Kotlin and Google Cloud Pub/Sub: Building Message-Oriented Apps
Message-oriented architecture is a cornerstone of modern, scalable applications. Google Cloud Pub/Sub, a fully managed messaging service, enables developers to build systems that can communicate asynchronously, ensuring reliable and scalable communication between services. When combined with Kotlin, a modern, concise, and expressive programming language, developers can create powerful and efficient message-oriented applications. This article explores how Kotlin can be effectively used with Google Cloud Pub/Sub, providing practical examples and best practices.
Understanding Google Cloud Pub/Sub
Google Cloud Pub/Sub is a messaging service that allows services to communicate asynchronously through messages. It follows a publish-subscribe model, where messages are sent (published) to a topic, and one or more subscribers receive them. This model decouples the sender and receiver, leading to more scalable and resilient systems.
Using Kotlin with Google Cloud Pub/Sub
Kotlin, with its concise syntax and full interoperability with Java, is an excellent choice for developing applications that use Google Cloud Pub/Sub. Kotlin’s strong type system, null safety, and coroutines make it well-suited for handling asynchronous communication efficiently.
1. Setting Up Google Cloud Pub/Sub
Before you can start building a message-oriented app, you need to set up Google Cloud Pub/Sub in your Google Cloud project. This involves creating a topic and subscriptions.
Example: Creating a Pub/Sub Topic
```kotlin import com.google.cloud.pubsub.v1.TopicAdminClient import com.google.pubsub.v1.ProjectTopicName fun createTopic(projectId: String, topicId: String) { val topicName = ProjectTopicName.of(projectId, topicId) TopicAdminClient.create().use { topicAdminClient -> topicAdminClient.createTopic(topicName) println("Topic created: $topicName") } } ```
This code snippet demonstrates how to create a topic in Google Cloud Pub/Sub using Kotlin.
2. Publishing Messages
Once the topic is set up, the next step is to publish messages to it. Kotlin’s interoperability with Java allows you to use the Google Cloud Client Library for Pub/Sub seamlessly.
Example: Publishing a Message to a Topic
```kotlin import com.google.cloud.pubsub.v1.Publisher import com.google.protobuf.ByteString import com.google.pubsub.v1.PubsubMessage fun publishMessage(projectId: String, topicId: String, message: String) { val topicName = ProjectTopicName.of(projectId, topicId) val publisher = Publisher.newBuilder(topicName).build() val pubsubMessage = PubsubMessage.newBuilder() .setData(ByteString.copyFromUtf8(message)) .build() publisher.publish(pubsubMessage).get() println("Message published: $message") } ```
In this example, a simple string message is published to a Pub/Sub topic.
3. Subscribing to Messages
Subscribers receive messages from a topic. Kotlin’s coroutines can be used to handle asynchronous message processing efficiently.
Example: Subscribing to a Topic
```kotlin import com.google.cloud.pubsub.v1.Subscriber import com.google.pubsub.v1.ProjectSubscriptionName import kotlinx.coroutines.Dispatchers import kotlinx.coroutines.withContext suspend fun subscribeToTopic(projectId: String, subscriptionId: String) { val subscriptionName = ProjectSubscriptionName.of(projectId, subscriptionId) withContext(Dispatchers.IO) { val receiver = { message: PubsubMessage, consumer: AckReplyConsumer -> println("Received message: ${message.data.toStringUtf8()}") consumer.ack() } val subscriber = Subscriber.newBuilder(subscriptionName, receiver).build() subscriber.startAsync().awaitRunning() } } ```
This example demonstrates how to subscribe to a topic and process incoming messages using Kotlin’s coroutines.
4. Managing Message Flow
Managing the flow of messages is crucial for ensuring that your application handles messages efficiently and without bottlenecks. Kotlin’s coroutine-based approach can help manage message flow by using structured concurrency.
Example: Handling Message Flow
```kotlin import kotlinx.coroutines.flow.collect import kotlinx.coroutines.flow.flow import kotlinx.coroutines.runBlocking fun messageFlow(): Flow<String> = flow { // Emit messages emit("Message 1") emit("Message 2") } fun main() = runBlocking { messageFlow().collect { message -> println("Processing: $message") // Simulate message processing } } ```
This code snippet shows how Kotlin’s Flow API can be used to handle a stream of messages, allowing for efficient message processing.
Conclusion
Kotlin, combined with Google Cloud Pub/Sub, offers a powerful solution for building message-oriented applications. Its modern features, such as coroutines and Flow, enable efficient and scalable message processing. By leveraging these capabilities, developers can build robust and maintainable systems that are ready to handle the demands of modern, distributed architectures.
Further Reading
Table of Contents
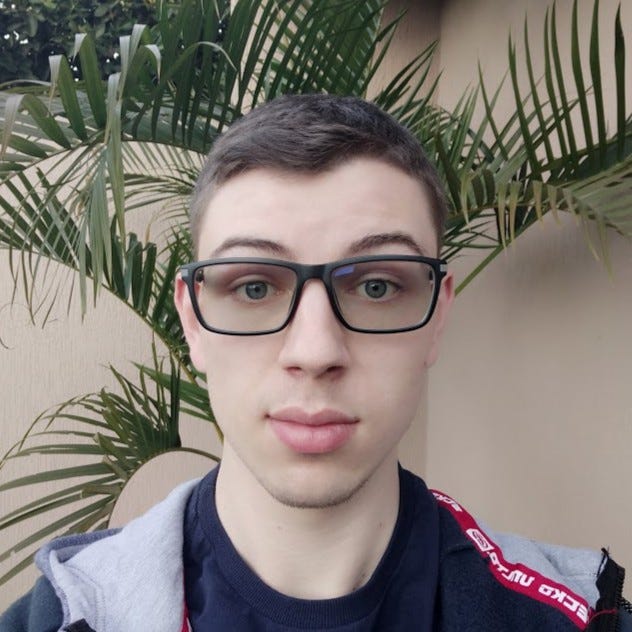
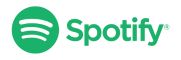