Kotlin and Elasticsearch: Searching and Analyzing Data
In today’s data-driven world, efficiently searching and analyzing large datasets is crucial. Elasticsearch, a powerful open-source search engine, is widely used for its full-text search capabilities and real-time data analytics. Kotlin, a modern programming language known for its concise syntax and interoperability with Java, can be effectively utilized to work with Elasticsearch. This article explores how Kotlin can be used with Elasticsearch to perform efficient data searches and analyses, complete with practical examples.
Understanding Elasticsearch
Elasticsearch is a distributed search and analytics engine designed for handling large volumes of data. It provides real-time search capabilities, scalable performance, and extensive querying options, making it ideal for various data search and analysis tasks.
Using Kotlin with Elasticsearch
Kotlin’s modern features and seamless integration with Java make it a great choice for interacting with Elasticsearch. Below are key aspects and code examples demonstrating how Kotlin can be used to search and analyze data with Elasticsearch.
1. Setting Up Elasticsearch Client in Kotlin
To start working with Elasticsearch in Kotlin, you need to set up the Elasticsearch client. The `elasticsearch-rest-high-level-client` library is commonly used for this purpose.
Example: Configuring Elasticsearch Client
```kotlin import org.elasticsearch.client.RestClient import org.elasticsearch.client.RestHighLevelClient import org.elasticsearch.client.RestClientBuilder import org.elasticsearch.client.RestClientBuilder.HttpClientConfigCallback fun createClient(): RestHighLevelClient { val builder: RestClientBuilder = RestClient.builder( HttpHost("localhost", 9200, "http") ) return RestHighLevelClient(builder) } ```
2. Indexing Data into Elasticsearch
Before you can search and analyze data, you need to index it into Elasticsearch. Kotlin makes this task straightforward with the Elasticsearch client.
Example: Indexing a Document
```kotlin import org.elasticsearch.action.index.IndexRequest import org.elasticsearch.action.index.IndexResponse import org.elasticsearch.client.RequestOptions import org.elasticsearch.client.RestHighLevelClient import org.elasticsearch.action.index.IndexRequest import org.elasticsearch.action.index.IndexResponse fun indexDocument(client: RestHighLevelClient, index: String, id: String, jsonData: String) { val request = IndexRequest(index).id(id).source(jsonData, XContentType.JSON) val response: IndexResponse = client.index(request, RequestOptions.DEFAULT) println("Indexed document with ID: ${response.id}") } ```
3. Searching Data in Elasticsearch
Elasticsearch provides a powerful querying mechanism to search for data. You can use Kotlin to craft queries and retrieve relevant data.
Example: Searching for Documents
```kotlin import org.elasticsearch.action.search.SearchRequest import org.elasticsearch.action.search.SearchResponse import org.elasticsearch.client.RequestOptions import org.elasticsearch.client.RestHighLevelClient import org.elasticsearch.index.query.QueryBuilders fun searchDocuments(client: RestHighLevelClient, index: String, query: String) { val searchRequest = SearchRequest(index) searchRequest.source().query(QueryBuilders.matchQuery("field", query)) val searchResponse: SearchResponse = client.search(searchRequest, RequestOptions.DEFAULT) searchResponse.hits.forEach { hit -> println("Document ID: ${hit.id}, Source: ${hit.sourceAsString}") } } ```
4. Analyzing Data with Aggregations
Elasticsearch supports aggregations to perform complex data analyses. Kotlin can be used to build aggregation queries and interpret results.
Example: Aggregating Data
```kotlin import org.elasticsearch.action.search.SearchRequest import org.elasticsearch.action.search.SearchResponse import org.elasticsearch.client.RequestOptions import org.elasticsearch.client.RestHighLevelClient import org.elasticsearch.search.aggregations.AggregationBuilders import org.elasticsearch.search.aggregations.Aggregations import org.elasticsearch.search.aggregations.bucket.histogram.Histogram fun aggregateData(client: RestHighLevelClient, index: String) { val searchRequest = SearchRequest(index) searchRequest.source().aggregation( AggregationBuilders.histogram("date_histogram").field("date").calendarInterval(DateHistogramInterval.DAY) ) val searchResponse: SearchResponse = client.search(searchRequest, RequestOptions.DEFAULT) val histogram = searchResponse.aggregations.get<Histogram>("date_histogram") histogram.buckets.forEach { bucket -> println("Date: ${bucket.keyAsString}, Count: ${bucket.docCount}") } } ```
Conclusion
Kotlin provides a modern, expressive way to interact with Elasticsearch, enabling efficient data search and analysis. From setting up Elasticsearch clients to indexing data, performing searches, and running aggregations, Kotlin’s features complement Elasticsearch’s powerful capabilities. By leveraging these tools effectively, you can enhance your data management strategies and gain valuable insights from your data.
Further Reading:
Table of Contents
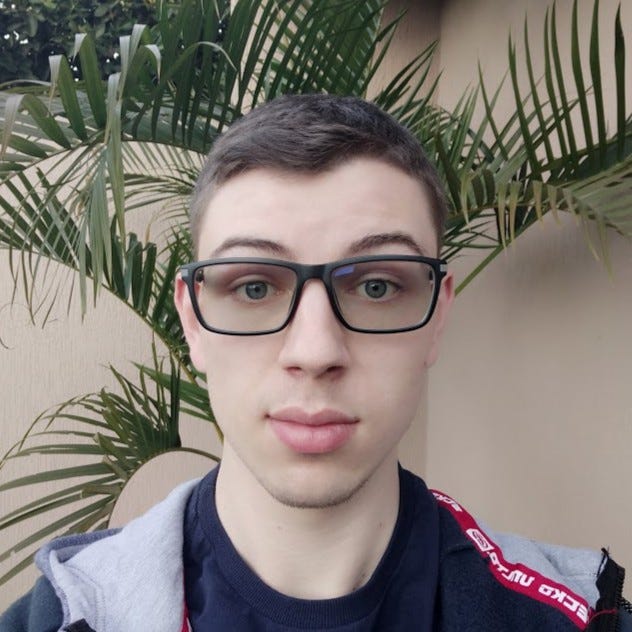
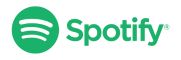