Kotlin and Firebase Cloud Functions: Extending Your App’s Functionality
Firebase Cloud Functions enable developers to run backend code in response to events triggered by Firebase features and HTTPS requests. Kotlin, with its expressive syntax and robust features, is a great choice for writing these functions. This article explores how to integrate Kotlin with Firebase Cloud Functions to add powerful, serverless features to your applications.
Understanding Firebase Cloud Functions
Firebase Cloud Functions is a serverless framework that allows you to execute backend code in response to events without managing your own servers. It supports various triggers, such as changes in your Firestore database, authentication events, or file uploads to Firebase Storage.
Setting Up Firebase Cloud Functions with Kotlin
To use Kotlin for Firebase Cloud Functions, you need to configure your environment and set up the necessary tools.
Prerequisites:
– Node.js installed on your machine
– Firebase CLI installed and configured
– Basic understanding of Kotlin and Firebase services
Initial Setup:
- Install Firebase CLI:
```bash npm install -g firebase-tools ```
- Initialize Firebase in your project:
```bash firebase init functions ```
- Add Kotlin to your project:
You can use the `kotlin` node module to transpile Kotlin to JavaScript.
Writing Your First Firebase Function in Kotlin
Let’s create a simple Firebase Cloud Function that triggers when a new document is added to Firestore.
Example: Firestore Trigger
```kotlin import firebase.functions.FirebaseFunction import firebase.firestore.FirestoreEvent class FirestoreTriggers : FirebaseFunction() { @CloudFunction fun onDocumentCreated(event: FirestoreEvent) { val newDocument = event.value // Access data from the new document val data = newDocument.getString("dataField") ?: "No data" println("New document created with data: $data") } } ```
Handling HTTP Requests
Firebase Cloud Functions can also respond to HTTPS requests, allowing you to create RESTful APIs. Here’s an example of handling an HTTP request in Kotlin.
Example: HTTP Trigger
```kotlin import firebase.functions.HttpFunction import firebase.functions.HttpRequest import firebase.functions.HttpResponse class HttpTriggers : HttpFunction() { @CloudFunction fun onRequest(request: HttpRequest, response: HttpResponse) { val name = request.query.get("name") ?: "World" response.send("Hello, $name!") } } ```
Integrating with Firebase Services
Firebase Cloud Functions can be used to enhance your app by integrating with various Firebase services, such as Firebase Authentication, Firestore, and Cloud Storage.
Example: Sending a Welcome Email with Firebase Authentication
When a new user signs up, you might want to send them a welcome email. Here’s how you can do it using Kotlin and Firebase Cloud Functions.
```kotlin import firebase.auth.AuthEvent import firebase.functions.FirebaseFunction class AuthTriggers : FirebaseFunction() { @CloudFunction fun onUserCreated(event: AuthEvent) { val email = event.email ?: return sendWelcomeEmail(email) } private fun sendWelcomeEmail(email: String) { // Code to send email println("Welcome email sent to: $email") } } ```
Deploying Your Functions
Deploying your Kotlin functions is straightforward using the Firebase CLI. Simply run:
```bash firebase deploy --only functions ```
This command will deploy your functions to Firebase, making them live and ready to handle events.
Conclusion
Kotlin and Firebase Cloud Functions provide a powerful combination for extending your app’s functionality. Whether you’re handling real-time events, processing HTTP requests, or integrating with other Firebase services, Kotlin makes it easier and more enjoyable to write cloud functions. By leveraging Firebase’s serverless architecture, you can scale your app effortlessly while focusing on writing clean and efficient code.
Further Reading:
Table of Contents
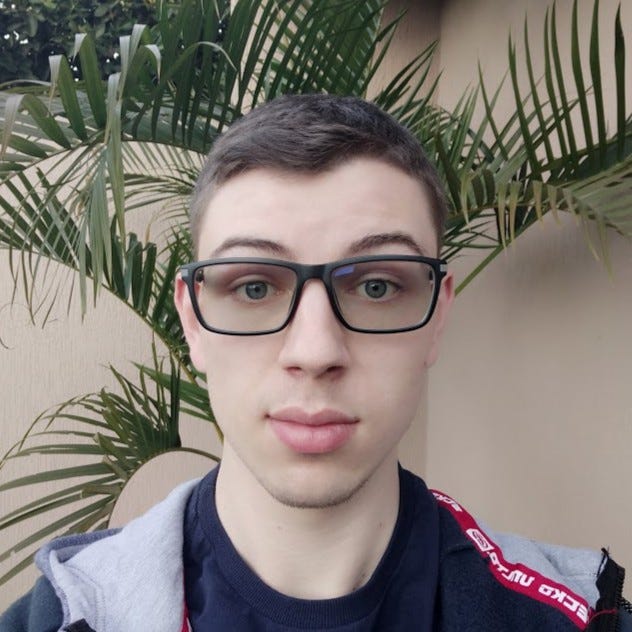
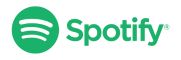