Bridge the Gap: Kotlin’s SAM Conversions and Their Role in Java Compatibility
In the fast-paced world of software development, staying at the forefront of technology is crucial for creating robust and efficient applications. Kotlin, a statically-typed programming language developed by JetBrains, has been making waves in recent years. One of the standout features of Kotlin is its seamless interoperability with Java. In this blog post, we’ll delve into a fascinating aspect of Kotlin known as SAM (Single Abstract Method) conversions and explore how they enhance the interoperability between Kotlin and Java.
Table of Contents
1. Understanding Kotlin’s SAM Conversions
Before we dive into examples, let’s grasp the concept of SAM conversions. In Kotlin, SAM conversions enable you to treat a function or lambda as an instance of a functional interface with a single abstract method. This aligns perfectly with Java’s functional interface concept, making it easier to work with Java libraries and frameworks in your Kotlin codebase.
2. Using Kotlin Lambdas with Java Functional Interfaces
Imagine you’re working on a Kotlin project and need to utilize a Java library that heavily relies on functional interfaces. Kotlin’s SAM conversions make this a breeze. Let’s consider a simple example using the `Runnable` functional interface from Java:
```kotlin val myRunnable: Runnable = Runnable { println("Executing Runnable") } ```
In this code snippet, we define a `Runnable` instance using a Kotlin lambda expression. Kotlin automatically converts the lambda into a `Runnable` object, allowing you to seamlessly integrate it into your Java-based code.
3. Custom Functional Interfaces
SAM conversions are not limited to Java’s built-in functional interfaces. You can create your custom functional interfaces in Kotlin and use them in Java code. Here’s an illustration:
```kotlin interface MathOperation { fun operate(a: Int, b: Int): Int } fun executeOperation(operation: MathOperation, x: Int, y: Int): Int { return operation.operate(x, y) } fun main() { val addition: MathOperation = MathOperation { a, b -> a + b } val result = executeOperation(addition, 10, 5) println("Result of addition: $result") } ```
In this example, we define a custom functional interface `MathOperation` in Kotlin and use it within a Java-compatible `executeOperation` function.
4. Retrofitting Java Libraries in Kotlin
Suppose you’re working on a Kotlin project that requires the use of a Java library like Retrofit for making HTTP requests. Thanks to SAM conversions, Retrofit seamlessly integrates with Kotlin, providing a more idiomatic and concise syntax:
```kotlin // Retrofit interface interface ApiService { @GET("users/{id}") fun getUser(@Path("id") id: Int): Call<User> } fun main() { val retrofit = Retrofit.Builder() .baseUrl("https://api.example.com/") .addConverterFactory(GsonConverterFactory.create()) .build() val apiService = retrofit.create(ApiService::class.java) val call = apiService.getUser(123) call.enqueue(object : Callback<User> { override fun onResponse(call: Call<User>, response: Response<User>) { val user = response.body() println("User: $user") } override fun onFailure(call: Call<User>, t: Throwable) { println("Request failed: ${t.message}") } }) } ```
In this snippet, we define an API service interface using Retrofit. The implementation of the `Callback` interface is concise and readable, thanks to Kotlin’s SAM conversions.
Conclusion
Kotlin’s SAM conversions are a powerful feature that simplifies the interoperability between Kotlin and Java. They allow you to seamlessly work with Java libraries and frameworks, making your code more concise and readable. By leveraging SAM conversions, you can harness the full potential of Kotlin while enjoying compatibility with existing Java codebases.
For more information on Kotlin’s SAM conversions, consider exploring the official Kotlin documentation: Kotlin SAM Conversions – https://kotlinlang.org/docs/reference/java-interop.html#sam-conversions
Table of Contents
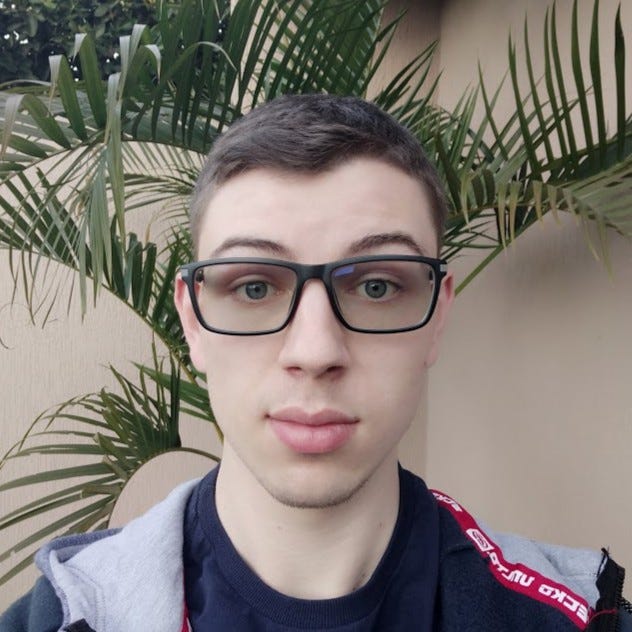
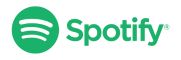