Empowering Kotlin Code with Extensions: A Practical Guide
When it comes to developing apps using Kotlin, one of the most powerful features at your disposal is extension functions and properties. Kotlin extensions allow you to add more functionality to existing classes, leading to more readable, expressive, and concise code. These abilities can be greatly beneficial when you want to hire Kotlin developers, as their familiarity with such tools can significantly boost productivity. In this article, we will dive deep into the world of Kotlin extensions and provide practical examples to enhance your coding skills and illustrate the proficiency that hiring Kotlin developers can bring to your project.
What Are Kotlin Extensions?
In Kotlin, an extension function is a function that, within its body, has access to the members of the class that it extends. This means that you can create new functions for a class from any library or even the Kotlin Standard Library, without inheriting from the class or using any kind of design pattern like Decorator.
Extension functions are resolved statically, which means they are called based on the declared type of the variable, not the actual type of the object. This behavior is different from usual polymorphism in object-oriented programming.
Syntax
The syntax of Kotlin extension functions is straightforward. You only need to prefix the name of the function with the class that you want to extend. Here’s a simple example:
```kotlin fun String.lastChar(): Char = this[this.length - 1] ```
This function adds a new `lastChar()` function to the String class. `this` refers to the object on which the function is called. Now, every String in your Kotlin code has a `lastChar()` function.
```kotlin val name = "Kotlin" println(name.lastChar()) // Prints "n" ```
Extending Kotlin Classes
Let’s see more examples of how we can use Kotlin extensions to make our code cleaner and more expressive.
1. List Extensions
A common task when working with lists is to get a particular range of elements. Without extensions, this can be a bit verbose:
```kotlin val list = listOf("Apple", "Banana", "Cherry", "Date", "Elderberry") val sublist = list.subList(1, 4) // Returns ["Banana", "Cherry", "Date"] ```
With an extension function, you can make this much more expressive:
```kotlin fun <T> List<T>.getRange(start: Int, end: Int): List<T> = this.subList(start, end) ```
Now you can write:
```kotlin val fruits = listOf("Apple", "Banana", "Cherry", "Date", "Elderberry") val selectedFruits = fruits.getRange(1, 4) // Returns ["Banana", "Cherry", "Date"] ```
This makes the code more readable and intuitive.
2. String Extensions
The String class has many useful functions, but there are always more that can be added. For example, if you often find yourself needing to count the number of vowels in a string, you could define an extension function to do this:
```kotlin fun String.countVowels(): Int { return this.count { it in "aeiouAEIOU" } } println("Hello, World!".countVowels()) // Prints 3 ```
3. Android View Extensions
If you are an Android developer, extension functions can simplify your life in many ways. One common task in Android development is hiding a view. Usually, you would do something like this:
```kotlin if (view.visibility == View.VISIBLE) { view.visibility = View.GONE } ```
With Kotlin extensions, you can create a function that encapsulates this behavior:
```kotlin fun View.hide() { if (visibility == View.VISIBLE) { visibility = View.GONE } } ``
`And use it like this:
```kotlin view.hide() ```
Extension Properties
In addition to functions, Kotlin also supports extension properties. They allow you to add properties to an existing class in a similar way as extension functions. Here is an example:
```kotlin val String.numVowels: Int get() = count { it in "aeiouAEIOU" } println("Hello, World!".numVowels) // Prints 3 ```
This defines a read-only property `numVowels` for the String class, using the same logic as the `countVowels()` function defined earlier.
Nullability and Extensions
Extensions can also be used with nullable types. If you define an extension for a nullable type, you can use it even if the object might be null. Here’s an example:
```kotlin fun String?.isNullOrEmptyOrBlank(): Boolean = this == null || this.isEmpty() || this.isBlank() val name: String? = null println(name.isNullOrEmptyOrBlank()) // Prints "true" ```
Limitations
While Kotlin extensions are powerful, there are some important limitations to keep in mind:
– Extensions do not actually modify the classes they extend. They are resolved statically, not dynamically, which means they do not support overriding.
– Extensions can’t be used to define new properties with backing fields. While you can define simple computed properties, these properties cannot maintain a state between different calls.
– Extensions can’t be used to define local functions. They need to be top-level functions or member functions in another class.
Conclusion
In this blog post, we’ve explored how Kotlin extensions can enhance your code by making it more readable, expressive, and concise. By adding new functionality to existing classes, you can create code that feels natural and intuitive to work with. Whether you’re looking to hire Kotlin developers or you’re a seasoned Kotlin developer or a beginner, leveraging the power of Kotlin extensions will certainly make your code better and your life easier.
Table of Contents
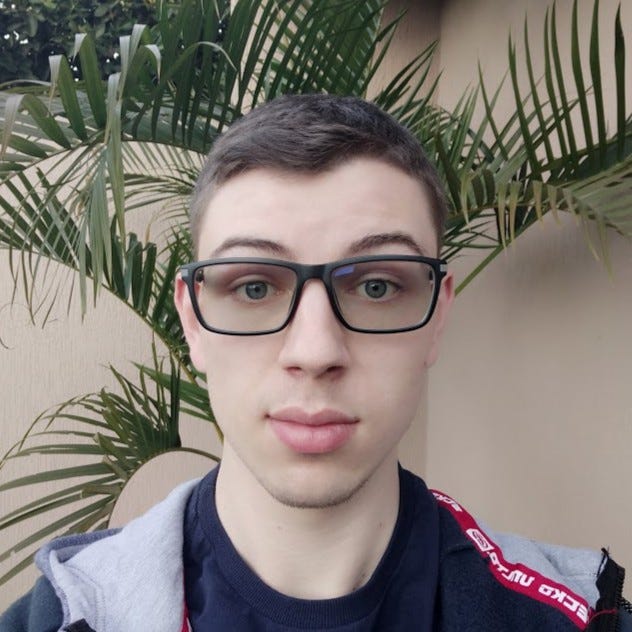
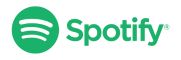