How do you define a constructor in Kotlin?
To define a primary constructor in Kotlin, you declare the constructor parameters directly after the class name:
kotlin class Person(val name: String, val age: Int) { // Class body }
In this example, name and age are parameters of the primary constructor, and they also double as properties of the Person class.
Alternatively, Kotlin also allows for the declaration of secondary constructors using the constructor keyword:
kotlin class Person { constructor(name: String, age: Int) { // Constructor body } }
Secondary constructors provide additional flexibility when initializing objects, allowing for custom initialization logic beyond what the primary constructor provides.
Kotlin classes can indeed have constructors, and they play a crucial role in initializing class instances and setting up object states. The flexibility provided by Kotlin’s constructor syntax contributes to the language’s expressiveness and ease of use in object-oriented programming scenarios.
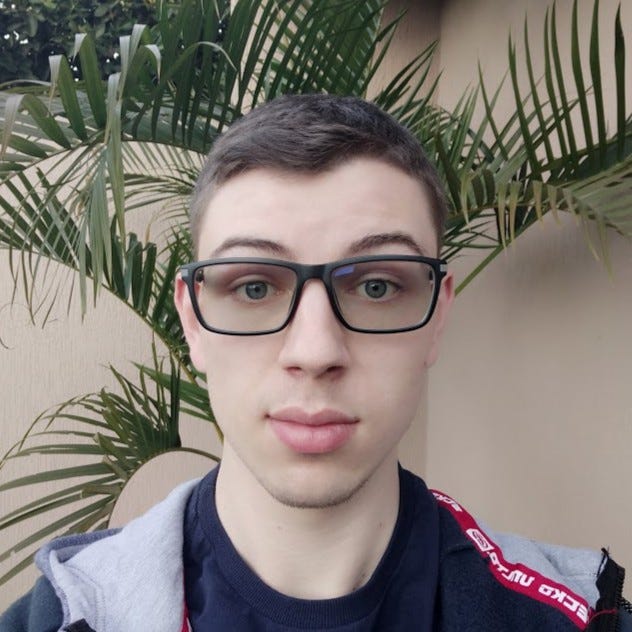
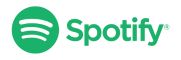