How do you define a lambda with receiver in Kotlin?
To define a lambda with receiver in Kotlin, you follow a specific syntax that includes a receiver type and a lambda expression. Here’s the general syntax for defining a lambda with receiver:
kotlin receiverType.lambdaName(receiverVariable: ReceiverType.() -> Unit)
In this syntax:
- receiverType specifies the type of the receiver object.
- lambdaName is the name of the lambda expression.
- receiverVariable is the name of the receiver object parameter.
- ReceiverType.() -> Unit denotes the lambda expression with receiver, where ReceiverType is the type of the receiver object.
Here’s a simple example of a lambda with receiver:
kotlin val myLambda: String.() -> Unit = { println("Hello, $this!") } fun main() { "Kotlin".myLambda() }
In this example, myLambda is a lambda with receiver that extends the String class. It prints “Hello, Kotlin!” using the receiver object (“Kotlin”) within the lambda body.
Lambda expressions with receivers are particularly useful for defining DSLs (Domain-Specific Languages) and building fluent APIs in Kotlin, where extension functions and lambdas are used to provide a concise and expressive syntax for defining and configuring objects.
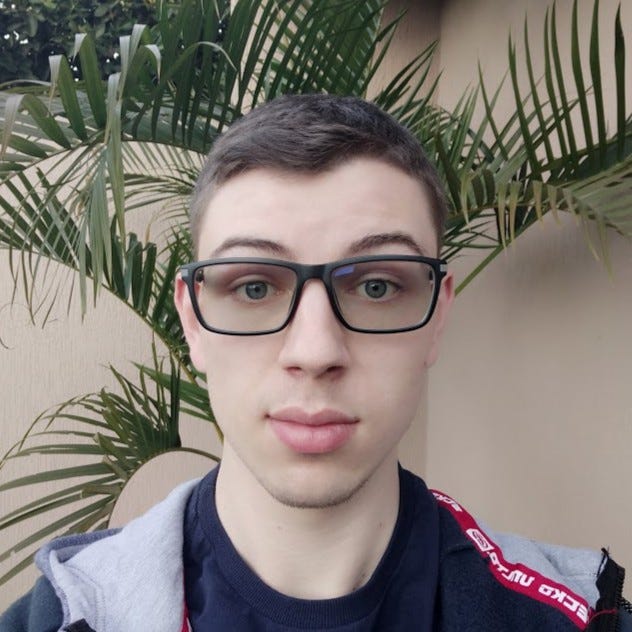
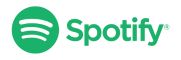