How do you define a lambda in Kotlin?
Defining a lambda expression in Kotlin involves specifying the lambda’s parameters and body within curly braces {}. The syntax for defining a lambda expression is concise and expressive, making it easy to encapsulate behavior and pass it around as a first-class citizen in the Kotlin language.
Here’s the general syntax for defining a lambda expression:
kotlin val lambdaName: (parameters) -> returnType = { parameters -> body }
lambdaName: The name of the lambda expression or the variable holding the lambda.
parameters: The input parameters of the lambda function.
returnType: The return type of the lambda function (if it’s not inferred by the compiler).
body: The code block to be executed when the lambda is invoked.
For example, consider a lambda expression to calculate the sum of two numbers:
kotlin val sum: (Int, Int) -> Int = { a, b -> a + b }
In this example, the lambda expression (Int, Int) -> Int denotes that the lambda takes two integers as input parameters and returns an integer. The body of the lambda { a, b -> a + b } calculates the sum of the two input integers a and b.
Once defined, the lambda expression can be invoked like a regular function, passing arguments as needed:
kotlin val result = sum(5, 3) // result is 8
This code invokes the sum lambda expression with arguments 5 and 3, resulting in the sum 8.
Lambda expressions in Kotlin provide a concise and powerful mechanism for defining behavior and promoting functional programming patterns in Kotlin codebases.
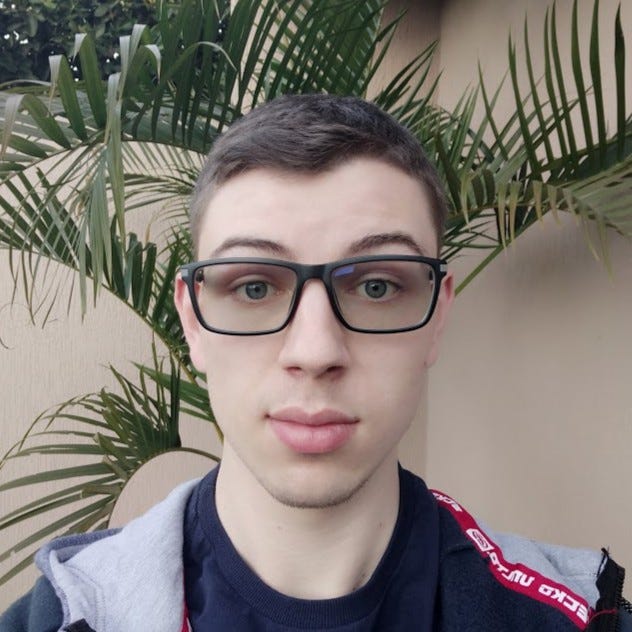
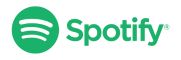