How to Supercharge Your Android App with Kotlin and Firebase Notifications
In today’s digital age, push notifications serve as a direct channel of communication between applications and users. It is essential for apps that require real-time information delivery, such as messaging apps, news apps, and many more. Firebase Cloud Messaging (FCM) is a robust solution for managing push notifications, and Kotlin’s concise syntax makes it easier than ever to integrate FCM in your Android apps.
In this article, we will explore how to set up Firebase Cloud Messaging with Kotlin to send and receive push notifications.
1. What is Firebase Cloud Messaging?
Firebase Cloud Messaging (FCM) is a free, cloud-based messaging service offered by Google that lets you send notifications to your users. It supports messaging on iOS, Android, and web applications.
FCM provides two types of messaging:
- Notification Messages: Predefined key-value pairs that automatically display messages when the app is in the background.
- Data Messages: Custom key-value pairs that allow the application to handle the message in the foreground.
2. Setting Up Firebase In Your Kotlin Project
2.1. Firebase Project Setup
– Go to the [Firebase Console](https://console.firebase.google.com/).
– Click on “Add Project” and follow the on-screen instructions.
– Once the project is created, select “Add Firebase to your Android app” and provide your app’s package name.
2.2. Add Firebase SDK
– Open the `build.gradle` file of your app and add the following dependency for FCM:
```kotlin implementation 'com.google.firebase:firebase-messaging:23.0.0' // make sure to check for the latest version ```
2.3. Initialize Firebase in your Kotlin App
– Ensure that your `Application` class initializes Firebase:
```kotlin class MyApp: Application() { override fun onCreate() { super.onCreate() FirebaseApp.initializeApp(this) } } ```
2.4. Add Internet Permission
– Add the following line to your `AndroidManifest.xml`:
```xml <uses-permission android:name="android.permission.INTERNET" /> ```
3. Handling FCM Push Notifications
3.1. Create a Service:
– To receive FCM messages, create a service that extends `FirebaseMessagingService`:
```kotlin class MyFirebaseMessagingService : FirebaseMessagingService() { override fun onMessageReceived(remoteMessage: RemoteMessage) { super.onMessageReceived(remoteMessage) // Check if the message contains data if (remoteMessage.data.isNotEmpty()) { // Handle the data message handleDataMessage(remoteMessage.data) } // Check if the message contains a notification payload remoteMessage.notification?.let { // Handle the notification message handleNotificationMessage(it) } } private fun handleDataMessage(data: Map<String, String>) { // Handle data payload of FCM here } private fun handleNotificationMessage(notification: RemoteMessage.Notification) { // Handle notification payload of FCM here } } ```
3.2. Register the Service:
– In the `AndroidManifest.xml`, register the service:
```xml <service android:name=".MyFirebaseMessagingService"> <intent-filter> <action android:name="com.google.firebase.MESSAGING_EVENT" /> </intent-filter> </service> ```
4. Sending Push Notifications
– Using Firebase Console:
You can send push notifications directly from the Firebase console. Simply go to the “Cloud Messaging” section in the console, compose a new message, select the target (your app), and send!
– Using Backend Service:
If you need more control, you can use a backend service. Send an HTTP POST request to `https://fcm.googleapis.com/fcm/send` with the following headers:
– Content-Type: application/json
– Authorization: key=YOUR_SERVER_KEY
The body of the POST request will contain the payload of the notification.
Conclusion
Firebase Cloud Messaging is an efficient and scalable solution for sending push notifications to your users. Kotlin’s concise and clear syntax coupled with the power of Firebase makes it straightforward to implement push notifications in your Android apps. As users increasingly rely on real-time information, leveraging FCM in your Kotlin apps will significantly enhance the user experience.
Remember, push notifications are a powerful tool but use them judiciously. Overusing notifications can frustrate users and lead to app uninstalls. Aim to strike a balance to keep your users engaged without overwhelming them.
Table of Contents
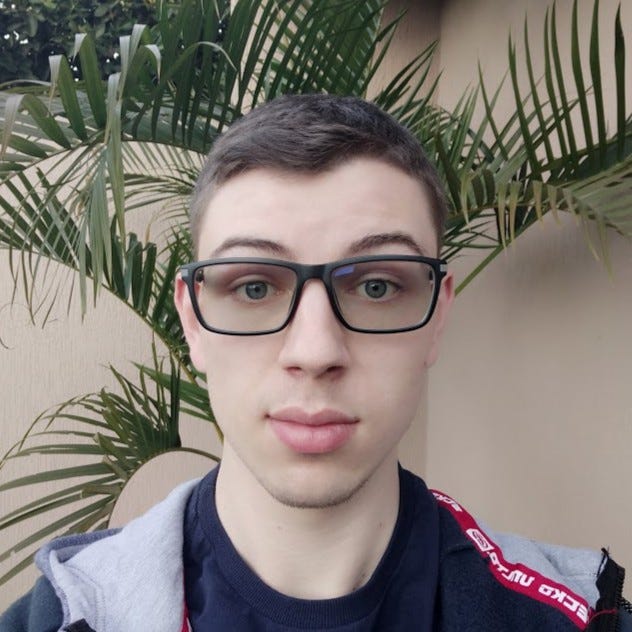
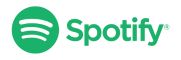