How do you use higher-order functions in Kotlin?
In Kotlin, higher-order functions are a powerful feature that allows functions to be treated as first-class citizens. They enable you to pass functions as arguments, return functions from other functions, and assign functions to variables. This flexibility promotes code reuse, modularity, and the implementation of functional programming paradigms within Kotlin applications.
One common use case for higher-order functions is to abstract behavior that varies among different contexts. For instance, consider a simple higher-order function called filter:
kotlin fun filter(numbers: List<Int>, predicate: (Int) -> Boolean): List<Int> { return numbers.filter(predicate) }
In this example, filter is a higher-order function that takes a list of integers and a predicate function as parameters. The predicate function determines whether an element should be included in the filtered list.
You can then use the filter function with different predicate functions to achieve various filtering criteria:
kotlin val numbers = listOf(1, 2, 3, 4, 5) val evenNumbers = filter(numbers) { it % 2 == 0 } val greaterThanThree = filter(numbers) { it > 3 }
Here, the lambda expressions { it % 2 == 0 } and { it > 3 } act as predicate functions to filter even numbers and numbers greater than three, respectively.
Another common scenario for using higher-order functions is to define custom control structures. For example, the run and apply functions in Kotlin are higher-order functions that enable concise and expressive object initialization and configuration.
kotlin val person = Person().apply { name = "John" age = 30 }
Here, the apply function is a higher-order function that takes a lambda expression as its receiver and allows you to configure the object’s properties within the lambda body.
Higher-order functions facilitate functional composition, allowing you to combine multiple functions to create new behaviors. This composability promotes code readability and maintainability by breaking down complex operations into smaller, reusable components.
Higher-order functions are a key feature of Kotlin’s expressive and flexible syntax. They empower developers to write concise and declarative code that focuses on the “what” rather than the “how” of program execution, leading to more readable, maintainable, and scalable software solutions.
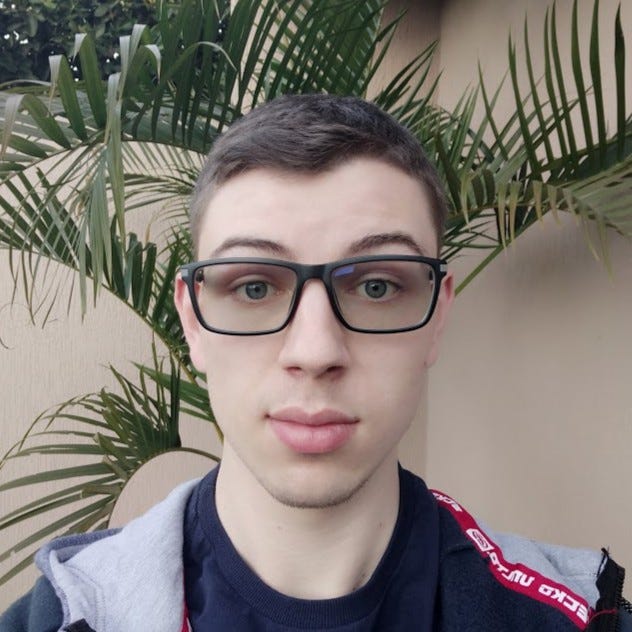
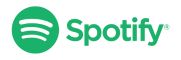