From Features to Functions: A Deep Dive into Kotlin-Cucumber BDD
Behavior-Driven Development (BDD) is an approach that centers around the behavior of an application rather than just its technical aspects. Cucumber is one of the most popular frameworks for BDD. When combined with Kotlin, a modern and expressive programming language, the duo can help developers achieve a clean and efficient BDD implementation.
1. What is Behavior-Driven Development?
BDD is an approach to software development that focuses on describing the behavior of the application from the perspective of its stakeholders. This is often done in simple, plain English (or other languages) sentences, which can be understood by non-technical team members as well. The main goal of BDD is to bridge the gap between developers, testers, and business stakeholders.
2. Why Cucumber?
Cucumber is an open-source tool used to support BDD. It allows you to write application behavior specifications in a natural language style, which is then translated into executable code. Cucumber supports various languages, including Java, Ruby, and of course, Kotlin.
3. Combining Kotlin with Cucumber
Kotlin is a modern, concise, and expressive language that runs on the Java Virtual Machine (JVM). Because of its compatibility with Java, it’s a natural fit for Cucumber, which has a mature ecosystem in the JVM space. Here are some reasons why Kotlin and Cucumber are a powerful combination:
- Expressiveness of Kotlin: Kotlin’s concise syntax means that test code can be much shorter and more readable.
- Safety: Kotlin’s type system can prevent many common errors in test code.
- Interoperability: Kotlin can use any Java libraries or frameworks, including Cucumber, without any issues.
4. Setting up a Cucumber Project with Kotlin
Let’s walk through the steps of setting up a Cucumber project using Kotlin.
4.1. Project Setup:
Start by creating a new Gradle project with Kotlin support. Add the necessary dependencies to your `build.gradle.kts` file:
```kotlin implementation("io.cucumber:cucumber-kotlin:7.1.0") implementation("io.cucumber:cucumber-junit:7.1.0") ```
4.2. Writing a Feature File:
Cucumber uses `.feature` files to describe the behavior of an application. Create a file named `calculator.feature` with the following content:
```gherkin Feature: Basic Calculator Scenario: Adding two numbers Given I have a calculator When I add 5 and 7 Then the result should be 12 ```
4.3. Implementing Step Definitions in Kotlin:
Now, let’s write the Kotlin code to turn these steps into executable actions. Create a Kotlin class named `StepDefinitions.kt`:
```kotlin import io.cucumber.java8.En import kotlin.test.assertEquals class CalculatorSteps : En { private var calculator = Calculator() private var result: Int = 0 init { Given("I have a calculator") { // No action needed, as we already initialized the calculator } When("I add {int} and {int}") { a: Int, b: Int -> result = calculator.add(a, b) } Then("the result should be {int}") { expected: Int -> assertEquals(expected, result) } } } ```
Of course, you’d also need the `Calculator` class. For simplicity, let’s assume it has an `add` method that takes two integers and returns their sum.
4.4. Running the Test:
With the above setup, you can now run your feature as a JUnit test. Remember to configure the test runner to use Cucumber’s JUnit platform.
Conclusion
Behavior-Driven Development is a powerful methodology that can ensure everyone in a development team is on the same page regarding an application’s behavior. Kotlin and Cucumber, when combined, make the process of adopting BDD smoother and more efficient. Kotlin brings the power of a modern programming language, while Cucumber provides a mature framework to structure BDD scenarios. The combination allows developers to write clearer, more maintainable, and more efficient BDD scenarios.
Table of Contents
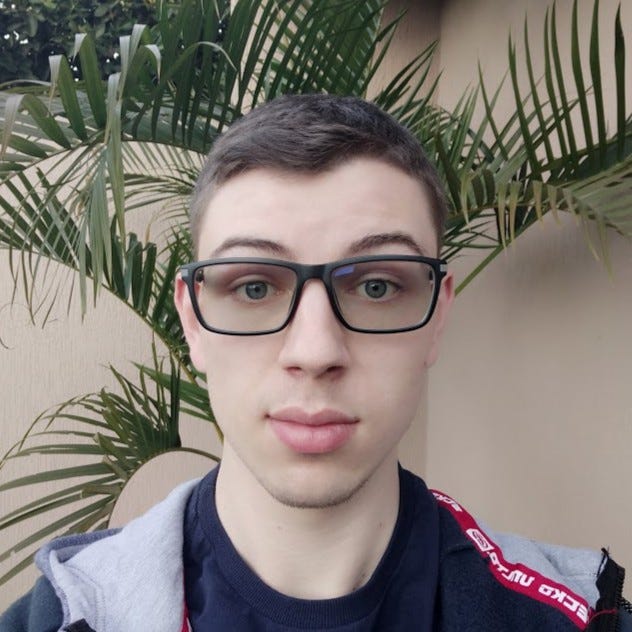
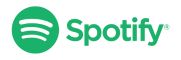