What is a companion object in Kotlin?
A companion object in Kotlin is a unique feature that allows you to define methods and properties associated with a class itself, rather than with instances of the class. It serves as a singleton instance associated with the class and provides a convenient way to encapsulate class-level functionality.
Unlike static members in other languages, companion objects are real objects and can implement interfaces, extend classes, or be passed as parameters. They offer a powerful mechanism for organizing and encapsulating class-level functionality in Kotlin.
One of the primary use cases for companion objects is to define factory methods for class instantiation. Since Kotlin does not have static methods, companion objects provide an elegant solution for creating instances of a class without the need for an accompanying instance.
For example:
kotlin class Logger private constructor() { companion object { fun create(): Logger { return Logger() } } }
In this example, the Logger class defines a private constructor to prevent direct instantiation. Instead, it provides a factory method create() within the companion object, allowing for controlled instantiation of Logger instances.
Companion objects also serve as containers for constants, utility methods, or shared resources associated with the class. Since companion objects are associated with the class itself, they offer a centralized location for accessing class-level functionality without the need for instance-specific references.
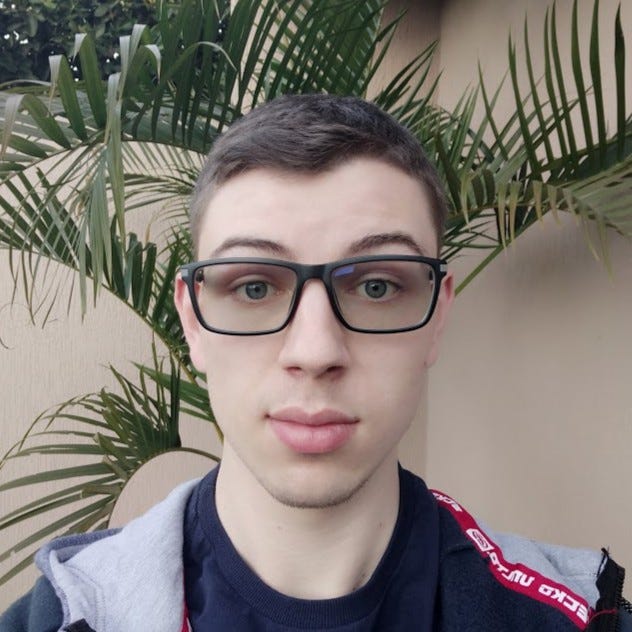
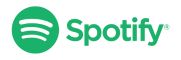