How do you define a companion object in Kotlin?
Defining a companion object in Kotlin is straightforward and intuitive. To create a companion object, you use the companion keyword followed by the object definition within the class body. The companion object contains methods, properties, or other declarations associated with the class itself rather than with instances of the class.
Here’s an example demonstrating the definition of a companion object within a class:
kotlin class MyClass { companion object { fun sayHello() { println("Hello from companion object!") } } }
In this example, the MyClass class contains a companion object with a single method sayHello(). This method is associated with the class itself and can be accessed using the class name, rather than through an instance of the class.
Companion objects offer a powerful mechanism for encapsulating class-level functionality and providing factory methods, constants, or utility functions associated with the class. They promote code organization, readability, and maintainability by centralizing class-level declarations within the scope of the class definition.
Companion objects in Kotlin provide a versatile and concise way to define class-level functionality and encapsulate shared resources. Their integration into the Kotlin language facilitates the development of modular and maintainable codebases, enhancing code organization and promoting best practices in object-oriented programming.
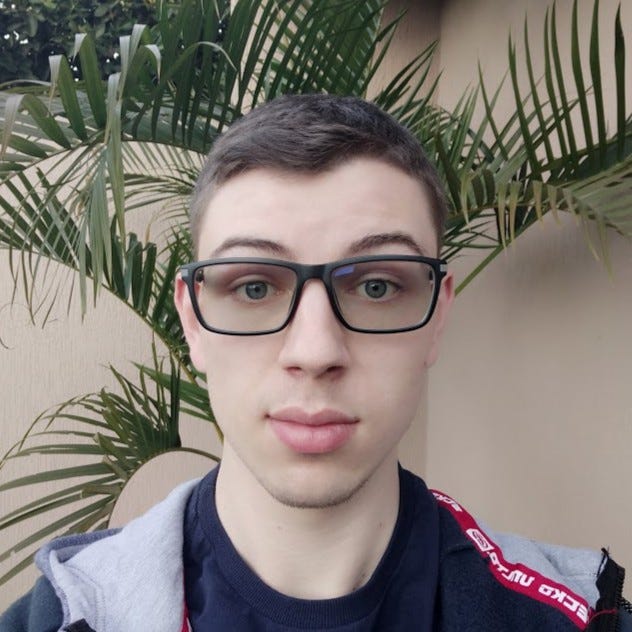
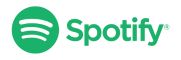