Kotlin Meets Google Maps: The Ultimate Guide to Location Services
Kotlin, the modern and expressive programming language, has rapidly gained popularity in the Android ecosystem. It offers concise syntax, interoperability with Java, and a host of other features that make it a top choice for developers. Among the myriad of functionalities that Kotlin and the Android platform offer, integrating with Google Maps and its location services stands out as an essential feature for numerous applications. Whether it’s ride-sharing, food delivery, or a travel app, a seamless location service is crucial.
In this post, we’ll delve deep into integrating Google Maps and its location services in an Android app using Kotlin.
Prerequisites
- Android Studio installed.
- A valid Google Cloud API key for accessing Maps and Location services.
Step 1: Setting Up the Project
Before diving into coding, we need to set up our project:
- Create a new Android Project in Android Studio.
- In the `build.gradle` (Module: app) file, add the dependencies:
```kotlin implementation 'com.google.android.gms:play-services-maps:17.0.1' implementation 'com.google.android.gms:play-services-location:18.0.0' ```
- Sync the project.
Step 2: Getting the API Key
Go to the Google Cloud Platform Console, create a new project, and enable the Maps SDK and the Location API for Android. Obtain the API key which will be used in your application.
Step 3: Integrating Google Maps
- In your `AndroidManifest.xml`, add the following permissions:
```xml <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" /> <application> ... <meta-data android:name="com.google.android.geo.API_KEY" android:value="YOUR_API_KEY" /> </application> ```
Replace `YOUR_API_KEY` with the API key from the Google Cloud Platform Console.
Now, let’s create a simple layout with a `Fragment` for our map. In `activity_main.xml`:
```xml <fragment xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/map" android:name="com.google.android.gms.maps.SupportMapFragment" android:layout_width="match_parent" android:layout_height="match_parent"/> ```
Step 4: Implementing the Map in Kotlin
In your `MainActivity.kt`, do the following:
```kotlin import com.google.android.gms.maps.CameraUpdateFactory import com.google.android.gms.maps.OnMapReadyCallback import com.google.android.gms.maps.SupportMapFragment import com.google.android.gms.maps.model.LatLng class MainActivity : AppCompatActivity(), OnMapReadyCallback { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) val mapFragment = supportFragmentManager.findFragmentById(R.id.map) as SupportMapFragment mapFragment.getMapAsync(this) } override fun onMapReady(googleMap: GoogleMap?) { googleMap?.apply { val someLocation = LatLng(40.748817, -73.985428) // Example location: New York's Empire State Building this.addMarker(MarkerOptions().position(someLocation).title("Empire State Building")) this.moveCamera(CameraUpdateFactory.newLatLngZoom(someLocation, 10f)) } } } ```
Step 5: Integrating Location Services
To fetch the user’s current location:
- Initialize the location services:
```kotlin private lateinit var fusedLocationClient: FusedLocationProviderClient override fun onCreate(savedInstanceState: Bundle?) { ... fusedLocationClient = LocationServices.getFusedLocationProviderClient(this) } ```
- Get the current location:
```kotlin fusedLocationClient.lastLocation.addOnSuccessListener { location: Location? -> location?.let { val currentLatLng = LatLng(it.latitude, it.longitude) googleMap?.addMarker(MarkerOptions().position(currentLatLng).title("You are here")) googleMap?.moveCamera(CameraUpdateFactory.newLatLngZoom(currentLatLng, 15f)) } } ```
Remember to handle the runtime permission for accessing location.
Examples of Use Cases:
- Travel Apps: Plot interesting landmarks on a map for tourists.
- Ride-Sharing Apps: Track and show the driver’s location in real-time.
- Food Delivery Apps: Display the location of food delivery personnel to the end-users.
Conclusion:
Kotlin’s seamless integration with Google Maps and location services enables developers to embed powerful location-based functionalities in their apps. This guide provides a straightforward introduction to such integrations, opening doors to a host of application ideas and features. Happy coding!
Table of Contents
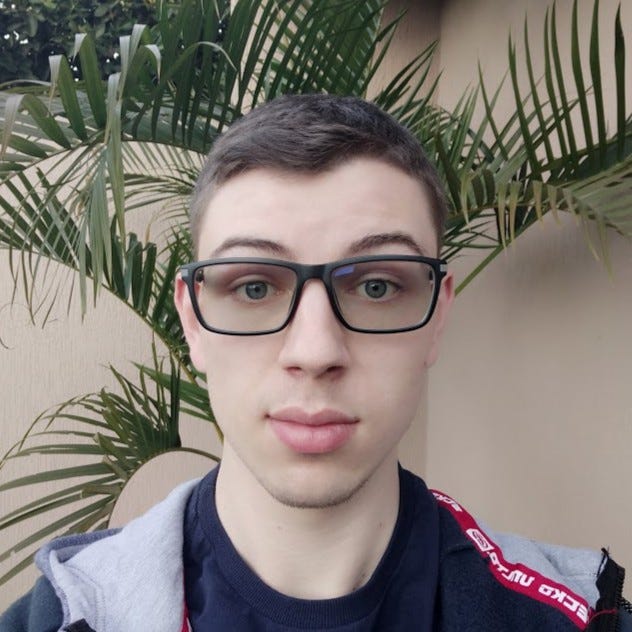
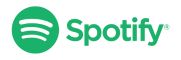