Kotlin and the Art of Higher-Order Functions: Code Examples Inside
Kotlin has garnered immense popularity in the world of software development. One of its selling points is its support for functional programming (FP), which aids in writing concise, readable, and maintainable code. At the core of FP in Kotlin are higher-order functions. Let’s dive deep into understanding these in Kotlin and see how they reshape our programming style.
Table of Contents
1. What are Higher-Order Functions?
In simple terms, a higher-order function is a function that does at least one of the following:
- Takes one or more functions as arguments.
- Returns a function as its result.
In essence, when you hear “higher-order function”, think “a function that works with other functions”.
2. Why use Higher-Order Functions?
Higher-order functions bring a lot to the table:
- Modularity and Reusability: You can create reusable pieces of code by passing around behavior in the form of functions.
- Abstraction: You can abstract away patterns and behaviors, leading to concise and expressive code.
- Flexibility: Higher-order functions offer more malleable and versatile code structures.
3. Kotlin Examples
- Using `filter` on a List
Suppose you have a list of numbers and you want to find all even numbers. You can use the `filter` function:
```kotlin val numbers = listOf(1, 2, 3, 4, 5, 6) val evens = numbers.filter { it % 2 == 0 } println(evens) // Prints [2, 4, 6] ```
In the above example, `filter` is a higher-order function that takes a lambda (`{ it % 2 == 0 }`) as its argument.
- Using `map` to Transform a List
Let’s say you want to square each number in a list:
```kotlin val squared = numbers.map { it * it } println(squared) // Prints [1, 4, 9, 16, 25, 36] ```
Here, `map` is the higher-order function, and it uses the provided lambda to transform each item.
- Custom Higher-Order Function
You can also create your own higher-order functions. Let’s create one that performs an operation on two numbers:
```kotlin fun operateOnNumbers(a: Int, b: Int, operation: (Int, Int) -> Int): Int { return operation(a, b) } val sum = operateOnNumbers(3, 4) { x, y -> x + y } println(sum) // Prints 7 ```
4. Returning Functions
Kotlin allows for returning functions from functions:
```kotlin fun multiplier(factor: Int): (Int) -> Int { return { number -> number * factor } } val triple = multiplier(3) println(triple(5)) // Prints 15 ```
`multiplier` is a higher-order function that returns another function, which is used to multiply a number by a factor.
5. Function Composition
Compose two functions to get a new function:
```kotlin fun <A, B, C> compose(f: (B) -> C, g: (A) -> B): (A) -> C { return { x -> f(g(x)) } } val square: (Int) -> Int = { it * it } val double: (Int) -> Int = { it * 2 } val squareThenDouble = compose(double, square) println(squareThenDouble(3)) // Squares 3 (9) and then doubles it (18). Prints 18. ```
Conclusion
Higher-order functions, while being a fundamental concept in functional programming, offer a way to simplify and modularize our code in Kotlin. They enable us to pass behavior around, leading to more readable and maintainable code structures.
As with every programming paradigm, it’s essential to understand the tool and its purpose rather than applying it blindly. Knowing when and how to use higher-order functions will elevate your Kotlin programming experience and help you write idiomatic Kotlin code.
Explore, practice, and embrace the functional aspect of Kotlin with higher-order functions and witness the clarity it brings to your code!
To hire pre- vetted Kotlin developers from CloudDevs, get in touch with our consultants today.
Table of Contents
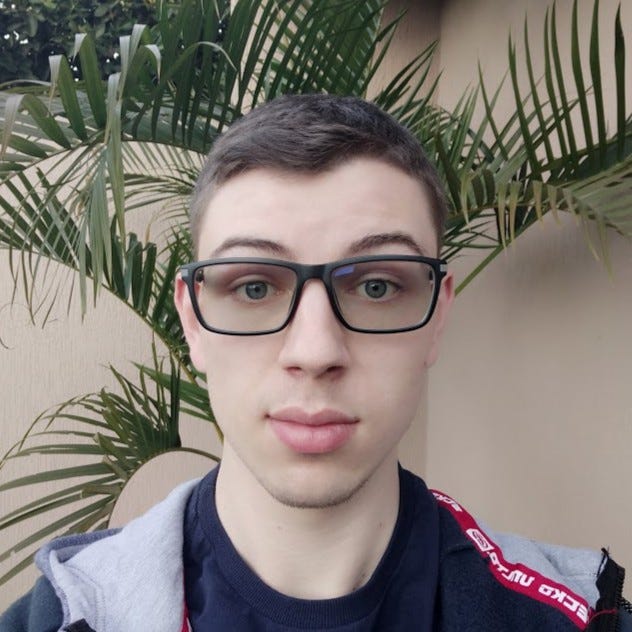
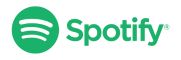