How do you iterate over collections in Kotlin?
In Kotlin, iterating over collections such as lists, sets, maps, and arrays is straightforward and can be done using various methods, including loops and functional-style operations.
Using for loop: You can iterate over a collection using a for loop, which iterates over each element sequentially. For example:
kotlin val numbers = listOf(1, 2, 3, 4, 5) for (number in numbers) { println(number) }
Using forEach() function: Kotlin provides the forEach() function, which iterates over each element of the collection and performs the specified action. For example:
kotlin val names = listOf("John", "Alice", "Bob") names.forEach { println(it) }
Using forEachIndexed() function: If you need to access both the index and the element while iterating, you can use the forEachIndexed() function. For example:
kotlin val numbers = listOf(1, 2, 3, 4, 5) numbers.forEachIndexed { index, number -> println("Index: $index, Value: $number") }
Using iterators: Kotlin collections provide iterator functions like iterator(), hasNext(), and next() to iterate over elements explicitly. For example:
kotlin val set = setOf("apple", "banana", "orange") val iterator = set.iterator() while (iterator.hasNext()) { println(iterator.next()) }
These methods offer flexibility and convenience in iterating over collections in Kotlin, allowing developers to choose the most suitable approach based on their specific requirements and coding style preferences.
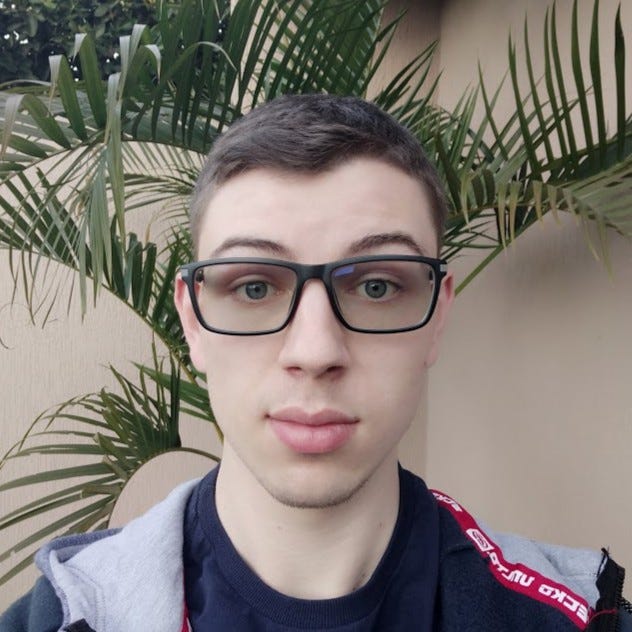
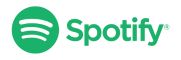