AWS services at your fingertips, you can build feature-rich, scalable, and secure apps efficiently. Embrace the cloud and harness the power of AWS in your Kotlin apps for a brighter development future.
Table of Contents
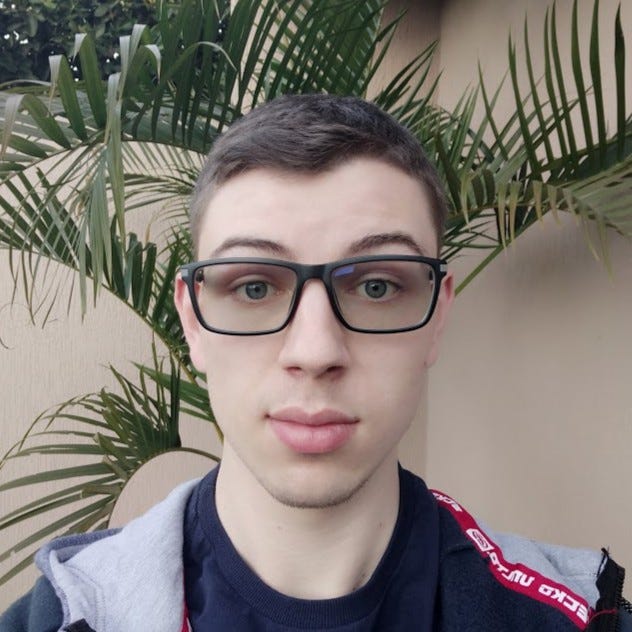
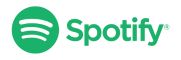