How do you use higher-order functions in Kotlin?
In Kotlin, higher-order functions are functions that can take other functions as parameters or return functions. They empower developers to write concise, expressive, and reusable code by abstracting common patterns and behaviors.
One of the primary ways to use higher-order functions in Kotlin is through function parameters. You can pass functions as arguments to other functions, enabling dynamic behavior based on the passed functions. For instance, consider a higher-order function filter:
kotlin fun filter(numbers: List<Int>, predicate: (Int) -> Boolean): List<Int> { return numbers.filter(predicate) }
Here, the filter function takes a list of integers and a predicate function as parameters. The predicate function determines whether an element should be included in the filtered list.
You can then call the filter function with a lambda expression as the predicate:
kotlin val numbers = listOf(1, 2, 3, 4, 5) val evenNumbers = filter(numbers) { it % 2 == 0 }
In this example, the lambda expression { it % 2 == 0 } acts as the predicate to filter even numbers from the list.
Another way to use higher-order functions is by returning functions from other functions. This technique allows for the creation of factory functions or functions that generate other functions based on certain conditions or parameters.
For instance, consider a function that creates a calculator based on the operation:
kotlin fun operationFactory(operation: String): (Int, Int) -> Int { return when (operation) { "add" -> { a, b -> a + b } "subtract" -> { a, b -> a - b } else -> throw IllegalArgumentException("Unsupported operation") } }
You can then use the operationFactory function to obtain specific calculator functions:
kotlin val add = operationFactory("add") val subtract = operationFactory("subtract") val result = add(5, 3) // result is 8
Here, the operationFactory function returns a function that performs the desired operation based on the input string.
Higher-order functions enable functional programming paradigms in Kotlin, promoting code reuse, composability, and declarative programming styles. They facilitate the creation of flexible and modular codebases that can adapt to changing requirements and promote code readability and maintainability.
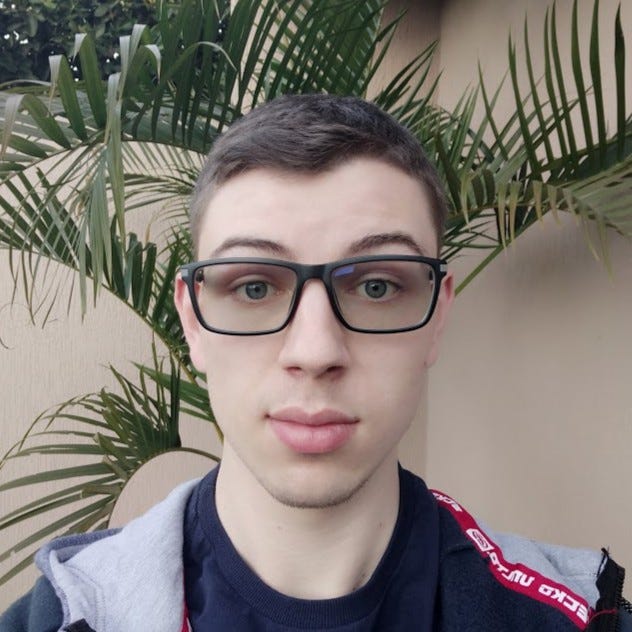
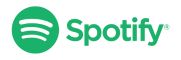