The Ultimate Guide to Simplifying Dependency Injection: Kotlin & Dagger Hilt
When building modern Android applications, dependency injection is a common pattern to ensure a clear separation of concerns and efficient management of resources. Dagger, one of the most popular DI libraries in the Android ecosystem, has evolved significantly over the years. Enter Dagger Hilt, an extension to Dagger that simplifies its use. And when combined with Kotlin, it becomes a potent combination.
In this article, we’ll explore Dagger Hilt, how it simplifies dependency injection, and provide practical examples of how to use it in your Kotlin-based Android applications.
1. What is Dependency Injection?
Dependency Injection (DI) is a design pattern where an object’s dependencies are injected into it, rather than the object creating its dependencies. DI decouples the creation and usage of objects, making the code more modular and testable.
Consider a simple example without DI:
```kotlin class Engine { /*...*/ } class Car { private val engine = Engine() } ```
With DI:
```kotlin class Engine { /*...*/ } class Car(private val engine: Engine) { /*...*/ } ```
2. Why Dagger Hilt?
While Dagger is a powerful DI library, it comes with a steep learning curve. Dagger Hilt builds on Dagger to simplify the DI setup process, make it more intuitive, and reduce boilerplate.
Hilt provides a standardized way to incorporate Dagger dependency injection into Android applications. By leveraging annotations and predefined components, Hilt takes away much of the complexity.
3. Setting up Dagger Hilt
To start with Hilt, add the required dependencies to your `build.gradle` file:
```kotlin implementation "com.google.dagger:hilt-android:2.x.x" kapt "com.google.dagger:hilt-compiler:2.x.x" ```
Next, initialize Hilt in your application:
```kotlin @HiltAndroidApp class MyApplication : Application() { /*...*/ } ```
4. Injecting Dependencies with Dagger Hilt
- Field Injection:
Hilt can inject dependencies directly into Android classes’ fields that support injection:
```kotlin class MainActivity : AppCompatActivity() { @Inject lateinit var engine: Engine override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // Now you can use `engine` } } ```
5. Constructor Injection:
For other classes (like your data repositories, use cases, etc.), Hilt can provide dependencies through the constructor:
```kotlin class CarRepository @Inject constructor(private val engine: Engine) { // Use the `engine` here } ```
6. Providing Dependencies
To let Hilt know how to provide instances of dependencies, you use the `@Module` and `@InstallIn` annotations:
```kotlin @Module @InstallIn(SingletonComponent::class) object AppModule { @Provides @Singleton fun provideEngine(): Engine { return Engine() } } ```
7. Component Scopes
Dagger Hilt uses component scopes to dictate the lifespan of provided instances:
– @Singleton: For objects that should live as long as the application.
– @ActivityRetainedScoped: For objects that should live as long as an activity, through configuration changes.
– @ActivityScoped: For objects that should live as long as the current activity instance.
– @FragmentScoped: For objects that should live as long as the fragment instance.
– @ViewScoped: For objects that should live as long as the view.
– @ViewModelScoped: For objects that should live as long as the ViewModel.
8. Using ViewModel with Dagger Hilt
Dagger Hilt integrates seamlessly with Android’s ViewModel:
```kotlin @HiltViewModel class MyViewModel @Inject constructor(private val repository: CarRepository) : ViewModel() { // Use `repository` here } ```
In your Android classes, you can then retrieve the ViewModel as usual:
```kotlin val viewModel by viewModels<MyViewModel>() ```
9. Benefits of Using Dagger Hilt with Kotlin
– Simplified Syntax: Kotlin’s concise syntax combined with Dagger Hilt’s annotations means cleaner code.
– Type Safety: Kotlin’s type system and Dagger Hilt ensure your dependencies are type-safe.
– Null Safety: Kotlin’s built-in null safety features combined with Dagger Hilt ensure that you won’t run into unexpected null issues.
– Reduced Boilerplate: With Kotlin extension functions and Dagger Hilt’s simplified setup, the amount of boilerplate code is reduced.
Conclusion
Dependency Injection is a powerful design pattern, and Dagger Hilt simplifies its implementation in Android applications. When combined with the elegance and power of Kotlin, developers can create clean, modular, and robust applications with ease. If you haven’t given Dagger Hilt a shot yet, it might be time to dive in and enhance your Android development experience.
Table of Contents
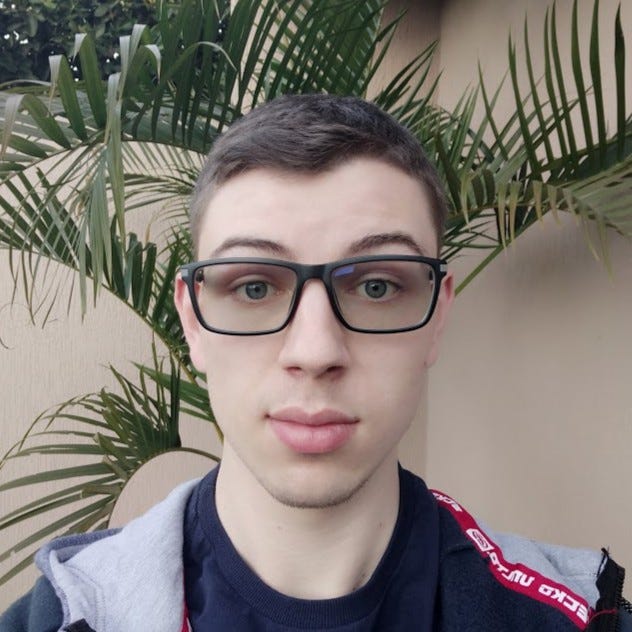
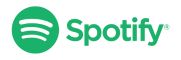