Maximizing Efficiency with Kotlin: A Deep Dive into Key Features and Syntax
Kotlin is a cross-platform, statically typed, general-purpose programming language with type inference. Initially designed by JetBrains, it was released in 2011 to tackle the issues and inflexibility programmers faced with Java. Today, many organizations are seeking to hire Kotlin developers due to the language’s concise, expressive nature and its design that aims to enhance developers’ productivity. As a result, the demand for Kotlin developers is on the rise. This post aims to shed light on the key features of Kotlin and explain its syntax through practical examples. If you’re looking to hire Kotlin developers, this post could also serve as a useful resource for understanding the skills and knowledge these developers should possess.
Null Safety
Kotlin’s primary design goal was to eliminate the Null Pointer Exception (NPE), famously termed “The Billion Dollar Mistake.” The language distinguishes nullable types from non-nullable types, thereby eradicating the likelihood of an NPE during compile time.
For instance, consider the following Java code:
```java String nullableString = null; System.out.println(nullableString.length()); ```
This Java code compiles fine but will throw a NullPointerException at runtime.
Now, compare this with the equivalent Kotlin code:
```kotlin var nullableString: String? = null println(nullableString.length) ```
This code won’t compile. Kotlin enforces null safety at the compile-time, preventing potential bugs from entering your runtime environment.
Coroutines
Coroutines are one of Kotlin’s most powerful features, making it simpler to write asynchronous and non-blocking code. They allow developers to avoid callback hell, a common issue with asynchronous programming, and write cleaner, more readable code.
Consider the following example where we use a coroutine to print a sequence of numbers with a delay:
```kotlin import kotlinx.coroutines.* fun main() = runBlocking { launch { for (i in 1..5) { delay(1000L) println(i) } } } ```
In the example above, `launch` starts a new coroutine. `delay` is a special suspend function that doesn’t block the thread but suspends the coroutine.
Extension Functions
Kotlin also introduces the concept of extension functions, allowing developers to extend a class with new functionality. This addition does not modify the class but makes the code cleaner and easier to read.
For instance, let’s add a function to the String class that reverses the string:
```kotlin fun String.reverse(): String { return this.reversed() } fun main() { val myString = "Kotlin" println(myString.reverse()) } ```
Here, `reverse` is an extension function for the String class. We can call it just like any other function of the String class.
Interoperability with Java
Kotlin is fully interoperable with Java, which means that developers can leverage all existing Java libraries, frameworks, and tools while enjoying the advantages of Kotlin. You can call Kotlin from Java and vice-versa without any overhead.
Consider the following Java class:
```java public class JavaClass { public static String getMessage() { return "Hello from Java!"; } } ```
You can call this Java code directly from Kotlin:
```kotlin fun main() { println(JavaClass.getMessage()) } ```
As seen above, Kotlin can directly interact with Java code, making it a great choice for migrating existing Java codebases or working in projects that use both languages.
Default and Named Arguments
Kotlin allows functions to define default values for arguments, reducing the need for function overloads. It also introduces named arguments, which can make function calls more readable.
Consider this function:
```kotlin fun greet(name: String, title: String = "Friend") { println("Hello, $title $name") } fun main() { greet("John") // uses the default title greet("Jane", "Ms.") // provides a specific title } ```
In this example, the `greet` function has a default value for the `title` argument. When we call `greet(“John”)`, it uses the default title. But we can provide a specific title by calling `greet(“Jane”, “Ms.”)`.
Higher-Order Functions and Lambdas
Kotlin treats functions as first-class citizens, meaning you can store functions in variables and data structures, pass them as arguments to other functions, and return them as results from other functions. This capability is a significant benefit when working with APIs that require or return functions.
Such a feature enables Kotlin developers to write clean, maintainable, and efficient code, and is among the key reasons organizations look to hire Kotlin developers. These developers, equipped with a deep understanding of Kotlin’s unique capabilities, can deftly navigate the demands of complex API-based development tasks, thereby boosting your project’s efficiency and scalability.
Consider the following example of a higher-order function:
```kotlin fun calculate(x: Int, y: Int, operation: (Int, Int) -> Int): Int { return operation(x, y) } fun main() { val sumResult = calculate(4, 5, ::sum) val multiplyResult = calculate(4, 5) { a, b -> a * b } println("sumResult $sumResult, multiplyResult $multiplyResult") } fun sum(x: Int, y: Int) = x + y ```
In the example above, `calculate` is a higher-order function. It takes two Integers and a function as parameters and applies the function to the integers.
Conclusion
Kotlin’s modern language design, its seamless interoperability with Java, and its ability to target multiple platforms, make it a powerful tool for developers. As a result, the demand to hire Kotlin developers has escalated. The language’s features, such as null safety, extension functions, coroutines, default and named arguments, and first-class functions, not only enhance developer productivity but also make code safer and easier to read. These attributes empower developers, including those you might hire as Kotlin developers, to create robust and efficient applications, thereby unlocking the full potential of Kotlin.
Diving deeper into Kotlin, you’ll find that its syntax and features offer a smooth learning curve and a joyful coding experience. This understanding could be beneficial for those who are in the process of hiring Kotlin developers, providing insights into the knowledge and skills that potential candidates should possess. It’s no wonder that Kotlin has emerged as a strong contender in the realm of programming languages, making the decision to hire Kotlin developers a strategic move for many organizations.
Table of Contents
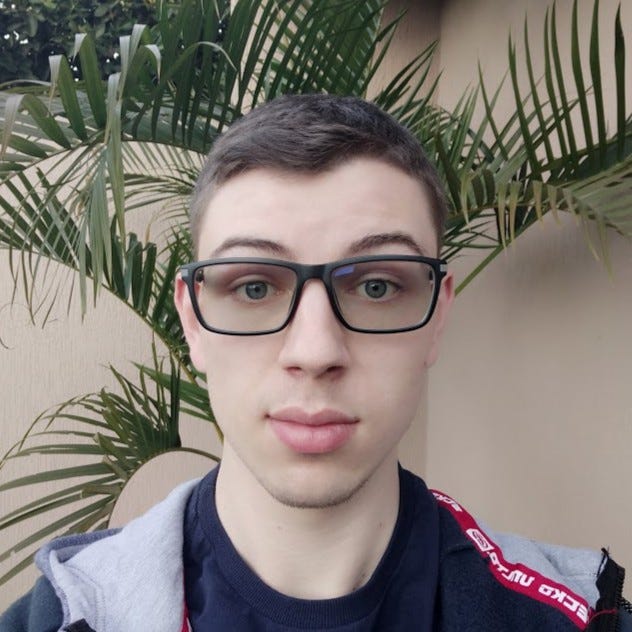
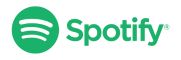