Kotlin Concurrency: Building Efficient and Scalable Apps
In today’s fast-paced world, building apps that can handle multiple tasks concurrently is essential for providing a seamless user experience. Kotlin, a modern and expressive programming language, offers robust concurrency support, allowing developers to create efficient and scalable applications that can handle multiple tasks at the same time. In this blog, we will explore Kotlin’s concurrency features, various techniques to utilize them effectively, and best practices to build apps that can harness the full power of concurrent programming.
1. Introduction to Kotlin Concurrency
Before delving into the specifics, let’s understand the concept of concurrency and why it matters for app development. Concurrency is the ability of a program to execute multiple tasks concurrently, rather than sequentially. By leveraging concurrency, we can improve the performance of our applications, as tasks can be executed in parallel, making better use of the available resources.
Kotlin, as a language designed to address the challenges of modern app development, provides powerful concurrency primitives and abstractions. Developers can make use of coroutines, channels, and other features to build responsive and efficient applications.
2. Understanding Coroutines
2.1 What are Coroutines?
Coroutines are at the heart of Kotlin’s concurrency model. They are lightweight and offer a way to perform asynchronous operations without blocking threads. Coroutines make it easy to write asynchronous code in a sequential style, making the code more readable and maintainable.
2.2 Creating Coroutines
To create a coroutine, we use the launch function from the kotlinx.coroutines library. Here’s a simple example:
kotlin import kotlinx.coroutines.* fun main() { println("Main thread: ${Thread.currentThread().name}") GlobalScope.launch { println("Coroutine: ${Thread.currentThread().name}") delay(1000) println("Coroutine after delay: ${Thread.currentThread().name}") } Thread.sleep(2000) }
In this example, we launch a coroutine using GlobalScope.launch, and it prints messages with thread names before and after a delay. The coroutine’s execution is asynchronous, so it won’t block the main thread.
2.3 Suspending Functions
Suspending functions are a fundamental concept in coroutines. They can be paused and resumed, allowing for easy handling of long-running tasks. Here’s an example of a suspending function:
kotlin suspend fun doTask() { delay(1000) println("Task completed!") }
2.4 Coroutine Scope
Coroutines need to run within a specific scope. Using coroutineScope or supervisorScope, we can define a scope for coroutines to ensure structured concurrency.
kotlin suspend fun main() = coroutineScope { launch { // Coroutine 1 } launch { // Coroutine 2 } }
3. Working with Channels
Channels are another powerful feature of Kotlin’s concurrency toolkit, enabling communication between coroutines. They allow safe data transfer between different coroutines.
3.1 Creating a Channel
To create a channel, we use the Channel factory function from the kotlinx.coroutines.channels package:
kotlin import kotlinx.coroutines.* import kotlinx.coroutines.channels.* fun main() = runBlocking { val channel = Channel<Int>() launch { for (i in 1..5) { channel.send(i) delay(200) } channel.close() } for (item in channel) { println(item) } }
In this example, a channel is created, and a coroutine is launched to send values to the channel. Another coroutine reads from the channel and prints the received values.
3.2 Buffering in Channels
Channels can be buffered to hold a limited number of elements. When the buffer is full, the sender coroutine is suspended until there is space available. Buffering can be useful for optimizing performance in certain scenarios.
kotlin val channel = Channel<Int>(capacity = 10)
3.3 Rendezvous Channels
Rendezvous channels have a buffer size of zero, making the sender and receiver coroutine synchronize with each other. The sender suspends until the receiver is ready to receive the data.
kotlin val channel = Channel<Int>(capacity = 0)
4. Thread Handling in Coroutines
Kotlin coroutines can be run on various dispatchers, which determine the thread or thread pool on which the coroutine runs. Understanding thread handling is essential for building efficient and scalable apps.
