Unlock the Secrets to Fast, Efficient Mobile Apps with Kotlin & GraphQL
In today’s world of mobile application development, it’s important to have a robust back-end solution that can deliver the data needs of a mobile app quickly, efficiently, and securely. Two technologies that have gained significant traction in the mobile app development realm are Kotlin and GraphQL. In this article, we will explore how Kotlin and GraphQL can be combined to build efficient APIs for mobile apps, using practical examples to elucidate our points.
1. The Rise of Kotlin and GraphQL
Kotlin: Kotlin is a statically typed, cross-platform language developed by JetBrains. It is interoperable with Java and has become a go-to choice for Android app development, thanks to its expressive syntax, safety features, and improved performance over Java.
GraphQL: Developed by Facebook in 2012 and open-sourced in 2015, GraphQL is a query language for your API and a runtime for executing those queries. It provides a more flexible, robust, and efficient alternative to the traditional REST API.
2. The Power of Kotlin and GraphQL
Combining Kotlin and GraphQL provides some significant advantages:
- Flexibility: GraphQL allows clients to request only the data they need. This reduces over-fetching or under-fetching of data, thus improving efficiency.
- Strong Typing: Both Kotlin and GraphQL are strongly typed, ensuring better compile-time checks and reducing runtime errors.
- Performance: Less data transfer due to optimized queries means faster loading times and reduced costs.
- Developer Experience: Kotlin’s concise syntax combined with GraphQL’s intuitive querying improves the development experience.
3. Building an API with Kotlin and GraphQL
To demonstrate the process, let’s consider building a mobile app for a library. Users can browse books, authors, and check availability.
Step 1: Setting Up the Environment
For our back-end, we’ll use Ktor (a Kotlin framework) and graphql-java (a GraphQL Java implementation).
```kotlin implementation "io.ktor:ktor-server-core:$ktor_version" implementation "io.ktor:ktor-server-netty:$ktor_version" implementation "com.graphql-java:graphql-java:$graphql_version" ```
Step 2: Defining our Data Models
Define your Kotlin data classes for ‘Book’ and ‘Author’:
```kotlin data class Book(val id: String, val title: String, val authorId: String) data class Author(val id: String, val name: String) ```
Step 3: Setting up GraphQL Schema
Using GraphQL’s Schema Definition Language (SDL):
```graphql type Book { id: ID! title: String! author: Author! } type Author { id: ID! name: String! } type Query { books: [Book]! authors: [Author]! } ```
Step 4: Implementing Resolvers
In GraphQL, resolvers fetch the actual data. Let’s create a simple resolver using Kotlin:
```kotlin class BookResolver : GraphQLResolver<Book> { fun author(book: Book): Author? { // Here, fetch the author for the book from your data source. // For simplicity, we will return a dummy author return Author(book.authorId, "John Doe") } } ```
Step 5: Executing Queries
With the schema and resolvers ready, you can execute GraphQL queries:
Query to get all books:
```graphql { books { title author { name } } } ```
In Kotlin, using graphql-java, the execution would look like:
```kotlin val graphql = GraphQL.newGraphQL(graphQLSchema).build() val response = graphql.execute(query) ```
Conclusion
The combination of Kotlin and GraphQL offers an efficient and developer-friendly approach to building APIs for mobile apps. While we have showcased a simple example, the scalability and power of both technologies can be leveraged to build complex systems with ease.
Developers should strongly consider using Kotlin with GraphQL to optimize the data retrieval process, cut down on redundant data transfers, and offer users a smoother mobile experience. Whether you’re starting a new project or looking to revamp an existing one, Kotlin and GraphQL should be on your radar!
Table of Contents
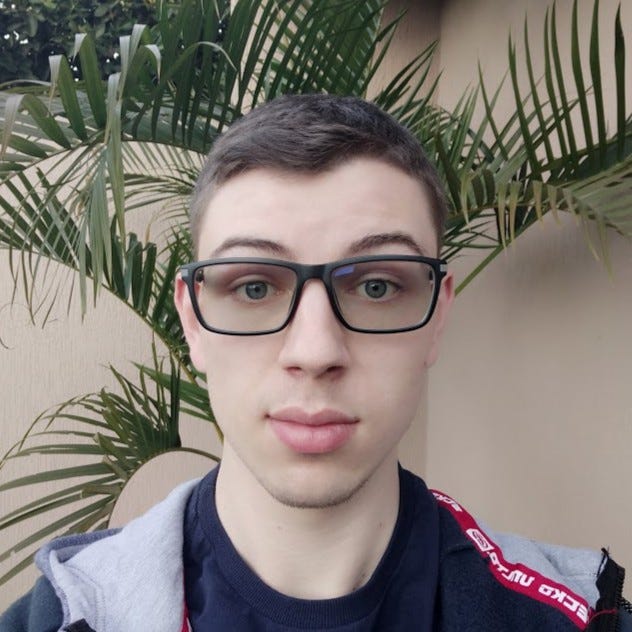
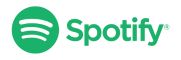