Kotlin’s Leap into Data Science: An In-depth Guide to Analysis & Visualization
Kotlin, initially heralded as the modernized successor to Java for Android development, has increasingly shown its prowess in various domains beyond mobile applications. One such domain where Kotlin is making significant strides is data science. With its concise syntax, interoperability with Java libraries, and ever-growing community, Kotlin is a budding contender for data analysis and visualization tasks. This blog post delves deep into the world of data science through Kotlin’s lens, highlighting examples and showcasing its potential.
1. Why Kotlin for Data Science?
- Concise Syntax: Kotlin’s expressive syntax allows for clear and concise code, reducing boilerplate and increasing readability.
- Java Interoperability: Kotlin’s seamless integration with Java provides access to a myriad of Java libraries, including those pertinent to data science.
- Safety: Kotlin’s inherent null-safety and robust type system prevent common pitfalls and errors.
- Growing Ecosystem: With libraries such as Kotlin for Apache Spark (Krangl) and Kotlin for scientific computing (KMath), Kotlin’s data science ecosystem is rapidly growing.
2. Data Analysis with Krangl
Krangl is an impressive data wrangling library, reminiscent of Python’s Pandas, but with Kotlin’s characteristic flair. Let’s consider an example:
Scenario: You’re working with a dataset containing sales data. The aim is to compute the total sales for each product.
```kotlin import krangl.* fun main() { val salesDataFrame = DataFrame.readCSV("sales.csv") salesDataFrame .groupBy("product") .summarize("total_sales" to { it["sales"].sum(removeNA = true) }) .print() } ```
This succinct piece of code reads a CSV file, groups it by the product column, and then summarizes to compute the total sales. With just a few lines, Kotlin and Krangl make data manipulation an effortless task.
3. Data Visualization with Let’s-Plot
Let’s-Plot is a visualization library for Kotlin, enabling a grammar of graphics similar to the famous ggplot2 in R.
Scenario: Given the above sales data, you wish to visualize the total sales per product.
```kotlin import lets_plot.* import lets_plot.plotting.ggplot import lets_plot.plotting.geom_bar fun main() { val salesData = mapOf( "product" to listOf("A", "B", "C", "A", "B", "A"), "sales" to listOf(10, 20, 30, 15, 25, 35) ) val p = ggplot(salesData) + geom_bar(stat = Stat.identity, aes(x = "product", y = "sales")) p.show() } ```
This code creates a bar plot showcasing sales for each product, allowing for easy comparisons and insights.
4. Deep Learning with KotlinDL
While data wrangling and visualization are key, another important facet of data science is machine learning. KotlinDL provides Kotlin-centric deep learning functionalities.
Scenario: You aim to train a neural network on the famous MNIST dataset to recognize handwritten digits.
```kotlin import org.jetbrains.kotlinx.dl.api.core.Sequential import org.jetbrains.kotlinx.dl.api.core.layer.core.Dense import org.jetbrains.kotlinx.dl.datasets.datasetOn import org.jetbrains.kotlinx.dl.datasets.mnist fun main() { val (train, test) = mnist() val model = Sequential.of( Dense(256, activation = Activations.Relu, inputShape = 784), Dense(128, activation = Activations.Relu), Dense(10, activation = Activations.Softmax) ) model.compile(optimizer = Adam(), loss = Losses.SOFT_MAX_CROSS_ENTROPY_WITH_LOGITS) model.fit(dataset = datasetOn(train), epochs = 10, batchSize = 128) val accuracy = model.evaluate(dataset = datasetOn(test)).metrics["accuracy"] println("Accuracy: $accuracy") } ```
This example demonstrates training a simple feed-forward neural network. The results, post-training, can be evaluated against the test set to get an accuracy metric.
Conclusion
Kotlin, while still nascent in the data science domain compared to Python or R, is making promising advancements. The examples showcased underscore the versatility of Kotlin in handling data tasks, from wrangling and visualization to deep learning. As the ecosystem matures, adopting Kotlin for data science tasks may become more prevalent, combining the power of Kotlin’s language features with robust tools and libraries. Whether you’re a seasoned data scientist or a Kotlin enthusiast, there’s an exciting confluence of Kotlin and data science waiting to be explored!
Table of Contents
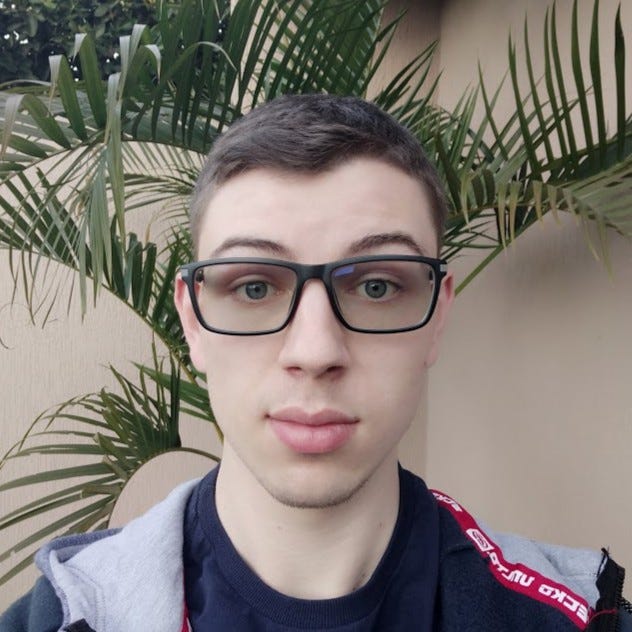
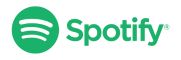