How do you create arrays in Kotlin?
In Kotlin, you can create arrays using various methods, depending on your requirements and the type of elements you want to store in the array. Kotlin provides convenient factory functions and array constructors for creating arrays of primitive types and objects.
Using arrayOf(): The arrayOf() function is used to create arrays of non-primitive types (objects). For example:
kotlin val names = arrayOf("John", "Alice", "Bob")
This creates an array of strings containing the specified elements.
Using intArrayOf(), doubleArrayOf(), etc.: Kotlin provides specialized functions for creating arrays of primitive types. For instance:
kotlin val numbers = intArrayOf(1, 2, 3, 4, 5)
This creates an array of integers.
Using Array constructor: You can also create arrays using the Array constructor, specifying the size and an initializer function to initialize the elements of the array. For example:
kotlin val squares = Array(5) { i -> i * i }
This creates an array of 5 elements containing the squares of their indices.
Arrays in Kotlin are zero-indexed, meaning the index of the first element is 0, the second element is at index 1, and so on. Kotlin arrays are mutable by default, allowing you to modify the elements after array creation if needed.
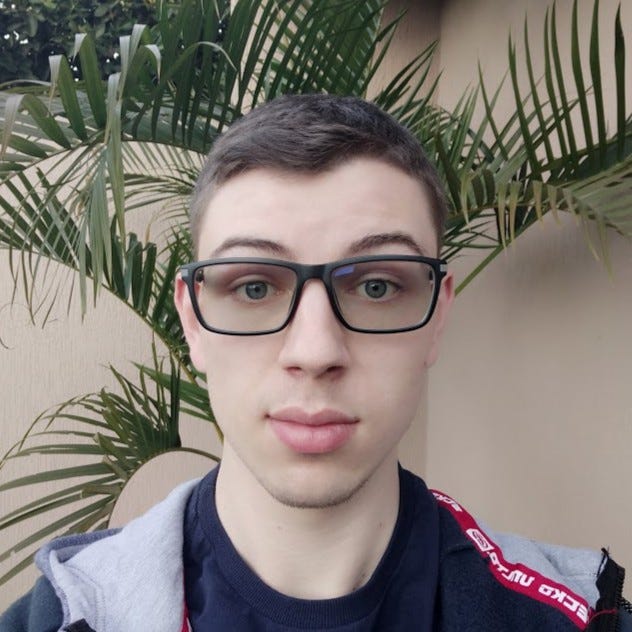
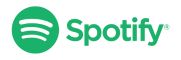