Kotlin and Azure Functions: Event-Driven Computing on Android
Event-driven computing has become an essential paradigm for building reactive and responsive applications. Azure Functions, Microsoft’s serverless compute service, pairs perfectly with Kotlin to enable efficient event-driven computing on Android. This article delves into how Kotlin and Azure Functions can be integrated to create event-driven applications, providing practical examples and use cases.
Understanding Event-Driven Computing
Event-driven computing is a programming paradigm where the flow of the program is determined by events such as user actions, sensor outputs, or message deliveries. This approach is particularly useful in scenarios where applications need to react immediately to changes or events.
Leveraging Kotlin for Event-Driven Development
Kotlin, with its expressive syntax and seamless interoperability with Java, is an ideal language for developing event-driven applications on Android. When combined with Azure Functions, Kotlin enables developers to offload event processing to the cloud, making applications more scalable and efficient.
Setting Up Azure Functions with Kotlin
Before diving into coding, you’ll need to set up Azure Functions and integrate them with your Kotlin project. Here’s a quick overview of the setup process:
- Create an Azure Function App
Log in to your Azure portal, navigate to Azure Functions, and create a new Function App. This app will host your event-driven functions.
- Install Azure Functions Extension for Visual Studio Code
If you’re using Visual Studio Code, install the Azure Functions extension to create and deploy functions easily.
- Add Kotlin Support
In your Kotlin Android project, add necessary dependencies to interact with Azure services using libraries like `Azure SDK for Java`.
Creating Event-Driven Functions in Kotlin
Azure Functions allow you to define code that runs in response to various triggers. Here’s how you can create a simple event-driven function in Kotlin.
Example: Responding to an HTTP Trigger
This example demonstrates how to create an Azure Function that responds to HTTP requests.
```kotlin import com.microsoft.azure.functions.* import com.microsoft.azure.functions.annotation.* class HttpTriggerFunction { @FunctionName("HttpTriggerFunction") fun run( @HttpTrigger( name = "req", methods = [HttpMethod.GET, HttpMethod.POST], authLevel = AuthorizationLevel.ANONYMOUS) request: HttpRequestMessage<String?>, context: ExecutionContext ): HttpResponseMessage { context.logger.info("HTTP trigger function executed.") val query = request.queryParameters["name"] val name = request.body.orEmpty() return request.createResponseBuilder(HttpStatus.OK) .body("Hello, ${query ?: name}!") .build() } } ```
This Kotlin function responds to HTTP GET and POST requests, allowing you to build interactive features in your Android application that leverage serverless functions.
Handling Real-Time Events with Azure Functions
Azure Functions can also handle real-time events such as messages in a queue, updates to a database, or changes to a blob storage. This enables your Android app to respond to cloud events seamlessly.
Example: Processing Queue Messages
Here’s an example of how you can create a function that processes messages from an Azure Storage Queue.
```kotlin import com.microsoft.azure.functions.* import com.microsoft.azure.functions.annotation.* class QueueTriggerFunction { @FunctionName("QueueTriggerFunction") fun run( @QueueTrigger( name = "message", queueName = "myqueue-items", connection = "AzureWebJobsStorage") message: String, context: ExecutionContext ) { context.logger.info("Queue trigger function processed a message: $message") } } ```
In this example, whenever a new message is added to the specified queue, the function is triggered, allowing your app to react to events like new orders or updates.
Integrating Azure Functions with Android
To leverage Azure Functions in your Android application, you can use HTTP requests to trigger the functions or connect to Azure services directly. Kotlin’s coroutines and async capabilities make it easy to handle these operations without blocking the main thread.
Example: Triggering an Azure Function from Android
```kotlin import kotlinx.coroutines.* import java.net.HttpURLConnection import java.net.URL fun triggerAzureFunction() { GlobalScope.launch(Dispatchers.IO) { val url = URL("https://<your-function-app>.azurewebsites.net/api/HttpTriggerFunction?name=Android") val connection = url.openConnection() as HttpURLConnection try { connection.requestMethod = "GET" connection.connect() if (connection.responseCode == HttpURLConnection.HTTP_OK) { val response = connection.inputStream.bufferedReader().readText() println("Function response: $response") } } finally { connection.disconnect() } } } ```
This example shows how to trigger an Azure Function from your Android app, enabling cloud-driven features like push notifications, event logging, and more.
Conclusion
Kotlin and Azure Functions together create a powerful duo for building event-driven applications on Android. By leveraging Kotlin’s language features and Azure’s serverless capabilities, developers can build responsive, scalable, and efficient mobile applications. This approach not only simplifies event processing but also enhances the overall user experience.
Further Reading:
Table of Contents
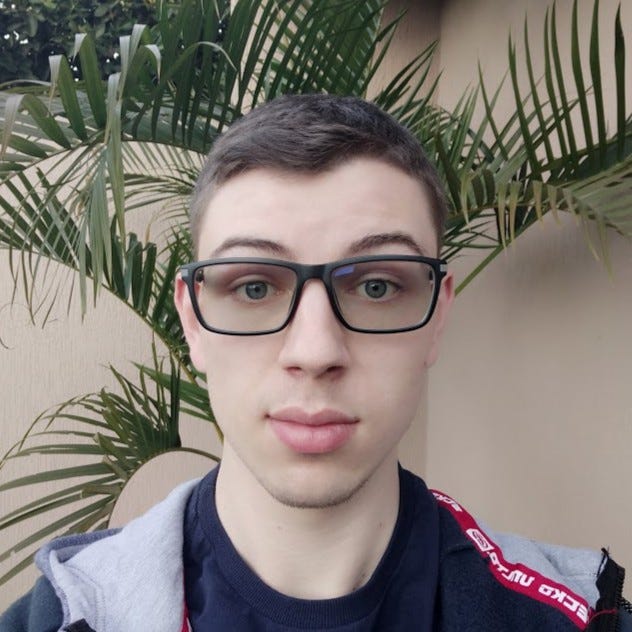
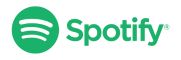