How do you define an abstract class in Kotlin?
To define an abstract class in Kotlin, you use the abstract keyword before the class declaration. Additionally, you can include abstract methods (methods without a body) within the abstract class, which must be implemented by subclasses.
For example:
kotlin abstract class Shape { abstract fun area(): Double abstract fun perimeter(): Double fun description() { println("This is a shape") } }
In this example, the Shape class is declared as abstract using the abstract keyword. It defines abstract methods area() and perimeter(), which must be implemented by subclasses. The description() method provides a concrete implementation and can be inherited by subclasses.
Abstract classes provide a way to define common behavior and structure for related classes while allowing subclasses to provide specific implementations for abstract methods. They serve as a key tool for promoting code reuse and abstraction in Kotlin applications.
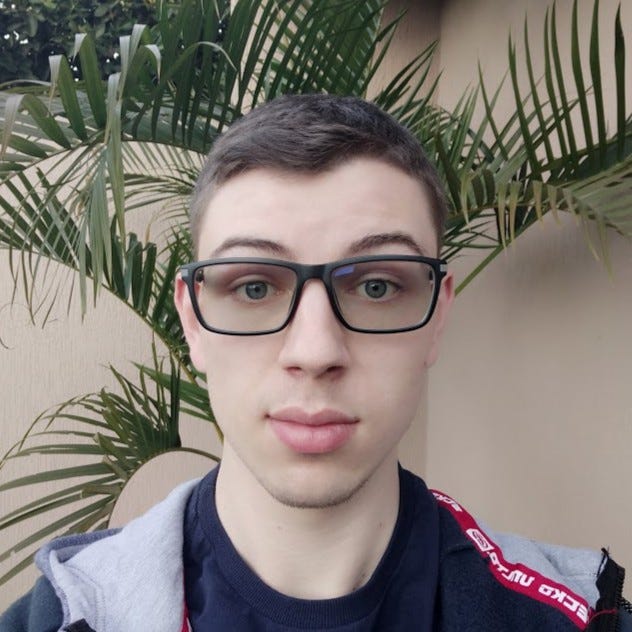
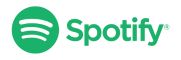