Exploring Functional Programming in Kotlin: Your Guide to Cleaner, More Efficient Code
In the realm of modern software development, choosing the right paradigm plays an essential role in shaping the efficiency and simplicity of your code. Kotlin, as a new-age programming language, has brought a lot to the table in terms of aligning itself with contemporary development needs, including its prowess in functional programming (FP). This has led to a significant rise in the demand to hire Kotlin developers who can leverage these features for efficient software development. This blog post aims to introduce you to functional programming with Kotlin, demonstrating its capacity to simplify your code and boost efficiency with practical examples. If you’re looking to stay on top of modern development trends, hiring skilled Kotlin developers might be the next step for your team.
Understanding Functional Programming
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. In essence, FP promotes higher-level abstractions over instructions. It emphasizes immutability, no side-effects, and first-class functions, enabling developers to write more predictable and maintainable code.
Kotlin Embracing Functional Programming
Kotlin is a statically typed, JVM-based language developed by JetBrains. While itβs an object-oriented programming (OOP) language at heart, Kotlin also incorporates a good number of functional programming features. These features encourage writing more readable, reusable, and concise code that can significantly boost efficiency. Given these benefits, it’s no surprise that businesses are increasingly looking to hire Kotlin developers. These professionals, adept in using Kotlin’s functional programming capabilities, can significantly enhance the quality and efficiency of your software projects.
Let’s dive into some of Kotlin’s functional programming aspects and how they can simplify your code and enhance your coding efficiency.
1. Higher-Order Functions
A higher-order function in Kotlin is a function that can accept functions as parameters and/or return a function. Higher-order functions can significantly simplify your code, making it more concise and readable.
Consider this simple example:
```kotlin fun calculate(operation: (Int, Int) -> Int, a: Int, b: Int): Int { return operation(a, b) } fun main() { val result = calculate({ x, y -> x + y }, 10, 20) println(result) // prints 30 } ```
In this example, `calculate()` is a higher-order function that takes a function `(Int, Int) -> Int` as a parameter along with two integers, and returns an integer.
2. Immutable data
In functional programming, once a variable is assigned, its value should not change, i.e., it should be immutable. Kotlin provides a `val` keyword to declare read-only properties, supporting immutability.
```kotlin fun main() { val message = "Hello, Kotlin!" println(message) // prints "Hello, Kotlin!" } ```
In this example, `message` is a read-only property. Trying to reassign `message` will cause a compile-time error.
3. Pure functions
Pure functions are functions where the return value is only determined by its input values, without observable side effects. This means if you call a function with the same arguments N number of times, you’ll get the same result N times.
```kotlin fun add(a: Int, b: Int): Int { return a + b } ```
`add()` function is a pure function because it will always return the same result if the same arguments are passed.
4. Lambda Expressions
Lambda expressions in Kotlin are anonymous functions; that is, functions without a name. They are used when a function is expected as a parameter in a function.
```kotlin val multiply: (Int, Int) -> Int = { a, b -> a * b } ```
The variable `multiply` is assigned a lambda function that takes two integers and returns their product.
5. Extension Functions
Kotlin allows you to extend a class with new functionality without having to inherit from the class. This is achieved through special declarations called extensions.
```kotlin fun String.removeFirstNChars(n: Int): String { return this.substring(n ) } fun main() { println("Hello, World!".removeFirstNChars(7)) // prints "World!" } ```
In the above example, we’ve extended the `String` class with a new method `removeFirstNChars()`.
6. Collections and Stream API
Kotlin provides a comprehensive set of useful methods for collections like `map`, `filter`, `reduce`, and many more.
```kotlin val numbers = listOf(1, 2, 3, 4, 5, 6) val evenNumbers = numbers.filter { it % 2 == 0 } println(evenNumbers) // prints [2, 4, 6] ```
In this example, we’ve used the `filter` function to get only even numbers from the list.
Boosting Efficiency with Functional Programming in Kotlin
Functional programming in Kotlin not only simplifies the code but also improves efficiency in several ways:
– Easy debugging and testing: Pure functions do not change any states and depend only on input parameters, making the debugging process simpler. Tests are more comfortable to write, more robust, and concise for pure functions.
– Conciseness: Features like higher-order functions, lambda expressions, and collection API reduce boilerplate code and make the code more concise.
– Improved maintainability: By avoiding shared state, mutable data, and side-effects, Kotlin’s functional programming features lead to fewer bugs and more maintainable code.
Conclusion
Kotlin, with its robust functional programming features, has found its way into the toolbox of many developers. Functional programming in Kotlin simplifies code structure and increases efficiency, making it a preferable choice for modern-day programming. This has led to a surge in demand to hire Kotlin developers, who can effectively harness these features to produce optimized code. So if you’re considering expanding your tech team, hiring skilled Kotlin developers could be a strategic move. As always, the key to Kotlin programming lies in understanding and leveraging these features effectively.
Table of Contents
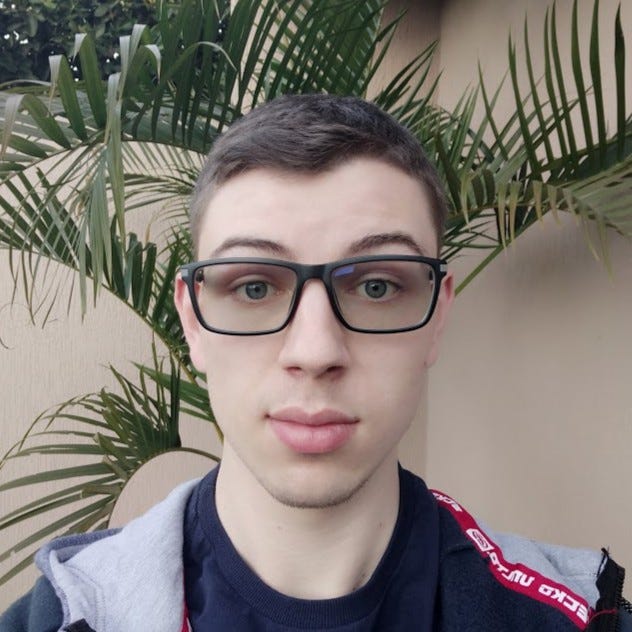
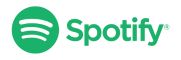