How do you perform function currying in Kotlin?
In Kotlin, you can perform function currying using higher-order functions and lambda expressions. One common approach is to define a currying extension function on a function type, which takes the first argument and returns a lambda that takes the remaining arguments.
Here’s a simple example of function currying in Kotlin:
kotlin fun <A, B, C> ((A, B) -> C).curried(): (A) -> (B) -> C { return { a: A -> { b: B -> this(a, b) } } } fun sum(a: Int, b: Int): Int { return a + b } fun main() { val curriedSum = ::sum.curried() val add5 = curriedSum(5) val result = add5(3) // Result will be 8 println("Result: $result") }
In this example, the curried extension function is defined for a function with two arguments ((A, B) -> C). It returns a curried version of the function, where each argument is applied one at a time, resulting in a chain of functions.
We then define a simple sum function that takes two integers and returns their sum. Using the curried extension function, we curry the sum function and obtain a new function add5 that adds 5 to its argument.
Finally, we invoke add5 with an argument of 3, resulting in the sum of 5 and 3, which is 8.
Function currying is a powerful technique in functional programming that enables partial function application, function composition, and building composable APIs in Kotlin. It promotes code reuse, modularity, and expressiveness, making it a valuable tool for functional programming enthusiasts.
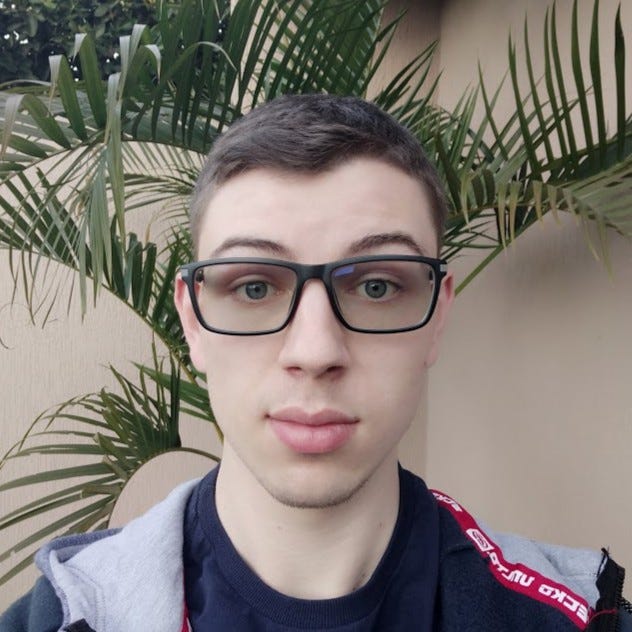
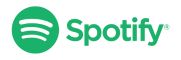