Discover How Kotlin is Revolutionizing the IoT Landscape
The Internet of Things (IoT) is a buzzing arena where physical devices connect to the digital world, often autonomously sharing data, receiving commands, and becoming part of a larger connected ecosystem. One of the challenges with IoT is choosing the right language to program these devices. That’s where Kotlin, the modern and concise programming language developed by JetBrains, enters the frame.
In this blog post, we will delve into the use of Kotlin for IoT, offering a broad perspective on its advantages and presenting hands-on examples.
1. Advantages of Kotlin for IoT:
- Interoperability with Java: Kotlin is fully interoperable with Java, allowing developers to leverage Java’s extensive libraries while enjoying Kotlin’s concise syntax.
- Safety Features: Kotlin has built-in null safety, preventing a lot of common runtime errors that can be disastrous in an IoT context.
- Conciseness: Kotlin’s syntax is expressive, reducing boilerplate code, which is crucial when deploying on devices with limited resources.
- Coroutines: They offer a more efficient way to manage asynchronous tasks without callbacks, making it easier to handle concurrency in IoT devices.
- Community Support: With Kotlin’s growing popularity, there’s an active community which can be beneficial for troubleshooting and finding libraries.
2. Kotlin for IoT: Practical Examples
2.1. A Simple Temperature Logger with Raspberry Pi:
To get started, we’ll create a basic IoT application that logs temperature readings from a sensor connected to a Raspberry Pi.
Prerequisites:
– Raspberry Pi with Kotlin configured.
– DHT22 or similar temperature sensor.
Code:
```kotlin import com.pi4j.io.gpio.* fun readTemperature(): Double { // Dummy function: In reality, integrate with a library to read from DHT22. return 25.0 // Assume 25°C for this example. } fun main() { println("Temperature Logger started") while (true) { val temperature = readTemperature() println("Current Temperature: $temperature°C") Thread.sleep(30000) // Wait for 30 seconds before next reading. } } ```
2.2. Controlling a LED using MQTT and Kotlin:
For this, we’ll use an MQTT broker to receive commands to control an LED.
Prerequisites:
– Raspberry Pi with Kotlin and Paho MQTT client library configured.
– LED connected to a GPIO pin.
Code:
```kotlin import org.eclipse.paho.client.mqttv3.MqttClient import org.eclipse.paho.client.mqttv3.MqttMessage import com.pi4j.io.gpio.* val pin = GpioFactory.getInstance().provisionDigitalOutputPin(RaspiPin.GPIO_01, "LED", PinState.LOW) fun handleMqttMessage(message: MqttMessage) { val payload = String(message.payload) when (payload) { "ON" -> pin.high() "OFF" -> pin.low() } } fun main() { val mqttClient = MqttClient("tcp://BROKER_ADDRESS", MqttClient.generateClientId()) mqttClient.setCallback(object : MqttCallback { override fun messageArrived(topic: String?, message: MqttMessage?) { if (message != null) handleMqttMessage(message) } }) mqttClient.connect() mqttClient.subscribe("home/led") println("Listening for MQTT messages...") } ```
3. Using Coroutines for Asynchronous Data Collection:
Here, we’ll asynchronously collect data from two sensors using Kotlin’s coroutines.
Prerequisites:
– Raspberry Pi with Kotlin and kotlinx.coroutines library.
Code:
```kotlin import kotlinx.coroutines.* fun readTemperatureSensor(): Double { // Dummy function for reading temperature. return 24.0 } fun readHumiditySensor(): Double { // Dummy function for reading humidity. return 60.0 } fun main() = runBlocking { val temperatureJob = async { readTemperatureSensor() } val humidityJob = async { readHumiditySensor() } val temperature = temperatureJob.await() val humidity = humidityJob.await() println("Temperature: $temperature°C, Humidity: $humidity%") } ```
Conclusion
The examples above are simple yet demonstrative of Kotlin’s power and simplicity when applied to IoT contexts. As devices and applications become more complex, having a language that is robust, safe, and developer-friendly becomes critical.
Kotlin, with its modern features and wide range of libraries, is aptly poised for the next wave of IoT innovations. Developers venturing into the IoT landscape can look to Kotlin as a promising tool in their arsenal.
Table of Contents
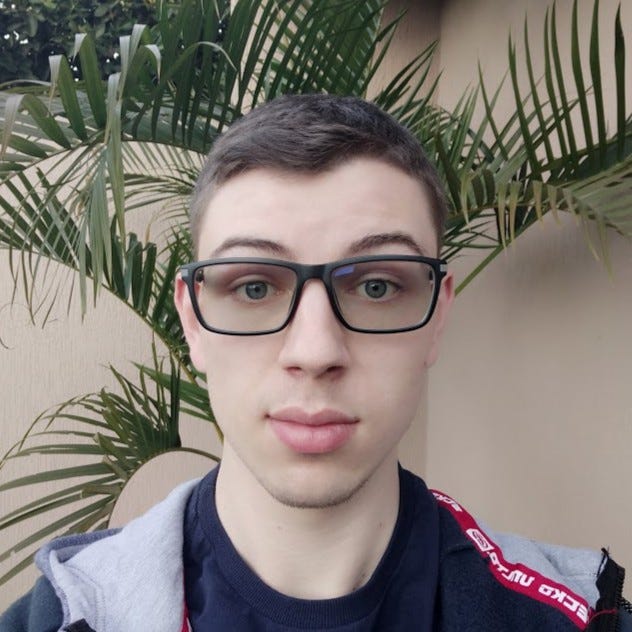
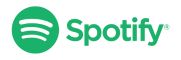