4.1 Default Dispatcher
By default, coroutines run on the Dispatchers.Default dispatcher, which uses a shared pool of threads optimized for CPU-intensive work.
kotlin fun main() = runBlocking { launch(Dispatchers.Default) { // Coroutine running on the Default dispatcher } }
4.2 Main Dispatcher
For UI-related tasks, coroutines can be launched on the Dispatchers.Main dispatcher, which runs on the main thread. This is particularly useful for Android app development.
kotlin import kotlinx.coroutines.* fun main() = runBlocking { launch(Dispatchers.Main) { // Coroutine running on the Main dispatcher } }
4.3 Custom Dispatcher
Developers can also define custom dispatchers tailored to specific requirements.
kotlin val customDispatcher = Executors.newFixedThreadPool(4).asCoroutineDispatcher() fun main() = runBlocking { launch(customDispatcher) { // Coroutine running on a custom dispatcher } }
5. Concurrency Patterns
Certain concurrency patterns can be applied to tackle common challenges faced during app development.
5.1 Producer-Consumer Pattern
The producer-consumer pattern involves one coroutine producing data, which is then consumed by another coroutine.
kotlin val channel = Channel<Int>() fun producer() = GlobalScope.launch { for (i in 1..5) { channel.send(i) delay(200) } channel.close() } fun consumer() = GlobalScope.launch { for (item in channel) { println(item) } } fun main() = runBlocking { producer() consumer().join() }
In this example, the producer coroutine produces values and sends them to the channel. The consumer coroutine reads from the channel and prints the received values.
5.2 MapReduce Pattern
The map-reduce pattern is useful for parallelizing data processing tasks. Coroutines can be employed to process data in parallel and then combine the results.
kotlin import kotlinx.coroutines.* fun processData(input: List<Int>): List<Int> = runBlocking { val chunks = input.chunked(100) // Split data into chunks val deferredResults = chunks.map { chunk -> async(Dispatchers.Default) { // Process each chunk in parallel chunk.map { /* processing logic */ } } } deferredResults.awaitAll().flatten() }
In this example, the processData function takes a large list of integers, splits it into smaller chunks, and processes each chunk concurrently using coroutines. The results are then combined and returned.
6. Best Practices for Kotlin Concurrency
To build efficient and scalable apps, keep the following best practices in mind:
6.1 Avoid Blocking Calls
Use suspending functions and coroutines instead of blocking calls to keep your app responsive.
6.2 Structured Concurrency
Always use structured concurrency by launching coroutines in a scope. This ensures proper handling and cleanup of coroutines.
6.3 Fine-tune Thread Pools
Adjust the number of threads in a thread pool based on the specific requirements of your application to achieve optimal performance.
6.4 Use Channels for Communication
Use channels for safe communication between coroutines to avoid data races and other synchronization issues.
6.5 Testing Concurrency
Test your concurrent code thoroughly to identify potential issues early in the development process.
Conclusion
Kotlin Concurrency opens up a world of possibilities for building efficient and scalable apps. With its powerful coroutines, channels, and other concurrency features, developers can create applications that perform exceptionally well, making the most of modern hardware. Understanding concurrency patterns and adhering to best practices will enable you to harness the full potential of Kotlin’s concurrency model and elevate your app development to new heights. Happy coding!
Table of Contents
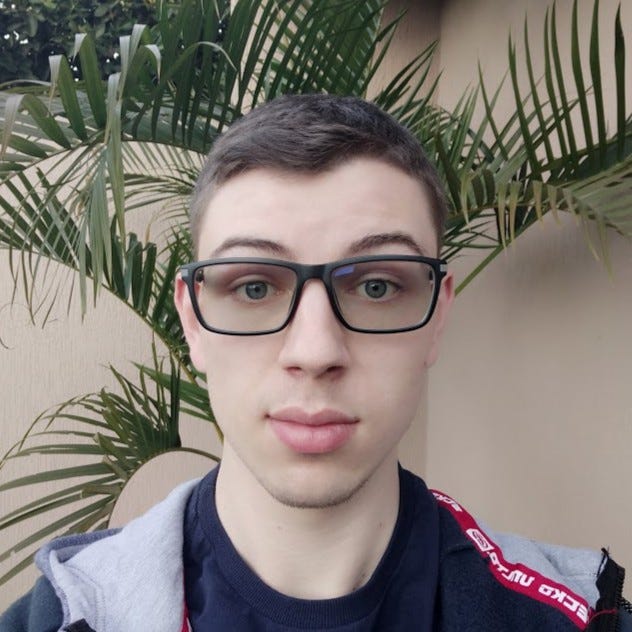
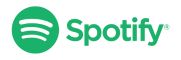