Step into Game Creation: Kotlin’s Guide to Iconic Games
Kotlin, renowned for its conciseness and versatility, is not only confined to Android development. It’s also an excellent choice for game development! In this post, we’ll walk you through the process of creating two classic games in Kotlin: Pong and Flappy Bird.
1. Pong
Pong is one of the earliest arcade video games, simulating table tennis. Each player controls a paddle by dragging it vertically across the screen’s left or right side. Players use their paddles to strike the ball back and forth.
Setup:
– Initialize a window: Use a library like [LibGDX](https://libgdx.badlogicgames.com/) to create your game window.
– Draw paddles and a ball on the screen.
Pseudocode:
```kotlin class PongGame : Game() { val paddleWidth = 15 val paddleHeight = 60 val ballSize = 15 var ballX = screenWidth / 2 var ballY = screenHeight / 2 var ballDirectionX = 1 var ballDirectionY = 1 var leftPaddleY = screenHeight / 2 var rightPaddleY = screenHeight / 2 override fun render() { // Handle paddle movement, ball movement, and collision detection here } } ```
Ball Movement:
The ball should move in a direction until it hits a wall or paddle, upon which it bounces.
Pseudocode:
```kotlin ballX += ballDirectionX * ballSpeed ballY += ballDirectionY * ballSpeed if (ballY <= 0 || ballY >= screenHeight) ballDirectionY *= -1 ```
Paddle Movement:
The paddles can move up and down based on user input.
Collision Detection:
Detect if the ball collides with a paddle and change its direction if it does.
Pseudocode:
```kotlin if (ballX <= paddleWidth && ballY + ballSize >= leftPaddleY && ballY <= leftPaddleY + paddleHeight || ballX + ballSize >= screenWidth - paddleWidth && ballY + ballSize >= rightPaddleY && ballY <= rightPaddleY + paddleHeight) { ballDirectionX *= -1 } ```
2. Flappy Bird
Flappy Bird is a side-scroller where the player controls a bird, attempting to fly between rows of green pipes without hitting them.
Setup:
– Initialize a window.
– Draw a bird and pipes on the screen.
Pseudocode:
```kotlin class FlappyBirdGame : Game() { val birdX = 50 var birdY = screenHeight / 2 var birdVelocity = 0 val gravity = -1 val jumpStrength = 15 var pipes = mutableListOf<Pipe>() override fun render() { // Handle bird movement, pipe movement, and collision detection here } } ```
Bird Movement:
The bird will continuously fall due to gravity, but a tap or click will make it “jump” or move upwards.
Pseudocode:
```kotlin birdVelocity += gravity birdY += birdVelocity if (userClickedOrTapped) birdVelocity = jumpStrength ```
Pipe Movement:
Pipes move from the right side of the screen to the left, and when they leave the screen, they are removed.
Pseudocode:
```kotlin for (pipe in pipes) { pipe.x -= pipeSpeed } pipes.removeIf { pipe -> pipe.x + pipeWidth < 0 } ```
Collision Detection:
Detect if the bird collides with a pipe or the ground.
Pseudocode:
```kotlin for (pipe in pipes) { if (birdX + birdSize > pipe.x && birdX < pipe.x + pipeWidth && (birdY + birdSize > pipe.topY || birdY < pipe.bottomY)) { gameOver() } } if (birdY <= 0) gameOver() ```
Conclusion
Kotlin’s expressiveness makes it an appealing choice for game development. The pseudocode above provides a skeletal idea of how one might implement these classic games. To get these games running, you’ll need to integrate a Kotlin-compatible game development framework like LibGDX, handle graphics rendering, and include more detailed game mechanics and physics.
Learning to develop games in Kotlin is a fun way to strengthen your grasp of the language and develop a deeper appreciation for game mechanics. As you progress, you can explore more advanced games and frameworks, or even try developing multiplayer games! The sky’s the limit.
Table of Contents
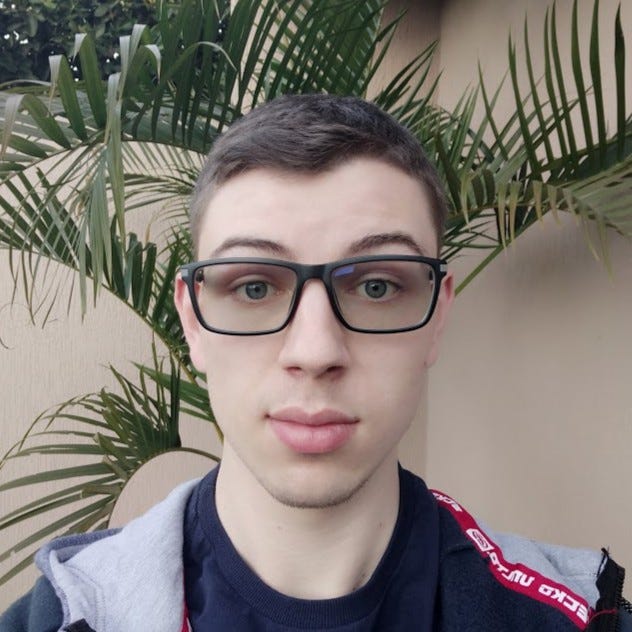
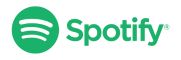